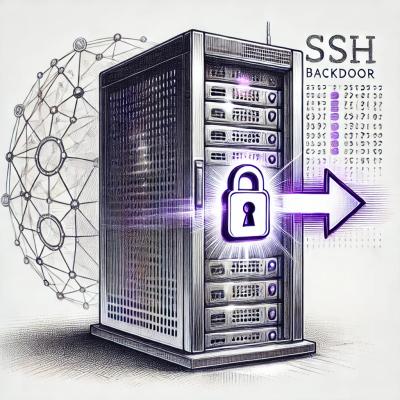
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
datacasting
Advanced tools
Make schemas and cast data declaratively
npm install --save datacasting
# or
yarn add datacasting
import { scheme, toDate } from 'datacasting'
const userScheme = scheme({
verified: Boolean,
created_at: toDate('yyyy-MM-dd'),
})
const casted = userScheme.cast([
{
first_name: 'Jonh',
last_name: 'Doe',
verified: 0,
created_at: '2020-01-23',
}
])
console.log(casted)
// [
// {
// "first_name": "Jonh",
// "last_name": "Doe",
// "verified": false,
// "created_at": Date(2020, 0, 23)
// }
// ]
const rewrited = userScheme.rewrite([
{
first_name: 'Jonh',
last_name: 'Doe',
verified: 0,
created_at: '2020-01-23',
}
])
console.log(rewrited)
// [
// {
// "verified": false,
// "created_at": Date(2020, 0, 23)
// }
// ]
import { scheme, toDate } from 'datacasting'
const userScheme = scheme({
verified: Boolean,
created_at: toDate('yyyy-MM-dd'),
})
const casted = userScheme.cast({
first_name: 'Jonh',
last_name: 'Doe',
verified: 0,
created_at: '2020-01-23',
})
console.log(casted)
// {
// "first_name": "Jonh",
// "last_name": "Doe",
// "verified": false,
// "created_at": Date(2020, 0, 23)
// }
const rewrited = userScheme.rewrite({
first_name: 'Jonh',
last_name: 'Doe',
verified: 0,
created_at: '2020-01-23',
})
console.log(rewrited)
// {
// "verified": false,
// "created_at": Date(2020, 0, 23)
// }
cast
– converts data according to the schema, inheriting the passed fields, even if they were not declared in the schema.rewrite
- converts data according to the schema without inheriting the original fields. The result will be an object only from the fields declared in the schema.First, you can use the built-in type conversion: String
, Number
and Boolean
import { scheme } from 'datacasting'
const dataScheme = scheme({
user_id: String,
verified: Boolean,
role: Number,
})
replace(searchValue: string | RegExp, replaceValue: string | RegExp): (value: string) => string
import { scheme, replace } from 'datacasting'
const dataScheme = scheme({
name: replace('admin', 'nobody'),
})
const casted = dataScheme.cast({
name: "John Doe - admin",
})
console.log(casted)
// {
// "name": "John Doe - nobody"
// }
toArrayOf<K = StringConstructor | NumberConstructor | BooleanConstructor>(constructor: K): (value: any) => K[]
import { scheme, toArrayOf } from 'datacasting'
const dataScheme = scheme({
skill: toArrayOf(String),
})
console.log(dataScheme.cast({
skill: "javascript",
}))
// {
// "skill": ["javascript"]
// }
console.log(dataScheme.cast({
skill: ["javascript", "vue", "react"],
}))
// {
// "skill": ["javascript", "vue", "react"]
// }
toDate(fromFormat: string): (value: string) => Date
Argument fromFormat
refers to date-fns
tokens. See: date-fns parse tokens.
import { scheme, toDate } from 'datacasting'
const dataScheme = scheme({
created_at: toDate('yyyy-MM-dd'),
})
console.log(dataScheme.cast({
created_at: "2020-01-15",
}))
// {
// "created_at": Date(2020, 0, 15)
// }
toInteger(value: any): number
import { scheme, toInteger } from 'datacasting'
const dataScheme = scheme({
balance: toInteger,
})
console.log(dataScheme.cast({
balance: '150.33',
}))
// {
// "balance": 150
// }
toLowerCase(value: any): string
import { scheme, toLowerCase } from 'datacasting'
const dataScheme = scheme({
name: toLowerCase,
})
console.log(dataScheme.cast({
name: 'John Doe',
}))
// {
// "name": "john doe"
// }
toUpperCase(value: any): string
import { scheme, toUpperCase } from 'datacasting'
const dataScheme = scheme({
name: toUpperCase,
})
console.log(dataScheme.cast({
name: 'John Doe',
}))
// {
// "name": "JOHN DOE"
// }
Arguments:
{any} value
{string} key
{Object} data
Returns:
{any} result
import { scheme } from 'datacasting'
const dataScheme = scheme({
role_id: (value, key, data) => {
return data.role.id
})
})
const casted = dataScheme.cast({
name: 'Jonh Doe',
role: {
id: 1,
name: 'user',
}
})
console.log(casted)
// {
// "name": "John Doe",
// "role_id": 1,
// "role": {
// "id": 1,
// "name": "user"
// },
// }
MIT © Daniel Slepov
FAQs
Data converting by schema
The npm package datacasting receives a total of 1 weekly downloads. As such, datacasting popularity was classified as not popular.
We found that datacasting demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.