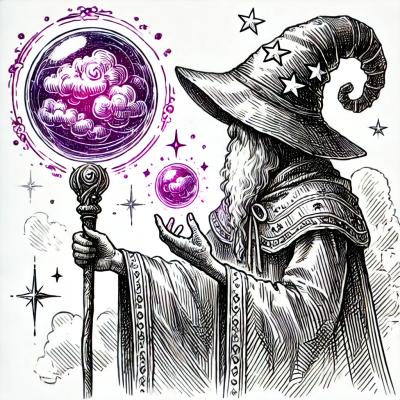
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
decompress
Advanced tools
The decompress npm package is a module that allows for decompressing archive files such as zip, tar, tar.gz, and many others. It provides a simple API to easily extract files from these archives.
Extracting archive files
This feature allows you to extract files from an archive like a zip file into a specified directory. The 'decompress' function returns a promise that resolves with an array of file objects when the extraction is complete.
const decompress = require('decompress');
decompress('unicorn.zip', 'dist').then(files => {
console.log('Files decompressed successfully');
});
Extracting specific files
This feature allows you to extract only specific files from an archive by using a filter function. The filter function is passed each file object, and you return a boolean to indicate whether the file should be extracted.
const decompress = require('decompress');
const filesToExtract = ['unicorn.png', 'rainbow.jpg'];
decompress('archive.zip', 'dist', {
filter: file => filesToExtract.includes(file.path)
}).then(files => {
console.log('Selected files decompressed successfully');
});
Using plugins to handle different archive types
The decompress package can be extended with plugins to support more archive types. In this example, the 'decompress-targz' plugin is used to decompress a '.tar.gz' file.
const decompress = require('decompress');
const decompressTargz = require('decompress-targz');
decompress('unicorn.tar.gz', 'dist', {
plugins: [
decompressTargz()
]
}).then(files => {
console.log('tar.gz file decompressed successfully');
});
adm-zip is a JavaScript implementation for zip data compression for NodeJS. It provides functionalities to read and write zip files, much like decompress, but it is focused solely on zip format.
yauzl is another npm package for reading and extracting zip files. It aims to be a low-level and high-performance library for zip file I/O, and unlike decompress, it does not support multiple file formats.
The 'tar' package provides the ability to create, extract, and list tar archives. It is similar to decompress in handling tar files but does not support other types of archives.
unzipper is a fully streaming unzip library for NodeJS, which provides a simple API for parsing and extracting zip files. It is similar to decompress but focuses on the zip format and offers a streaming interface.
Extracting archives made easy
$ npm install --save decompress
var Decompress = require('decompress');
var decompress = new Decompress({mode: '755'})
.src('foo.zip')
.dest('destFolder')
.use(Decompress.zip({strip: 1}));
decompress.run(function (err) {
if (err) {
throw err;
}
console.log('Archive extracted successfully!');
});
Creates a new Decompress
instance.
Type: String
Set mode on the extracted files, i.e { mode: '755' }
.
Type: Number
Equivalent to --strip-components
for tar.
Type: Array|Buffer|String
Set the files to be extracted.
Type: String
Set the destination to where your file will be extracted to.
Type: Function
Add a plugin
to the middleware stack.
Extract your file with the given settings.
Type: Function
The callback will return an array of vinyl files in files
.
The following plugins are bundled with decompress:
Extract TAR files.
var Decompress = require('decompress');
var decompress = new Decompress()
.use(Decompress.tar({strip: 1}));
Extract TAR.BZ files.
var Decompress = require('decompress');
var decompress = new Decompress()
.use(Decompress.tarbz2({strip: 1}));
Extract TAR.GZ files.
var Decompress = require('decompress');
var decompress = new Decompress()
.use(Decompress.targz({strip: 1}));
Extract ZIP files.
var Decompress = require('decompress');
var decompress = new Decompress()
.use(Decompress.zip({strip: 1}));
$ npm install --global decompress
$ decompress --help
Usage
$ decompress <file> [directory]
$ cat <file> | decompress [directory]
Example
$ decompress --strip 1 file.zip out
$ cat file.zip | decompress out
Options
-m, --mode Set mode on the extracted files
-s, --strip Equivalent to --strip-components for tar
MIT © Kevin Mårtensson
FAQs
Extracting archives made easy
The npm package decompress receives a total of 1,868,511 weekly downloads. As such, decompress popularity was classified as popular.
We found that decompress demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.