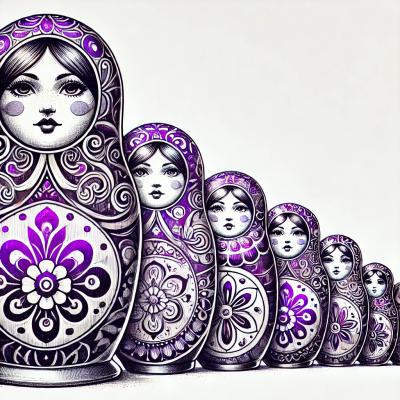
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
d3plus-shape
Advanced tools
Fancy SVG shapes for visualizations
If you use NPM, npm install d3plus-shape
. Otherwise, download the latest release. The released bundle supports AMD, CommonJS, and vanilla environments. Create a custom bundle using Rollup or your preferred bundler. You can also load directly from d3plus.org:
<script src="https://d3plus.org/js/d3plus-shape.v0.8.full.min.js"></script>
Let's say you want to draw 2 rectangles with distinct labels and colors. If you structure your data like this:
var data = [
{text: "Box #1", width: 200, height: 150, x: 100, y: 75, color: "cornflowerblue"},
{text: "Box #2", width: 150, height: 100, x: 285, y: 100, color: "firebrick"}
];
It can be passed to the rectangle generator like this:
new d3plus.Rect()
.data(data)
.fill(function(d) { return d.color; })
.label(function(d) { return d.text; })
.render();
It even detects that the blue rectangle should have a dark label and the red rectangle's should be light!
Please note that the x and y positions are relative to the center of the rectangles.
Click here to view this example live on the web.
Shape
Kind: global class
Extends: Shape
Creates SVG circles based on an array of data.
If value is specified, sets the radius accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current radius accessor.
Kind: static method of Circle
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.r;
}
Updates the style and positioning of the elements matching selector and returns the current class instance. This is helpful when not wanting to loop through all shapes just to change the style of a few.
Kind: static method of Circle
Param | Type |
---|---|
selector | String | HTMLElement |
If value is specified, sets the x accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current x accessor. The number returned should correspond to the horizontal center of the rectangle.
Kind: static method of Circle
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.x;
}
If value is specified, sets the y accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current y accessor. The number returned should correspond to the vertical center of the rectangle.
Kind: static method of Circle
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.y;
}
Kind: global class
Creates SVG images based on an array of data.
Example (a sample row of data)
var data = {"url": "file.png", "width": "100", "height": "50"};
Example (passed to the generator)
new Image().data([data]).render();
Example (creates the following)
<image class="d3plus-shape-image" opacity="1" href="file.png" width="100" height="50" x="0" y="0"></image>
Example (this is shorthand for the following)
image().data([data])();
Example (which also allows a post-draw callback function)
image().data([data])(function() { alert("draw complete!"); })
Renders the current Image to the page. If a callback is specified, it will be called once the images are done drawing.
Kind: static method of Image
Param | Type |
---|---|
[callback] | function |
If data is specified, sets the data array to the specified array and returns the current class instance. If data is not specified, returns the current data array. An tag will be drawn for each object in the array.
Kind: static method of Image
Param | Type | Default |
---|---|---|
[data] | Array | [] |
If ms is specified, sets the animation duration to the specified number and returns the current class instance. If ms is not specified, returns the current animation duration.
Kind: static method of Image
Param | Type | Default |
---|---|---|
[ms] | Number | 600 |
If value is specified, sets the height accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current height accessor.
Kind: static method of Image
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.height;
}
If value is specified, sets the id accessor to the specified function and returns the current class instance. If value is not specified, returns the current id accessor. This is useful if you want to duplicate the same image.
Kind: static method of Image
Param | Type |
---|---|
[value] | function |
Example
function(d) {
return d.url;
}
If selector is specified, sets the SVG container element to the specified d3 selector or DOM element and returns the current class instance. If selector is not specified, returns the current SVG container element.
Kind: static method of Image
Param | Type | Default |
---|---|---|
[selector] | String | HTMLElement | d3.select("body").append("svg") |
If value is specified, sets the URL accessor to the specified function and returns the current class instance. If value is not specified, returns the current URL accessor.
Kind: static method of Image
Param | Type |
---|---|
[value] | function |
Example
function(d) {
return d.url;
}
If value is specified, sets the width accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current width accessor.
Kind: static method of Image
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.width;
}
If value is specified, sets the x accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current x accessor.
Kind: static method of Image
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.x || 0;
}
If value is specified, sets the y accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current y accessor.
Kind: static method of Image
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.y || 0;
}
Shape
Kind: global class
Extends: Shape
Creates SVG lines based on an array of data.
If value is specified, sets the line curve to the specified string and returns the current class instance. If value is not specified, returns the current line curve. The number returned should correspond to the horizontal center of the rectangle.
Kind: static method of Line
Param | Type | Default |
---|---|---|
[value] | String | "linear" |
Updates the style and positioning of the elements matching selector and returns the current class instance. This is helpful when not wanting to loop through all shapes just to change the style of a few.
Kind: static method of Line
Param | Type |
---|---|
selector | String | HTMLElement |
If value is specified, sets the x accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current x accessor. The number returned should correspond to the horizontal center of the rectangle.
Kind: static method of Line
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.x;
}
If value is specified, sets the y accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current y accessor. The number returned should correspond to the vertical center of the rectangle.
Kind: static method of Line
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.y;
}
Shape
Kind: global class
Extends: Shape
Creates SVG rectangles based on an array of data. See this example for help getting started using the rectangle generator.
If value is specified, sets the height accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current height accessor.
