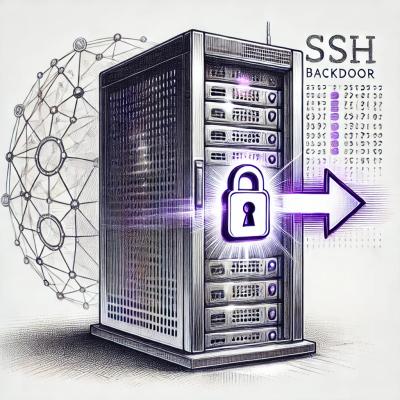
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
deep-props
Advanced tools
Creates an array of deep paths and properties associated with an object. Non-recursively iterates through deep objects until an endpoint is reached. Optionally unpacks prototypes and non-enumerable property descriptors. Supports Objects, Arrays, Maps, and
Creates an array of deep paths and properties associated with an object. Non-recursively iterates through deep objects until an endpoint is reached. Optionally unpacks prototypes and non-enumerable property descriptors. Supports Objects, Arrays, Maps, and Sets automatically.
Endpoints may be previously discovered object references, primitives, or objects whose children are inaccessible due to settings or otherwise.
Avoids recursion by using a task queue; very deep objects may be traversed without hitting the stack limit.
Any unsupported data structure may be accessed by supplying a customizer function. See the global docs.
Circular references or otherwise duplicate references to objects will be signified using a 'ref' property, rather than a value. See the return details.
Node.JS version 8.7.0 or above.
npm install deep-props
The following command will test the package for errors. It prints a large selection of examples to the console; scroll through its output if you want to learn more about the utility.
npm test --prefix /path/to/node_modules/deep-props
const props = require('deep-props')
Nested object extraction
const data = { foo: { bar: { baz: 'qux' } } }
// returns { path: [ 'foo', 'bar', 'baz' ], value: 'qux' }
props(data)
Unrooting of Object Keys
const data = new Map().set(
{ foo: 'bar' }, new Map().set(
{ baz: 'beh' }, new Map().set(
{ qux: 'quz' }, new Map().set(
{ quux: 'quuz' }, 'thud'
)
)
)
)
// returns:
// [
// {
// path: [ { foo: 'bar' }, { baz: 'beh' }, { qux: 'quz' }, { quux: 'quuz' } ],
// value: 'thud'
// },
// { host: { quux: 'quuz' }, path: ['quux'], value: 'quuz' },
// { host: { qux: 'quz' }, path: ['qux'], value: 'quz' },
// { host: { baz: 'beh' }, path: ['baz'], value: 'beh' },
// { host: { foo: 'bar' }, path: ['foo'], value: 'bar' }
// ]
props(data)
Extraction from complicated nests
const data = {
foo: [
new Map().set(
'bar', new Set([
{
baz: {
qux: {
quz: [
'quux',
'quuz'
]
}
}
},
{
lorem: {
ipsum: 'dolor'
}
}
])
)
]
}
// returns:
// [
// {
// path: [ 'foo', '0', 'bar', '0', 'baz', 'qux', 'quz', '0' ],
// value: 'quux' },
// { path: [ 'foo', '0', 'bar', '0', 'baz', 'qux', 'quz', '1' ],
// value: 'quuz' },
// { path: [ 'foo', '0', 'bar', '1', 'lorem', 'ipsum' ],
// value: 'dolor'
// }
// ]
props(data)
Verbose Options
const data = { foo: { bar: 'baz' } }
Object.freeze(data.foo)
// returns:
// [
// {
// path: ['foo'],
// value: { bar: 'baz' },
// writable: true,
// enumerable: true,
// configurable: true,
// parentIsFrozen: false,
// parentIsSealed: false,
// parentIsExtensible: true
// },
// {
// path: [ 'foo', 'bar' ],
// value: 'baz',
// writable: false,
// enumerable: true,
// configurable: false,
// parentIsFrozen: true,
// parentIsSealed: true,
// parentIsExtensible: false
// }
// ]
props(data, { stepwise: true, descriptors: true, permissions: true })
Array.<PropAt>
| Search
Returns: Array.<PropAt>
| Search
- Array of paths and values or references. Returns Search generator if opt.gen is true.
Param | Type | Default | Description |
---|---|---|---|
host | Host | Object to unpack. | |
[opt] | Options | {} | Execution settings. |
Object
See: Options
Execution-wide settings supplied to the module. Modifies types of data attached to results. Modifies types of children to extract.
Properties
Name | Type | Default | Description |
---|---|---|---|
[inherited] | boolean | Whether or not to search for inherited properties. Attaches these keys behind a '__proto__' key. | |
[own] | boolean | true | Whether or not to search for own properties. Defaults to true. |
[nonEnumerable] | boolean | Whether or not to search for and return non-enumerable properties. | |
[permissions] | boolean | Whether or not to attach Permissions to results. | |
[descriptors] | boolean | Whether or not to attach property descriptors other than 'value' to results. | |
[stepwise] | boolean | Whether or not to yield a PropAt object at every step down the chain. | |
[includeRefValues] | boolean | Whether or not to attach a value to Props with Refs attached. | |
[gen] | boolean | Whether or not to return a generator instead of executing the entire search. | |
[full] | boolean | If true, replaces undefined options with maximum search settings (all options except for propsCustomizer will be set to true). User supplied options supercede any changes here. | |
[propsCustomizer] | PropsCustomizer | Function used for custom extraction of PropEntries from a Target. |
Object
See: PropAt
Description of a given level of the chain. Transformed Prop Object with location attched.
Properties
Name | Type | Description |
---|---|---|
[host] | Host | When a non-primitive key has been encountered, a separate chain will be created with that key. Items on that chain will be labeled with a 'host' property to specify which Host the path applies to. PropAt Objects lacking a 'host' property imply that the path applies to the initially supplied Host. |
path | Path | Describes the steps taken from the Host in order to reach the Prop's value. |
[value] | * | Value described at the Prop's location (if any). In cases of a previously discovered reference (circular or otherwise), value will be replaced with a ref property (unless opt.showRefValues is true). |
[writable] | boolean | 'Writable' property descriptor of the value. |
[enumerable] | boolean | 'Enumerable' property descriptor of the value. |
[configurable] | boolean | 'Configurable' property descriptor of the value. |
[parentIsFrozen] | boolean | Frozen status of the parent object. |
[parentIsSealed] | boolean | Sealed status of the parent object. |
[parentIsExtensible] | boolean | Extensible status of the parent object. |
[ref] | Ref | If the value strictly equals a previously discovered Target, the Host and Path of that Target will be provided. |
Versioned using SemVer. For available versions, see the tags on this repository.
This project is licensed under the MIT License - see the LICENSE file for details
0.0.8 (2018-04-28)
| Complete Additions | Release Notes | README | --- | --- | --- |
| Source Code (zip) | Source Code (tar.gz) | | --- | --- |
FAQs
Provides a collection of non-recursive tools for performing operations on deeply nested object properties and prototypes. Allows for custom execution settings including non-native dataset handling.
The npm package deep-props receives a total of 6 weekly downloads. As such, deep-props popularity was classified as not popular.
We found that deep-props demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.