What is dependency-cruiser?
dependency-cruiser is a tool to analyze and visualize the dependencies in your JavaScript and TypeScript projects. It helps you understand the structure of your codebase, identify potential issues, and enforce architectural rules.
What are dependency-cruiser's main functionalities?
Dependency Graph Generation
Generates a dependency graph for the specified source directory. This helps in visualizing the dependencies between different modules in your project.
const depCruiser = require('dependency-cruiser');
const result = depCruiser.cruise(['src']);
console.log(result.output);
Enforcing Architectural Rules
Allows you to define and enforce architectural rules, such as preventing circular dependencies. This helps maintain a clean and manageable codebase.
const depCruiser = require('dependency-cruiser');
const config = {
forbidden: [
{
name: 'no-circular',
severity: 'error',
comment: 'Circular dependencies are not allowed',
from: {},
to: {
circular: true
}
}
]
};
const result = depCruiser.cruise(['src'], config);
console.log(result.output);
Reporting
Generates reports in various formats (e.g., JSON, HTML) to help you analyze the dependency structure and identify potential issues.
const depCruiser = require('dependency-cruiser');
const result = depCruiser.cruise(['src'], {}, { outputType: 'json' });
console.log(JSON.stringify(result.output, null, 2));
Other packages similar to dependency-cruiser
madge
Madge is a JavaScript library that visualizes the module dependency graph of your project. It can detect circular dependencies and generate visual graphs. Compared to dependency-cruiser, Madge is more focused on visualization and less on enforcing architectural rules.
depcheck
Depcheck is a tool that helps you find unused dependencies in your project. While it doesn't provide the same level of dependency graph visualization as dependency-cruiser, it is useful for cleaning up your package.json file by identifying dependencies that are no longer in use.
webpack-bundle-analyzer
Webpack Bundle Analyzer is a tool that visualizes the size of webpack output files with an interactive zoomable treemap. It is more focused on analyzing the size and composition of your webpack bundles rather than the dependency structure of your source code.
Dependency cruiser 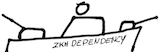
Validate and visualize dependencies. With your rules. JavaScript. TypeScript. CoffeeScript. ES6, CommonJS, AMD.
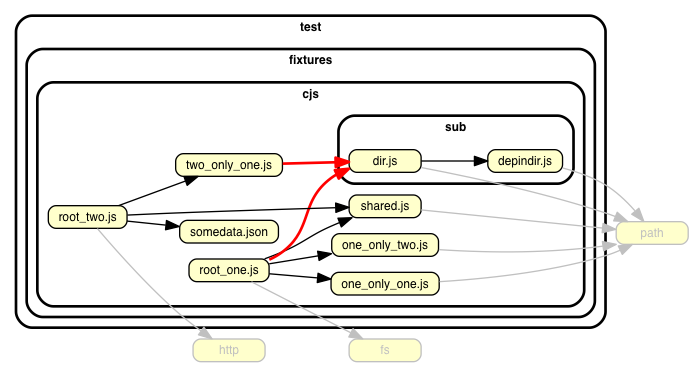
What's this do?
- Run through the dependencies in any JavaScript, TypeScript or CoffeeScript project and ...
- ... validate them against (your own) rules
- ... report violated rules
- in text (for your builds)
- in graphics (for your eyeballs)
As a side effect it can generate cool dependency graphs
you can stick on the wall to impress your grandma.
How do I use it?
Install it
Dependency cruiser works most comfortably when you install it globally.
npm install --global dependency-cruiser
Show stuff
To create a graph of the dependencies in your src folder, you'd run dependency
cruiser with output type dot
and run GraphViz dot on the result. In
a one liner:
depcruise --exclude "^node_modules" --output-type dot src | dot -T svg > dependencygraph.svg
The --exclude "^node_modules"
makes sure dependency-cruiser does not scan
paths starting with node_modules.
- You can read more about what you can do with
--exclude
and other command line
options in the
command line interface
documentation. - Real world samples
contains dependency cruises of some of the most used projects on npm.
Validate stuff
Declare some rules
To have dependency-cruiser report on dependencies going into the test folder
(which is totally weird, right?) create a rules file (e.g. my-rules.json
)
and put this in there:
{
"forbidden": [{
"name": "not-to-test",
"comment": "don't allow dependencies from outside the test folder to test",
"severity": "error",
"from": { "pathNot": "^test" },
"to": { "path": "^test" }
}]
}
- To read more about writing rules check the
writing rules
section.
- There is practical rules configuration to get you started
here
Report them
Pass the --validate
parameter, to the command line followed by the rules
file.
Most output-types will show violations of your rules in one way or another.
The dot
reporter, for instance, will color edges representing violated
dependencies in a signaling color (red for errors, orange for warnings) - the
picture on top of this README is a sample of that.
The err
reporter only emits (text) output when there's something wrong.
This is useful when you want to check the rules in your build process:
depcruise --validate my-rules.json --output-type err src

- Read more about the err, dot, but also the csv and html reporters in the
command line interface
documentation.
- dependency-cruiser uses itself to check on itself in its own build process;
see the
dependency-cruise
target in the
Makefile
I want to know more!
You've come to the right place :-) :
License
MIT
Thanks
- Marijn Haverbeke and other people who
colaborated on acorn -
the excelent javascript parser dependency-cruiser uses to infer
dependencies.
