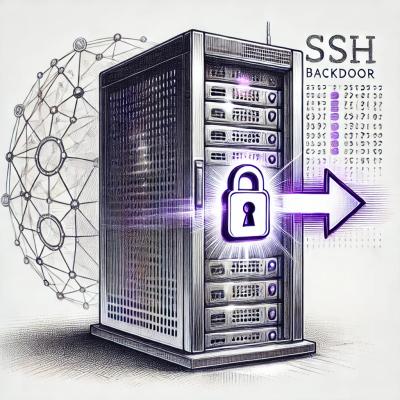
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
dear-image
Advanced tools
A class that represents a graphical image.
npm i dear-image
npm i canvas # to support node
import DearImage from 'dear-image';
let DearImage = require('dear-image');
<script src="https://unpkg.com/dear-image"></script>
The module is globally available as DearImage
.
.isDearImage(value)
Determines whether the passed value is an instance of DearImage
.
argument | description |
---|---|
value | The value to be checked. |
Returns true
if the passed value is an instance of DearImage
, false
otherwise.
.from(value)
Creates a DearImage
instance from the given value.
argument | description |
---|---|
value | The value to create from. Supported value types are ImageData , HTMLCanvasElement , OffscreenCanvas , HTMLImageElement and DearImage . |
Returns the created DearImage
instance.
let element = document.getElementById('my-image');
let image = DearImage.from(element);
document.body.appendChild(image.toHTMLCanvasElement());
.fromExcept(value)
Creates a DearImage
instance from the given value if it's not one.
argument | description |
---|---|
value | The value to create from. |
Returns the same or created DearImage
instance.
let element = document.getElementById('my-image');
let image = DearImage.fromExcept(element);
let otherImage = DearImage.fromExcept(image);
console.log(otherImage === image); // => true
.loadFrom(value)
Asynchronously loads a DearImage
instance from the given value.
argument | description |
---|---|
value | The value to load from. Supported value types are String , URL , Buffer , Blob , HTMLImageElement and everything the function .from supports. |
Returns a promise that resolves to the created DearImage
instance.
let url = '/path/to/image.jpg';
let image = await DearImage.loadFrom(url);
document.body.appendChild(image.toHTMLCanvasElement());
.loadFromExcept(value)
Asynchronously loads a DearImage
instance from the given value if it's not one.
argument | description |
---|---|
value | The value to load from. |
Returns a promise that resolves to the same or created DearImage
instance.
let url = '/path/to/image.jpg';
let image = await DearImage.loadFrom(url);
let otherImage = await DearImage.loadFromExcept(image);
console.log(otherImage === image); // => true
.blank(sizeX = 0, sizeY = 0)
Creates a DearImage
instance without content.
argument | description |
---|---|
sizeXY | The size of the image. |
Returns the created DearImage
instance.
.measureText(text, font = {
family: 'sans-serif',
size: 10,
style: 'normal',
variant: 'normal',
weight: 'normal',
})
Creates a TextMetrics
instance representing the dimensions of the drawn text.
argument | description |
---|---|
text | The text. |
font.family | The font family. |
font.size | The font size. |
font.style | The font style. |
font.variant | The font variant. |
font.weight | The font weight. |
Returns the created TextMetrics
instance.
.text(text, options = {
fill: '#000',
font: {
family: 'sans-serif',
size: 10,
style: 'normal',
variant: 'normal',
weight: 'normal',
},
padding: 0.28,
})
Creates a DearImage
instance with the drawn text.
argument | description |
---|---|
text | The text. |
options.fill | The fill color. |
options.font.family | The font family. |
options.font.size | The font size. |
options.font.style | The font style. |
options.font.variant | The font variant. |
options.font.weight | The font weight. |
options.padding | The space around the text. The value is relative to the font size. |
Returns the created DearImage
instance.
.sizeX
The size of the image along the x-axis.
let image = DearImage.blank(300, 150);
console.log(image.sizeX); // => 300
.sizeY
The size of the image along the y-axis.
let image = DearImage.blank(300, 150);
console.log(image.sizeY); // => 150
.size
The size of the image.
let image = DearImage.blank(300, 150);
console.log(image.size); // => {x: 300, y: 150}
.minSize
The minimum size of the image.
.maxSize
The maximum size of the image.
.resize(sizeX = this.sizeX, sizeY = this.sizeY)
Changes the size of the image.
argument | description |
---|---|
sizeXY | The new size of the image. |
Returns the created DearImage
instance.
let image = DearImage.from(source).resize(300, 150);
.resizeX(size = this.sizeY, proportionally = false)
Changes the size of the image along the x-axis.
argument | description |
---|---|
size | The new size of the image along the x-axis. |
proportionally | If true , the aspect ratio of the image is preserved. |
Returns the created DearImage
instance.
