Directed-Acyclic-Graph-Builder-js (dag-builder-js)

What is this?
dag-builder-js is a simple-to-use Javascript DAG library with support to N:N vertices/edges. It supports validating that no cycle can be created in real-time, import/export states and it's built on SVG so you can render graphs pretty much anywhere.
Demo
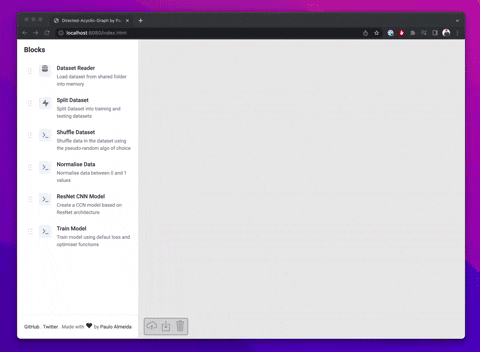
Live demo: https://paulomigalmeida.github.io/directed-acyclic-graph-builder-js/demo/public/index.html
Running the demo in your computer can be achieved by running:
cd <root-dir>
npm install
npm run build
cd ./demo
npm install
npm run start
Installation
Adding dag-builder-js is pretty simple:
Minified version
<script src='https://cdn.jsdelivr.net/npm/dag-builder-js' type="module" charset="utf-8"></script>
Debug version
<script src='https://cdn.jsdelivr.net/npm/dag-builder-js/dist/dag.debug.js' type="module" charset="utf-8"></script>
Or
npm i dag-builder-js --save
Usage
Define a container in which the DAG will be rendered
<div id="graph"></div>
Initialise DAG builder
import {
Graph,
Vertex,
MouseCoordinate,
ShapeSize,
InputVertexConnector,
CustomInputVertexConnector,
OutputVertexConnector,
GraphSerializable,
} from "dag-builder-js";
const graph = new Graph('#graph');
const onVertexAdded = (type, graph, vertex) => {};
graph.addVertexAddedListener(onVertexAdded);
const onVertexRemoved = (type, graph, vertex) => {};
graph.addVertexRemovedListener(onVertexRemoved);
const onEdgeAdded = (type, graph, edge) => {};
graph.addEdgeAddedListener(onEdgeAdded);
const onEdgeRemoved = (type, graph, edge) => {};
graph.addEdgeRemovedListener(onEdgeRemoved);
const onCustomInputEdgeConnectorClicked = (type, graph, vertex, edge, event) => {};
graph.addCustomInputEdgeConnectorClickedListener(onCustomInputEdgeConnectorClicked);
graph.appendVertex(new Vertex(
new MouseCoordinate(100, 100),
new ShapeSize(200, 100),
'Vertex Title',
[
new InputVertexConnector(0, 'data_in', "type1"),
new InputVertexConnector(1, 'other_in', 'type2'),
new CustomInputVertexConnector(2, 'other_in', 'type2', 42),
],
[new OutputVertexConnector(0, 'data_out', 'type3')],
));
graph.update();
Callbacks
onVertexAdded
function onVertexAdded(type, graph, vertex){
}
Gets triggered when vertex is appended to graph.
Parameter | Type | Description |
---|
type | number | event code (see more at: ACTION_TYPE) |
graph | Graph | Graph instance |
vertex | Vertex | Vertex added |
onVertexRemoved
function onVertexRemoved(type, graph, vertex){
}
Gets triggered when vertex is removed from graph.
Parameter | Type | Description |
---|
type | number | event code (see more at: ACTION_TYPE) |
graph | Graph | Graph instance |
vertex | Vertex | Vertex removed |
onEdgeAdded
function onEdgeAdded(type, graph, edge){
}
Gets triggered when edge is appended to graph.
Parameter | Type | Description |
---|
type | number | event code (see more at: ACTION_TYPE) |
graph | Graph | Graph instance |
edge | Edge | Edge added |
onEdgeRemoved
function onEdgeRemoved(type, graph, edge){
}
Gets triggered when edge is removed from graph.
Parameter | Type | Description |
---|
type | number | event code (see more at: ACTION_TYPE) |
graph | Graph | Graph instance |
edge | Edge | Edge removed |
onCustomInputEdgeConnectorClicked
function onCustomInputEdgeConnectorClicked(type, graph, vertex, edge, event){
}
Gets triggered CustomInputEdgeConnector is clicked
Parameter | Type | Description |
---|
type | number | event code (see more at: ACTION_TYPE) |
graph | Graph | Graph instance |
vertex | Vertex | Vertex removed |
edge | Edge | Edge removed |
event | object | DOM event |
Features
Credits/Inspiration/Pieces of code I got from other projects
Flowy
Repository: alyssaxuu/flowy
I got the demo page HTML/CSS and some ideas for documentation and callback listeners. It's a very interesting project and the fact that Alyssa did it all in vanilla javascript is commendable. Kudos to her.
Directed-Graph-Creator
Repository: cjrd/directed-graph-creator
I got a lot of inspiration from the implemenation that @cjrd has written so he also deserves to be listed here. I got the import/export idea from his implementation and learned that I could use d3 for SVG manipulation (which I didn't know at the time).