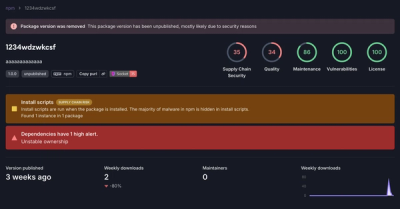
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
The date-fns npm package provides a comprehensive, yet simple and consistent toolset for manipulating JavaScript dates in a browser & Node.js. It offers a variety of functions to parse, validate, manipulate, and format dates.
Parsing Dates
Parse strings in ISO format to JavaScript Date objects.
const parseISO = require('date-fns/parseISO');
const result = parseISO('2023-04-12');
Formatting Dates
Format Date objects into strings with a given format.
const format = require('date-fns/format');
const result = format(new Date(2023, 3, 12), 'yyyy-MM-dd');
Comparing Dates
Compare two dates to determine if one comes before the other.
const isBefore = require('date-fns/isBefore');
const result = isBefore(new Date(2023, 3, 12), new Date(2023, 3, 13));
Manipulating Dates
Perform date calculations such as adding or subtracting time spans.
const addDays = require('date-fns/addDays');
const result = addDays(new Date(2023, 3, 12), 10);
Validating Dates
Check if a date is valid.
const isValid = require('date-fns/isValid');
const result = isValid(new Date('2023-04-12'));
Moment.js is a legacy project, now in maintenance mode, which provides similar functionalities for parsing, validating, manipulating, and formatting dates. It's more object-oriented and mutable compared to the functional and immutable design of date-fns.
Day.js is a lightweight date library that offers a similar API to Moment.js but with a smaller footprint. It is immutable and chainable, like date-fns, but with a different plugin system for extending functionality.
Luxon is a powerful, modern, and chainable library for working with dates and times. It offers a rich set of features for parsing, formatting, manipulating, and querying dates. It's built on the Intl API and provides time zone support out of the box, which is more comprehensive than date-fns's approach to time zones.
Date helpers in the function-per-file style.
npm install date-fns --save
bower install date-fns
var isLastDayOfMonth = require('date-fns/is_last_day_of_month')
var date = new Date(2014, 1, 28)
console.log(isLastDayOfMonth(date))
//=> true
Code is fully documented, check the source for the reference.
format
- format the date.isFuture
- is the given date in the future?isPast
- is the given date in the past?isEqual
- are the given dates equal?isBefore
- is the first date before the second one?isAfter
- is the first date after the second one?compareAsc
- compare the two dates and return -1, 0 or 1.compareDesc
- compare the two dates reverse chronologically and return -1, 0 or 1.max
- return the latest of the given dates.min
- return the earliest of the given dates.closestTo
- return a date from the array closest to the given date.parse
- parse the ISO-8601-formatted date.isValid
- is the given date valid?isValidDateValues
- is the date constructed from the given values exist?distanceInWords
- return the distance between the given dates in words.distanceInWordsToNow
- return the distance between the given date and now in words.isWithinRange
- is the given date within the range?getMilliseconds
- get the milliseconds.setMilliseconds
- set the milliseconds.addMilliseconds
- add the milliseconds to the given date.subMilliseconds
- subtract the milliseconds from the given date.differenceInMilliseconds
- get the number of milliseconds between the given dates.getSeconds
- get the seconds.setSeconds
- set the seconds.startOfSecond
- return the start of a second for the given date.endOfSecond
- return the end of a second for the given date.addSeconds
- add the seconds to the given date.subSeconds
- subtract the seconds from the given date.differenceInSeconds
- get the number of seconds between the given dates.isSameSecond
- are the given dates in the same second?isThisSecond
- is the given date in the same second as the current date?getMinutes
- get the minutes.setMinutes
- set the minutes.startOfMinute
- return the start of a minute for the given date.endOfMinute
- return the end of a minute for the given date.addMinutes
- add the minutes to the given date.subMinutes
- subtract the minutes from the given date.differenceInMinutes
- get the number of minutes between the given dates.isSameMinute
- are the given dates in the same minute?isThisMinute
- is the given date in the same minute as the current date?getHours
- get the hours.setHours
- set the hours.startOfHour
- return the start of an hour for the given date.endOfHour
- return the end of an hour for the given date.addHours
- add hours to the given date.subHours
- subtract hours from the given date.differenceInHours
- get the number of hours between the given dates.isSameHour
- are the given dates in the same hour?isThisHour
- is the given date in the same hour as the current date?getDate
- get the day of the month.setDate
- set the day of the month.getDayOfYear
- get the day of the year.setDayOfYear
- set the day of the year.startOfDay
- return the start of a day for the given date.endOfDay
- return the end of a day for the given date.startOfYesterday
- return the start of yesterday.endOfYesterday
- return the end of yesterday.startOfToday
- return the start of today.endOfToday
- return the end of today.startOfTomorrow
- return the start of tomorrow.endOfTomorrow
- return the end of tomorrow.addDays
- add the specified number of days to the given date.subDays
- subtract the specified number of days from the given date.differenceInDays
