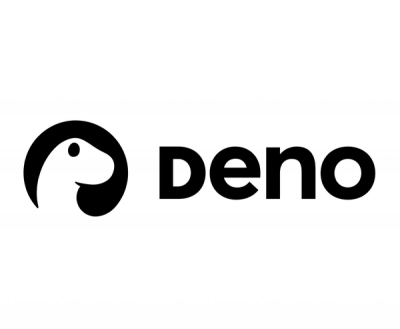
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Danger is a tool that helps automate code review chores. It allows you to create rules that can be enforced during the code review process, ensuring that certain standards and practices are followed.
Automate Code Review
This feature allows you to automate code review tasks by defining rules that can fail or warn if certain conditions are not met. For example, you can fail a pull request if it does not have a description or warn if it does not have any labels.
danger({
fail: 'This PR does not have a description.',
warn: 'This PR does not have any labels.'
});
Check for Changelog Updates
This feature checks if the changelog has been updated in the pull request. If not, it can warn the user to add a changelog entry for their changes.
if (!danger.git.modified_files.includes('CHANGELOG.md')) {
warn('Please add a changelog entry for your changes.');
}
Enforce PR Size Limits
This feature allows you to enforce size limits on pull requests. If a pull request exceeds a certain number of additions and deletions, it can warn the user to consider breaking it down into smaller PRs.
const bigPRThreshold = 500;
if (danger.github.pr.additions + danger.github.pr.deletions > bigPRThreshold) {
warn('This PR is too large. Consider breaking it down into smaller PRs.');
}
ESLint is a tool for identifying and reporting on patterns found in ECMAScript/JavaScript code. While it focuses on linting and enforcing coding standards, it can be used in conjunction with Danger to ensure code quality.
Prettier is an opinionated code formatter that enforces a consistent style by parsing your code and re-printing it. It complements Danger by ensuring code style consistency, which can be part of the automated code review process.
Husky is a tool that allows you to run scripts before committing or pushing code. It can be used to enforce pre-commit hooks, ensuring that certain checks (like those defined in Danger) are run before code is committed.
Danger on Node, wonder what's going on? see VISION.md
This is like, kinda early. If you can take a bit of heat, it's usable in production as of 0.0.4.
Welcome!
So, what's the deal? Well, right now Danger JS does the MVP of the Ruby version. You can look at Git metadata, or GitHub metadata on Travis CI.
Danger can fail your build, write a comment on GitHub, edit it as your build changes and then delete it once you've passed review.
To install:
npm install danger --save-dev
Then add a run command to your Package.json
"danger": "danger"
Then create a dangerfile.js
in the project root with some rules:
import { danger, fail } from "danger"
// Make sure there are changelog entries
const hasChangelog = danger.git.modified_files.includes("changelog.md")
if (!hasChangelog) {
fail("No Changelog changes!")
}
Then you add npm run danger
to the end of your CI run, and Danger will run. 👍
Notes:
Dangerfile.js
needs to be able to run on node without transpiling right now.git
and pr
Awesommmmee.
git clone https://github.com/danger/danger-js.git
cd danger-js
# if you don't have have yarn installed
npm install -g yarn
yarn install
You can then verify your install by running the tests, and the linters:
npm test
npm run lint
npm run flow
We use quite a few semi-bleeding edge features of JS in Danger. Please see the glossary for an overview. Notably Flow, Interfaces, Async/Await and Typealiases.
You'll have a nicer experience as a developer if you use VS Code with Flow enabled, and if you install flow-typed.
npm install -g flow-typed
flow-typed install
( and maybe flow-typed install jest@14
)
Tips:
danger
command globally from your dev build by running npm run link
.debugger
to get a repl and context.Check the issues, I try and keep my short term perspective there. Long term is in the VISION.md.
0.0.5-0.0.10
FAQs
Unit tests for Team Culture
The npm package danger receives a total of 416,330 weekly downloads. As such, danger popularity was classified as popular.
We found that danger demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.