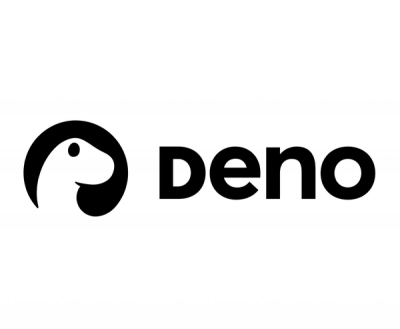
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
dark-sky-skeleton
Advanced tools
Based on Elias Hussary's dark-sky.
An isomorphic barebones js wrapper library for Dark Sky API (previously known as Forecast.io). See Dark Sky developer docs: https://darksky.net/dev/docs.
For a more robust solution see dark-sky-api.
npm install dark-sky-skeleton
import DarkSkySkeleton from 'dark-sky-skeleton';
DarkSkySkeleton(apiKey, proxy)
const api = new DarkSkySkeleton('your-dark-sky-api-key');
The above is simple and great for testing, but it exposes your api key in client side requests. Using a server-side proxy to make the actual api call to dark sky and is highly suggested as this hides the API key from client side requests [ref].
The proxy in this would receive a request issued by dark-sky-api and attach this query to a base uri (like the following: https://api.darksky.net/forecast/your-api-key
) prior to returning a final request.
import DarkSkySkeleton from 'dark-sky-skeleton';
const api = new DarkSkySkeleton(false, '//base-url-to-proxy/service');
const DarkSkySkeleton = require('dark-sky-skeleton');
DarkSkySkeleton(apiKey, proxy)
const api = new DarkSkySkeleton('your-dark-sky-api-key', true);
Passing true as the proxy parameter indicates that the caller is server-side (and essentially a proxy).
darkSky.latitude(lat)
.longitude(long)
.units('us')
.language('en')
.time('2000-04-06T12:20:05') // moment().year(2000).format('YYYY-MM-DDTHH:mm:ss')
.extendHourly(true)
.get();
.then(data => console.log(data));
Feel free to omit setting of latitude and longitude for subsequent calls i.e.:
darkSky.latitude(lat)
.longitude(long)
.get()
.then(data => console.log(data));
darkSky.get().then(data => console.log(data));
"Exclude some number of data blocks from the API response. This is useful for reducing latency and saving cache space (see 'Request Parameters')."
const excludes = ['alerts', 'currently', 'daily', 'flags', 'hourly', 'minutely'],
exludesBlock = excludes.filter(val => val != 'currently').join(',')
darkSky.latitude(lat)
.longitude(long)
.exclude(excludesBlock)
.get()
.then(data => console.log(data));
FAQs
barebones dark sky weather api - for client or server-side js
We found that dark-sky-skeleton demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.