- get the number of full days between the given dates.differenceInCalendarDays
- get the number of calendar days between the given dates.isSameDay
- are the given dates in the same day?isYesterday
- is the given date yesterday?isToday
- is the given date today?isTomorrow
- is the given date tomorrow?eachDay
- return the array of dates within the specified range.getDay
- get the day of the week.setDay
- set the day of the week.isWeekend
- is the given date in a weekend?isSunday
- is the given date Sunday?isMonday
- is the given date Monday?isTuesday
- is the given date Tuesday?isWednesday
- is the given date Wednesday?isThursday
- is the given date Thursday?isFriday
- is the given date Friday?isSaturday
- is the given date Saturday?startOfWeek
- return the start of a week for the given date.endOfWeek
- return the end of a week for the given date.lastDayOfWeek
- return the last day of a week for the given date.addWeeks
- add specified number of weeks to the given date.subWeeks
- subtract specified number of weeks from the given date.differenceInWeeks
- get the number of full weeks between the given dates.differenceInCalendarWeeks
- get the number of calendar weeks between the given dates.isSameWeek
- are the given dates in the same week?isThisWeek
- is the given date in the same week as the current date?getISOWeek
- get the ISO week.setISOWeek
- set the ISO week.startOfISOWeek
- return the start of an ISO week for the given date.endOfISOWeek
- return the end of an ISO week for the given date.lastDayOfISOWeek
- return the last day of an ISO week for the given date.differenceInCalendarISOWeeks
- get the number of calendar ISO weeks between the given dates.isSameISOWeek
- are the given dates in the same ISO week?isThisISOWeek
- is the given date in the same ISO week as the current date?getMonth
- get the month.setMonth
- set the month.startOfMonth
- return the start of a month for the given date.endOfMonth
- return the end of a month for the given date.lastDayOfMonth
- return the last day of a month for the given date.addMonths
- add the specified number of months to the given date.subMonths
- subtract the specified number of months from the given date.differenceInMonths
- get the number of full months between the given dates.differenceInCalendarMonths
- get the number of calendar months between the given dates.isSameMonth
- are the given dates in the same month?isThisMonth
- is the given date in the same month as the current date?isFirstDayOfMonth
- is the given date the first day of a month?isLastDayOfMonth
- is the given date the last day of a month?getDaysInMonth
- get the number of days in a month of the given date.getQuarter
- get the year quarter.setQuarter
- set the year quarter.startOfQuarter
- return the start of a year quarter for the given date.endOfQuarter
- return the end of a year quarter for the given date.lastDayOfQuarter
- return the last day of a year quarter for the given date.addQuarters
- add the specified number of year quarters to the given date.subQuarters
- subtract the specified number of year quarters from the given date.differenceInQuarters
- get the number of full quarters between the given dates.differenceInCalendarQuarters
- get the number of calendar quarters between the given dates.isSameQuarter
- are the given dates in the same year quarter?isThisQuarter
- is the given date in the same quarter as the current date?getYear
- get the year.setYear
- set the year.startOfYear
- return the start of a year for the given date.endOfYear
- return the end of a year for the given date.lastDayOfYear
- return the last day of a year for the given date.addYears
- add the specified number of years to the given date.subYears
- subtract the specified number of years from the given date.differenceInYears
- get the number of full years between the given dates.differenceInCalendarYears
- get the number of calendar years between the given dates.isSameYear
- are the given dates in the same year?isThisYear
- is the given date in the same year as the current date?isLeapYear
- is the given date in the leap year?getDaysInYear
- get the number of days in a year of the given date.getISOYear
- get the ISO week-numbering year.setISOYear
- set the ISO week-numbering year.startOfISOYear
- return the start of an ISO week-numbering year for the given date.endOfISOYear
- return the end of an ISO week-numbering year for the given date.lastDayOfISOYear
- return the last day of an ISO week-numbering year for the given date.addISOYears
- add the specified number of ISO week-numbering years to the given date.subISOYears
- subtract the specified number of ISO week-numbering years from the given date.differenceInISOYears
- get the number of full ISO week-numbering years between the given dates.differenceInCalendarISOYears
- get the number of calendar ISO week-numbering years between the given dates.isSameISOYear
- are the given dates in the same ISO week-numbering year?isThisISOYear
- is the given date in the same ISO week-numbering year as the current date?getISOWeeksInYear
- get the number of weeks in the ISO week-numbering year.TODO
Kudos to SauceLabs for the provided Automate API. Thereby we run cross-browser tests on every push!
FAQs
Modern JavaScript date utility library
The npm package date-fns receives a total of 14,696,240 weekly downloads. As such, date-fns popularity was classified as popular.
We found that date-fns demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.