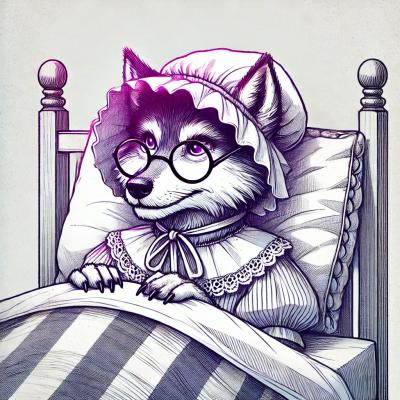
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The 'deepcopy' npm package is used to create deep copies of JavaScript objects. This means that it recursively copies all nested objects and arrays, ensuring that the new object is completely independent of the original.
Deep Copy of Objects
This feature allows you to create a deep copy of an object, ensuring that nested objects are also copied.
const deepcopy = require('deepcopy');
const original = { a: 1, b: { c: 2 } };
const copy = deepcopy(original);
console.log(copy); // { a: 1, b: { c: 2 } }
Deep Copy of Arrays
This feature allows you to create a deep copy of an array, ensuring that nested arrays and objects are also copied.
const deepcopy = require('deepcopy');
const original = [1, [2, 3], { a: 4 }];
const copy = deepcopy(original);
console.log(copy); // [1, [2, 3], { a: 4 }]
Handling Circular References
This feature allows you to handle circular references in objects, ensuring that the deep copy process does not result in an infinite loop.
const deepcopy = require('deepcopy');
const original = { a: 1 };
original.b = original;
const copy = deepcopy(original);
console.log(copy); // { a: 1, b: [Circular] }
Lodash is a popular utility library that provides a wide range of functions for manipulating arrays, objects, and other data types. It includes a `cloneDeep` function that performs deep copying similar to `deepcopy`. However, Lodash offers many additional utilities beyond deep copying.
The 'rfdc' (Really Fast Deep Clone) package is designed for performance and provides a very fast way to deep clone objects. It is similar to `deepcopy` in functionality but is optimized for speed.
The 'clone-deep' package is another utility for deep cloning objects and arrays. It is similar to `deepcopy` but offers additional options for customizing the cloning process.
deep copy module for node.js
$ npm install deepcopy
var deepcopy = require('deepcopy');
var source = {
data: {
num: 123,
str: 'a',
now: new Date,
reg: /node/ig,
arr: [ true, false, null, undefined ],
obj: { aaa: 1, bbb: 2, ccc: 3 }
}
};
var shallow = source,
deep = deepcopy(source);
delete source.data;
console.dir(source);
// {}
console.dir(shallow);
// {}
console.dir(deep);
// { data:
// { num: 123,
// str: 'a',
// now: Sun Jan 27 2013 23:31:12 GMT+0900 (JST),
// reg: /node/gi,
// arr: [ true, false, null, undefined ],
// obj: { aaa: 1, bbb: 2, ccc: 3 } } }
var deepcopy = require('deepcopy');
var a = {},
b = {};
a.to = b;
b.to = a;
try {
deepcopy(a);
} catch (e) {
console.error(e); // [RangeError: Maximum call stack size exceeded]
}
targetObject
any types - copy targetreturn
targetObject types - copied valuereturn deep copy if targetObject
is Date, RegExp or primitive types.
return shallow copy if targetObject
is function.
this function throws RangeError if targetObject has circular reference.
$ npm install
$ npm test
The MIT License. Please see LICENSE file.
0.1.2 / 2013-03-29
FAQs
deep copy data
The npm package deepcopy receives a total of 275,751 weekly downloads. As such, deepcopy popularity was classified as popular.
We found that deepcopy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.