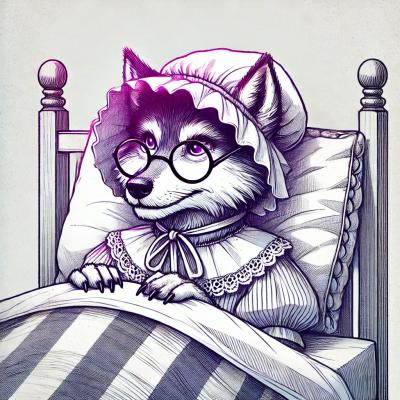
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Micro dom manipulation library. Because jQuery is not needed always.
npm install d_js
and bower bower install d.js
//Get all .buttons elements
var buttons = d.getAll('.buttons');
//Change css properties
d.css(buttons, {
fontFamily: 'Arial',
color: 'red',
transition: 'all 2s'
});
//Handle events
d.on('click', buttons, () => alert('clicked'));
Returns the first element found:
{"selector": elementContext}
or a Node/NodeListvar container = d.get('.container');
//Use an object to specify the context
var buttonInContainer = d.get({'.button': container});
Returns NodeList
with all elements found:
{"selector": elementContext}
d.getAll('.button').forEach(el => el.classList.add('selected'));
Returns an Array
with all siblings of another.
{"selector": elementContext}
or a Node/NodeListd.getSiblings('li'); //return all siblings
d.getSiblings('li', '.filtered'); //return all siblings with class '.filtered'
Attach an event to the elements
Event
{"selector": elementContext}
or a Node/NodeListfunction clickAction(e) {
alert('Event ' + e.type);
}
d.on('click', '.button', clickAction);
Delegate an event to the elements
Event
{"selector": elementContext}
or a Node/NodeListfunction clickAction(e) {
alert('Event ' + e.type);
}
d.on('click', '.navigation', 'a', clickAction);
Removes an event from the elements
Event
{"selector": elementContext}
or a Node/NodeListd.off('click', '.button', clickAction);
Trigger an event of the elements
Event
{"selector": elementContext}
or a Node/NodeListd.trigger('click', '.button');
Set/get data-*
attributes. It can detect and convert primitive types like integers, floats and booleans. If it's an array or object, is converted to json.
//Get a data value
var clicked = d.data('.button', 'clicked');
//Set a new value
d.data('.button', 'clicked', true);
//Set several values
d.data('.button', {
boolean: true,
array: ['blue', 'red', 'green'],
object: {one: '1', two: 2},
integer: 123,
float: 123.45,
});
//Get some values
var someValues = d.data('.button', ['clicked', 'boolean', 'array']);
//Get all values
var allValues = d.data('.button');
Set/get the css properties of the first element. The vendor prefixes are handled automatically.
//Get the value
var color = d.css('.button', 'color');
//Set a new value
d.css('.button', 'color', 'blue');
//Set several values
d.css('.button', {
color: 'red',
backgroundColor: ['blue', 'red', 'green'], //set different values for each element
transform: 'rotate(5deg)' //don't care about vendor prefixes
width: function (el, index) { //use a function to calculate the value for each element
return (100 + (100*index)) + 'px';
}
});
Parses html and returns a DocumentFragment
//parse one element
var button = d.parse('<button>Hello</button>').firstChild;
//parse a list of elements
var buttons = d.parse('<button>Hello</button><button>World</button>');
This library provides also some polyfills to add support for some DOM manipulation convenience methods missing in Explorer and Edge:
Element.prototype.matches
Element.prototype.closest
Element.prototype.remove
Element.prototype.append
Element.prototype.prepend
Element.prototype.before
Element.prototype.after
Element.prototype.replaceWith
NodeList.prototype.forEach
:scope
selectorFAQs
DOM manipulation micro-library
We found that d_js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.