.resizeY(size = this.sizeX, proportionally = false)
Changes the size of the image along the y-axis.
argument | description |
---|---|
size | The new size of the image along the y-axis. |
proportionally | If true , the aspect ratio of the image is preserved. |
Returns the created DearImage
instance.
.crop(originX = 0, originY = 0, sizeX = this.sizeX, sizeY = this.sizeY)
Selects an area from the image.
argument | description |
---|---|
originXY | The origin of the area. A negative number indicates the origin from the end of the image. |
sizeXY | The size of the area. A negative number reverses the direction. |
Returns the created DearImage
instance.
let image = DearImage.from(source).crop(100, -200, -50, 150);
.reframe(sizeX = this.sizeX, sizeY = this.sizeY, alignmentX = 'center', alignmentY = 'center')
Aligns the image inside an area.
argument | description |
---|---|
sizeXY | The size of the area. |
alignmentXY | The alignment of the image. Possible values are 'start' , 'center' and 'end' . |
Returns the created DearImage
instance.
let image = DearImage.from(source).reframe(300, 150, 'start', 'end');
.rescale(scalingX = 1, scalingY = 1)
Changes the size of the image factorially.
argument | description |
---|---|
scalingXY | The scaling factor. |
Returns the created DearImage
instance.
let image = DearImage.from(source).rescale(3/2, 2/3);
.scale(scaling = 1)
Changes the size of the image factorially. The aspect ratio of the image is preserved.
argument | description |
---|---|
scaling | The scaling factor. |
Returns the created DearImage
instance.
let image = DearImage.from(source).scale(3/2);
.scaleIn(sizeX, sizeY)
Scales the image inside an area. The aspect ratio of the image is preserved.
argument | description |
---|---|
sizeXY | The size of the area. |
Returns the created DearImage
instance.
.scaleOut(sizeX, sizeY)
Scales the image outside an area. The aspect ratio of the image is preserved.
argument | description |
---|---|
sizeXY | The size of the area. |
Returns the created DearImage
instance.
.scaleDownIn(sizeX, sizeY)
If necessary, scales the image down inside an area. The aspect ratio of the image is preserved.
argument | description |
---|---|
sizeXY | The size of the area. |
Returns the created DearImage
instance.
.scaleDownOut(sizeX, sizeY)
If necessary, scales the image down outside an area. The aspect ratio of the image is preserved.
argument | description |
---|---|
sizeXY | The size of the area. |
Returns the created DearImage
instance.
.scaleUpIn(sizeX, sizeY)
If necessary, scales the image up inside an area. The aspect ratio of the image is preserved.
argument | description |
---|---|
sizeXY | The size of the area. |
Returns the created DearImage
instance.
.scaleUpOut(sizeX, sizeY)
If necessary, scales the image up outside an area. The aspect ratio of the image is preserved.
argument | description |
---|---|
sizeXY | The size of the area. |
Returns the created DearImage
instance.
.flipX()
Flips the image along the x-axis.
Returns the created DearImage
instance.
.flipY()
Flips the image along the y-axis.
Returns the created DearImage
instance.
.toDataURL(format, quality)
Creates a data URL string representing the content.
Returns the created data URL string.
.toImageData()
Creates an ImageData
object representing the content.
Returns the created ImageData
object.
.toBlob(format, quality)
browser only
Creates a Blob
instance representing the content.
Returns the created Blob
instance.
.toBuffer(format, quality)
node only
Creates a Buffer
instance representing the content.
Returns the created Buffer
instance.
.toHTMLCanvasElement()
browser only
Creates a HTMLCanvasElement
instance representing the content.
Returns the created HTMLCanvasElement
instance.
.toOffscreenCanvas()
browser only
Creates an OffscreenCanvas
instance representing the content.
Returns the created OffscreenCanvas
instance.
.toHTMLImageElement(format, quality)
browser only
Creates a HTMLImageElement
instance representing the content.
Returns the created HTMLImageElement
instance.
let image = DearImage.from(source);
let element = image.toHTMLImageElement('image/jpeg', 0.75);
element.style.border = '1px solid BlueViolet';
document.body.appendChild(element);
.saveToFileSystem(file, format, quality)
node only
Asynchronously saves the content to the file system.
argument | description |
---|---|
file | The file to save to. |
Returns a promise.
let image = DearImage.from(source);
await image.saveToFileSystem('/path/to/image.jpg', 'image/jpeg', 0.75);
FAQs
A class that represents a graphical image.
We found that dear-image demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.