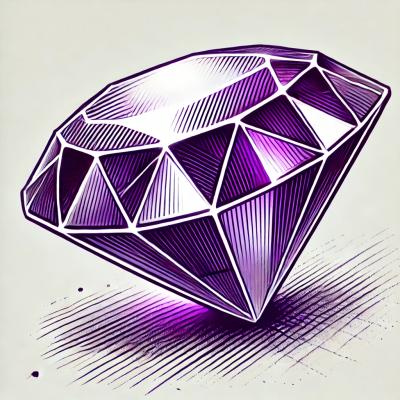
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
dark-sky-api
Advanced tools
A simple and robust wrapper library for Dark Sky API (previously known as Forecast.io).
Features:
See Dark Sky developer docs: https://darksky.net/dev/docs.
Need something even smaller? Dark sky api uses dark-sky-skeleton.
npm install dark-sky-api
import DarkSkyApi from 'dark-sky-api';
Configuring dark-sky-api with an api key is supported but each request will expose said api key (for anyone to capture).
For this reason Dark Sky strongly suggests hiding your API key through use of a proxy [ref].
// one of the two is required
DarkSkyApi.apiKey = 'your-dark-sky-api-key';
DarkSkyApi.proxyUrl = '//base-url-to-proxy/service';
// optional configuration
DarkSkyApi.setUnits = 'si'; // default 'us'
DarkSkyApi.language = 'de'; // default 'en'
DarkSkyApi.postProcessor = (item) => { // default null;
item.day = item.dateTime.format('ddd');
return item;
}
Today's weather:
DarkSkyApi.loadCurrent()
.then(result => console.log(result));
Forecasted week of weather:
DarkSkyApi.loadForecast()
.then(result => console.log(result));
Specific time request:
DarkSkyApi.loadTime('2000-04-06T12:20:05')
.then(result => console.log(result));
By default dark-sky-api will use Geolocation.getCurrentPosition to grab the current browser location automatically.
To manually set geolocation position pass along a position object:
const position = {
latitude: 43.075284,
longitude: -89.384318
};
DarkSkyApi.loadCurrent(position)
.then(result => console.log(result));
Retrieve the current location from the browser
let position;
DarkSkyApi.loadPosition()
.then(pos => {
position = pos;
});
To get the units used in dark sky api responses per configured unit type (default is 'us') use GetResponseUnits
after configuration. Keep in mind that the units would need to be retrieved again if you changed the api units.
const responseUnits = DarkSkyApi.getResponseUnits();
DarkSkyAPi.loadCurrent()
.then((data) => {
console.log(`The temperature is ${data.temperature} degrees ${responseUnits.temperature}`);
console.log(`The wind speed is ${data.windSpeed} ${responseUnits.windSpeed}`);
});
Use extendHourly
to return hour-by-hour data for the next 168 hours, instead of the next 48.
// turn on
DarkSkyApi.extendHourly(true);
DarkSkyApi.loadForecast()
.then(console.log);
// turn off
DarkSkyApi.extendHourly(false);
The post processor method is mapped to all weather items. It's an easy way to add or manipulate responses for an app.
// import
import DarkSkyApi from 'dark-sky-api';
// configure
DarkSkyApi.apiKey = 'my-api-key';
DarkSkyApi.postProcessor = (item) => { // must accept weather data item param
// add a nice date representation using moment.calender
item.dayNice = item.dateTime.calendar(null, {
sameDay: '[Today]',
nextDay: 'ddd',
nextWeek: 'ddd',
lastDay: '[Yesterday]',
lastWeek: '[Last] ddd',
sameElse: 'ddd'
});
// add units object onto item
item.units = DarkSkyApi.getResponseUnits(); // this would be outdated if you changed api units later
return item; // must return weather data item
};
// use
DarkSkyApi.loadCurrent()
.then(data => console.log(data.dayNice)); // Today
To retrieve weather data for a specfic point in time use loadTime
. See docs for more info.
DarkSkyApi.loadTime(time, position);
Time can be a moment or a formatted date string.
const time = moment().year(2000);
DarkSkyApi.loadTime(time)
.then(result => console.log(result));
use setTime
and call without time parameter
DarkSkyApi.setTime('2000-04-06T12:20:05'); // moment().year(2000).format('YYYY-MM-DDTHH:mm:ss')
DarkSkyApi.loadTime()
.then(result => console.log(result));
To retrieve any of these results use loadItAll with optional excludesBlock. ExcludesBlock indicates which data points to omit.
DarkSkyApi.loadItAll(excludes, position);
DarkSkyApi.loadItAll()
.then(console.log);
DarkSkyApi.loadItAll('daily,hourly,minutely,flags') // just return alerts
Tldr: Initialization of the api is automatic, but configure before making api calls.
Static configuration settings such as apiKey, proxyUrl, units, language, and postProcessor are set prior to initialization (configuration phase), locked in during initalization (implicit or explicit), and can be changed after initialization.
Implicit (suggested)
This happens automatically when making a method call such as loadCurrent, loadForecast or loadItAll. Remember to configure beforehand.
// configuration code
DarkSkyApi.apiKey = 'my-api-key';
// api call somewhere else
DarkSkyApi.loadCurrent(); // initialized automatically
Explicit
DarkSkyApi.apiKey = 'my-api-key';
DarkSkyApi.initialize();
or
DarkSkyApi.initialize(apiKey, proxyUrl, units, language, postProcessor); // only apiKey or proxyUrl are required
It's possible to change units, language, postProcessor, extendHourly, and time after initialization. Note: calling any of the static set[Config]
methods will initialize the api so make sure you've added a proxy url or api key before using them.
DarkSkyApi.apiKey = 'my-api-key';
DarkSkyApi.loadCurrent();
// config after initialization
DarkSkyApi.setUnits('auto');
DarkSkyApi.setLanguage('x-pig-latin');
DarkSkyApi.setPostProcessor((item) => {
return {
temperature: item.temperatureMax || item.temperature,
icon: item.icon
};
});
// can only be set after initialization
DarkSkyApi.setTime('2000-04-06T12:20:05');
DarkSkyApi.extendHourly(true);
If you need to maintain multiple instances (configurations) of dark-sky-api create an instance.
// import
import DarkSkyApi from 'dark-sky-api';
// instantiate
const api = new DarkSkyApi(apiKey, proxyUrl, units, language, processor); // only apiKey or proxyUrl are required
// instance config methods support method chaining
api.units('us')
.language('en')
.postProcessor(item => {
item.newProp = val;
return item;
})
.loadCurrent()
.then(console.log);
// extend hourly available for forecasts
api.extendHourly(true)
.loadForecast()
.then(console.log);
// turn off extend hourly
api.extendHourly(false)
.loadForecast()
.then(console.log);
// change position
position = {
latitude: 43.075284,
longitude: -89.384318
};
api.position(position)
.loadCurrent()
.then(console.log);
// change back
api.loadPositionAsync() // get current position
.then(position => api.position(position));
// time machine request
api.time('2000-04-06T12:20:05')
.loadTime()
.then(console.log);
// or
api.loadTime('2000-04-06T12:20:05')
.then(console.log)
FAQs
a simple and robust dark sky api service for client-side js
The npm package dark-sky-api receives a total of 4 weekly downloads. As such, dark-sky-api popularity was classified as not popular.
We found that dark-sky-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.