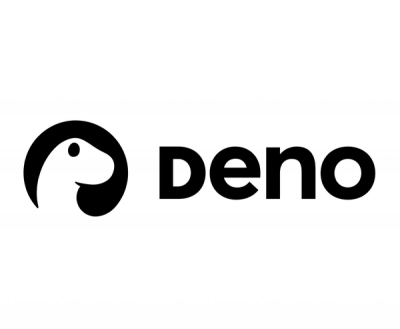
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
deferred-queue
Advanced tools
Node.js project
Version: 0.1.0
A deferred queue enqueues tasks synchronously and executes them asynchronously.
Have you seen the Redis driver? This is how a deferred queue works.
This module is a very lighweight and simplified version of promises. It's meant to be the glue between synchronous api calls and asynchronous executions. Look at the api example to see a representative use case.
This module can be helpful to you if you are exposing an api like the following one:
var Reader = require ("...");
var r = new Reader ("file");
r.read (10, function (error, bytes){
if (error) return console.error (error);
fn1 (bytes);
r.read (20, function (error, bytes){
if (error) return console.error (error);
fn2 (bytes);
r.close (function (error){
if (error) return console.error (error);
fn3 ();
});
});
});
The above example has two problems: the callback nesting and the error handling. With a deferred queue the example can be rewritten as follows:
var Reader = require ("...");
var r = new Reader ("file");
r.on ("error", function (error){
console.error (error);
});
r.read (10, fn1);
r.read (20, fn2);
r.close (fn3);
npm install deferred-queue
module.create() : DeferredQueue
Creates a new DeferredQueue
.
Methods
DeferredQueue#pause() : undefined
Pauses the queue execution.
DeferredQueue#push(task[, callback]) : DeferredQueue
Adds a task and tries to execute it. If there are pending tasks, the task waits until all the previous tasks have been executed.
The task is what you want to execute. The callback is executed with the result of the task.
If the task is asynchronous you don't need to call any callback, simply return. If you want to return an error, throw it, it will be catched. The value that is returned is passed to the callback.
q.push (function (){
return 1;
}, function (error, value){
//error is null
//value is 1
});
q.push (function (){
throw 1;
}, function (error, value){
//error is 1
//value is undefined
});
If the task is asynchronous, a function is passed as parameter. As usual, the error is the first parameter.
q.push (function (cb){
cb (null, 1, 2);
}, function (error, v1, v2){
//error is null
//v1 is 1
//v2 is 2
});
q.push (function (cb){
cb (1);
}, function (error, v1, v2){
//error is 1
//v1 and v2 are undefined
});
There are subtle differences when the tasks are synchronous and asynchronous:
q.push (A);
q.push (function (){
q.push (C);
q.push (D);
});
q.push (B);
If A, B, C, D are asynchronous: A → B → C → D. Asynchronous example.
If A, B, C, D are synchronous: A → C → D → B. Synchronous example.
The error is also emitted with an error
event. The queue is automatically paused, so if you want to resume it you'll need to call to resume().
DeferredQueue#resume() : undefined
Resumes the queue execution from the task it was paused.
DeferredQueue#unshift(task[, callback]) : DeferredQueue
Adds a task to the beginning of the queue. It has the same functionality as the push() function.
FAQs
Series control flow library
The npm package deferred-queue receives a total of 83 weekly downloads. As such, deferred-queue popularity was classified as not popular.
We found that deferred-queue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.