Kind: static method of Rect
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.height;
}
Updates the style and positioning of the elements matching selector and returns the current class instance. This is helpful when not wanting to loop through all shapes just to change the style of a few.
Kind: static method of Rect
Param | Type |
---|---|
selector | String | HTMLElement |
If value is specified, sets the width accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current width accessor.
Kind: static method of Rect
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.width;
}
If value is specified, sets the x accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current x accessor. The number returned should correspond to the horizontal center of the rectangle.
Kind: static method of Rect
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.x;
}
If value is specified, sets the y accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current y accessor. The number returned should correspond to the vertical center of the rectangle.
Kind: static method of Rect
Param | Type |
---|---|
[value] | function | Number |
Example
function(d) {
return d.y;
}
Kind: global class
An abstracted class for generating shapes.
If value is specified, sets the background-image accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current background-image accessor.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | function | String | false |
If value is specified, sets the methods that correspond to the key/value pairs and returns the current class instance. If value is not specified, returns the current configuration.
Kind: static method of Shape
Param | Type |
---|---|
[value] | Object |
If data is specified, sets the data array to the specified array and returns the current class instance. If data is not specified, returns the current data array. A shape will be drawn for each object in the array.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[data] | Array | [] |
If ms is specified, sets the animation duration to the specified number and returns the current class instance. If ms is not specified, returns the current animation duration.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[ms] | Number | 600 |
If value is specified, sets the fill accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current fill accessor.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | function | String | "black" |
If value is specified, sets the font-color accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current font-color accessor, which by default returns a color that contrasts the fill color. If an array is passed or returned from the function, each value will be used in conjunction with each label.
Kind: static method of Shape
Param | Type |
---|---|
[value] | function | String | Array |
If value is specified, sets the font-family accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current font-family accessor. If an array is passed or returned from the function, each value will be used in conjunction with each label.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | function | String | Array | "Verdana" |
If value is specified, sets the font resizing accessor to the specified function or boolean and returns the current class instance. If value is not specified, returns the current font resizing accessor. When font resizing is enabled, the font-size of the value returned by label will be resized the best fit the shape. If an array is passed or returned from the function, each value will be used in conjunction with each label.
Kind: static method of Shape
Param | Type |
---|---|
[value] | function | Boolean | Array |
If value is specified, sets the font-size accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current font-size accessor. If an array is passed or returned from the function, each value will be used in conjunction with each label.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | function | String | Array | 12 |
If value is specified, sets the id accessor to the specified function and returns the current class instance. If value is not specified, returns the current id accessor.
Kind: static method of Shape
Param | Type |
---|---|
[value] | function |
If value is specified, sets the label accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current text accessor, which is undefined
by default. If an array is passed or returned from the function, each value will be rendered as an individual label.
Kind: static method of Shape
Param | Type |
---|---|
[value] | function | String | Array |
If bounds is specified, sets the label bounds to the specified function and returns the current class instance. If bounds is not specified, returns the current inner bounds accessor.
Kind: static method of Shape
Param | Type | Description |
---|---|---|
[bounds] | function | The given function is passed the data point, index, and internally defined properties of the shape and should return an object containing the following values: width , height , x , y . If an array is returned from the function, each value will be used in conjunction with each label. |
Example
function(d, i, shape) {
return {
"width": shape.width,
"height": shape.height,
"x": -shape.width / 2,
"y": -shape.height / 2
};
}
If value is specified, sets the label padding to the specified number and returns the current class instance. If value is not specified, returns the current label padding. If an array is passed or returned from the function, each value will be used in conjunction with each label.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | function | Number | Array | 10 |
If value is specified, sets the line-height accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current line-height accessor. If an array is passed or returned from the function, each value will be used in conjunction with each label.
Kind: static method of Shape
Param | Type |
---|---|
[value] | function | String | Array |
Adds or removes a listener to each shape for the specified event typenames. If a listener is not specified, returns the currently-assigned listener for the specified event typename. Mirrors the core d3-selection behavior.
Kind: static method of Shape
Param | Type |
---|---|
[typenames] | String | Object |
[listener] | function |
If value is specified, sets the opacity accessor to the specified function or number and returns the current class instance. If value is not specified, returns the current opacity accessor.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | Number | 1 |
Renders the current Shape to the page. If a callback is specified, it will be called once the shapes are done drawing.
Kind: static method of Shape
Param | Type |
---|---|
[callback] | function |
If value is specified, sets the scale accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current scale accessor.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | function | Number | 1 |
If selector is specified, sets the SVG container element to the specified d3 selector or DOM element and returns the current class instance. If selector is not specified, returns the current SVG container element.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[selector] | String | HTMLElement | d3.select("body").append("svg") |
If value is specified, sets the stroke accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current stroke accessor.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | function | String | "black" |
If value is specified, sets the stroke-width accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current stroke-width accessor.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | function | Number | 0 |
If value is specified, sets the text-anchor accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current text-anchor accessor, which is "start"
by default. Accepted values are "start"
, "middle"
, and "end"
. If an array is passed or returned from the function, each value will be used in conjunction with each label.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | function | String | Array | "start" |
If value is specified, sets the vertical alignment accessor to the specified function or string and returns the current class instance. If value is not specified, returns the current vertical alignment accessor, which is "top"
by default. Accepted values are "top"
, "middle"
, and "bottom"
. If an array is passed or returned from the function, each value will be used in conjunction with each label.
Kind: static method of Shape
Param | Type | Default |
---|---|---|
[value] | function | String | Array | "start" |
FAQs
Fancy SVG shapes for visualizations
The npm package d3plus-shape receives a total of 748 weekly downloads. As such, d3plus-shape popularity was classified as not popular.
We found that d3plus-shape demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.