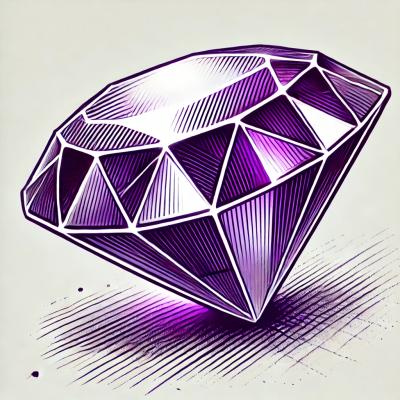
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
The deepmerge npm package is a library for deep (recursive) merging of Javascript objects. It is useful for combining objects with nested structures, such as configuration settings or state objects in applications.
Merging two objects
This feature allows you to merge two objects deeply. Properties from the second object will be added to the first, and if properties are objects themselves, they will be merged recursively.
{"const merge = require('deepmerge');
const x = { foo: { bar: 3 } };
const y = { foo: { baz: 4 } };
const z = merge(x, y);
console.log(z); // { foo: { bar: 3, baz: 4 } }"}
Merging with array concatenation
This feature allows you to specify how arrays are merged. By default, arrays are merged by concatenation, but you can provide a custom arrayMerge function.
{"const merge = require('deepmerge');
const x = { foo: [1, 2, 3] };
const y = { foo: [4, 5, 6] };
const z = merge(x, y, { arrayMerge: (destinationArray, sourceArray) => destinationArray.concat(sourceArray) });
console.log(z); // { foo: [1, 2, 3, 4, 5, 6] }"}
Merging with array replacement
This feature allows you to replace the destination array with the source array instead of merging or concatenating them.
{"const merge = require('deepmerge');
const x = { foo: [1, 2, 3] };
const y = { foo: [4, 5, 6] };
const z = merge(x, y, { arrayMerge: (destinationArray, sourceArray) => sourceArray });
console.log(z); // { foo: [4, 5, 6] }"}
Merging with custom options
This feature allows you to provide custom merge functions to handle the merging process according to your specific requirements.
{"const merge = require('deepmerge');
const x = { foo: { bar: 3 } };
const y = { foo: { bar: 4, baz: 5 } };
const overwriteMerge = (destinationArray, sourceArray, options) => sourceArray;
const z = merge(x, y, { arrayMerge: overwriteMerge });
console.log(z); // { foo: { bar: 4, baz: 5 } }"}
Lodash provides a merge function that can recursively merge own and inherited enumerable string keyed properties of source objects into the destination object. It's similar to deepmerge but is part of the larger lodash utility library.
The extend package is a port of the jQuery.extend method that can deep copy both arrays and objects. It is less specialized than deepmerge and does not provide as many options for customizing the merge behavior.
This package offers functionality similar to Object.assign but with deep merging capabilities. It is a smaller and more focused utility compared to deepmerge, but it may not offer the same level of customization for array merging and other specific use cases.
~540B gzipped, ~1.1kB minified
Merge the enumerable attributes of two objects deeply.
var x = {
foo: { bar: 3 },
array: [{
does: 'work',
too: [ 1, 2, 3 ]
}]
}
var y = {
foo: { baz: 4 },
quux: 5,
array: [{
does: 'work',
too: [ 4, 5, 6 ]
}, {
really: 'yes'
}]
}
var expected = {
foo: {
bar: 3,
baz: 4
},
array: [{
does: 'work',
too: [ 1, 2, 3, 4, 5, 6 ]
}, {
really: 'yes'
}],
quux: 5
}
merge(x, y) // => expected
var merge = require('deepmerge')
Merge two objects x
and y
deeply, returning a new merged object with the
elements from both x
and y
.
If an element at the same key is present for both x
and y
, the value from
y
will appear in the result.
Merging creates a new object, so that neither x
or y
are be modified. However, child objects on x
or y
are copied over - if you want to copy all values, you must pass true
to the clone option.
Merges two or more objects into a single result object.
var x = { foo: { bar: 3 } }
var y = { foo: { baz: 4 } }
var z = { bar: 'yay!' }
var expected = { foo: { bar: 3, baz: 4 }, bar: 'yay!' }
merge.all([x, y, z]) // => expected
The merge will also merge arrays and array values by default. However, there are nigh-infinite valid ways to merge arrays, and you may want to supply your own. You can do this by passing an arrayMerge
function as an option.
function concatMerge(destinationArray, sourceArray, options) {
destinationArray // => [1, 2, 3]
sourceArray // => [3, 2, 1]
options // => { arrayMerge: concatMerge }
return destinationArray.concat(sourceArray)
}
merge([1, 2, 3], [3, 2, 1], { arrayMerge: concatMerge }) // => [1, 2, 3, 3, 2, 1]
Defaults to false
. If clone
is true
then both x
and y
are recursively cloned as part of the merge.
With npm do:
npm install deepmerge
Just want to download the file without using any package managers/bundlers? Click here.
With npm do:
npm test
MIT
FAQs
A library for deep (recursive) merging of Javascript objects
The npm package deepmerge receives a total of 21,399,839 weekly downloads. As such, deepmerge popularity was classified as popular.
We found that deepmerge demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.