What is dependency-cruiser?
dependency-cruiser is a tool to analyze and visualize the dependencies in your JavaScript and TypeScript projects. It helps you understand the structure of your codebase, identify potential issues, and enforce architectural rules.
What are dependency-cruiser's main functionalities?
Dependency Graph Generation
Generates a dependency graph for the specified source directory. This helps in visualizing the dependencies between different modules in your project.
const depCruiser = require('dependency-cruiser');
const result = depCruiser.cruise(['src']);
console.log(result.output);
Enforcing Architectural Rules
Allows you to define and enforce architectural rules, such as preventing circular dependencies. This helps maintain a clean and manageable codebase.
const depCruiser = require('dependency-cruiser');
const config = {
forbidden: [
{
name: 'no-circular',
severity: 'error',
comment: 'Circular dependencies are not allowed',
from: {},
to: {
circular: true
}
}
]
};
const result = depCruiser.cruise(['src'], config);
console.log(result.output);
Reporting
Generates reports in various formats (e.g., JSON, HTML) to help you analyze the dependency structure and identify potential issues.
const depCruiser = require('dependency-cruiser');
const result = depCruiser.cruise(['src'], {}, { outputType: 'json' });
console.log(JSON.stringify(result.output, null, 2));
Other packages similar to dependency-cruiser
madge
Madge is a JavaScript library that visualizes the module dependency graph of your project. It can detect circular dependencies and generate visual graphs. Compared to dependency-cruiser, Madge is more focused on visualization and less on enforcing architectural rules.
depcheck
Depcheck is a tool that helps you find unused dependencies in your project. While it doesn't provide the same level of dependency graph visualization as dependency-cruiser, it is useful for cleaning up your package.json file by identifying dependencies that are no longer in use.
webpack-bundle-analyzer
Webpack Bundle Analyzer is a tool that visualizes the size of webpack output files with an interactive zoomable treemap. It is more focused on analyzing the size and composition of your webpack bundles rather than the dependency structure of your source code.
Dependency cruiser 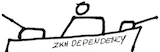
Validate and visualise dependencies. With your rules. JavaScript. TypeScript. CoffeeScript. ES6, CommonJS, AMD.
What's this do?
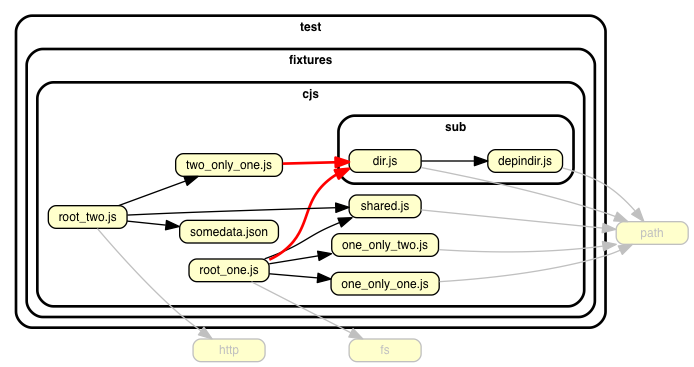
This runs through the dependencies in any JavaScript, TypeScript, LiveScript or CoffeeScript project and ...
- ... validates them against (your own) rules
- ... reports violated rules
- in text (for your builds)
- in graphics (for your eyeballs)
As a side effect it can generate dependency graphs in various output formats including cool visualizations
you can stick on the wall to impress your grandma.
How do I use it?
Install it ...
npm install --save-dev dependency-cruiser
# or
yarn add -D dependency-cruiser
pnpm add -D dependency-cruiser
... and generate a config
npx depcruise --init
This will look around in your environment a bit, ask you some questions and create
a .dependency-cruiser.js
configuration file attuned to your project12.
Show stuff to your grandma
To create a graph of the dependencies in your src folder, you'd run dependency
cruiser with output type dot
and run GraphViz dot3 on the result. In
a one liner:
npx depcruise src --include-only "^src" --output-type dot | dot -T svg > dependency-graph.svg
dependency-cruiser v12 and older: add --config option
While not necessary from dependency-cruiser v13 and later, in v12 and older
you'll have to pass the --config option to make it find the .dependency-cruiser.js
configuration file:
npx depcruise src --include-only "^src" --config --output-type dot | dot -T svg > dependency-graph.svg
- You can read more about what you can do with
--include-only
and other command line
options in the command line interface documentation. - Real world samples
contains dependency cruises of some of the most used projects on npm.
- If your grandma is more into formats like
mermaid
, json
, csv
, html
or plain text
we've got her covered
as well.
Validate things
Declare some rules
When you ran depcruise --init
above, the command also added some rules
to .dependency-cruiser.js
that make sense in most projects, like detecting
circular dependencies, dependencies missing in package.json, orphans,
and production code relying on dev- or optionalDependencies.
Start adding your own rules by tweaking that file.
Sample rule:
{
"forbidden": [
{
"name": "not-to-test",
"comment": "don't allow dependencies from outside the test folder to test",
"severity": "error",
"from": { "pathNot": "^test" },
"to": { "path": "^test" }
}
]
}
Report them
npx depcruise src
dependency-cruiser v12 and older: add --config option
While not necessary from dependency-cruiser v13, in v12 and older you'll have
to pass the --config option to make it find the .dependency-cruiser.js
configuration file:
npx depcruise --config .dependency-cruiser.js src
This will validate against your rules and shows any violations in an eslint-like format:

There's more ways to report validations; in a graph (like the one on top of this
readme) or in an self-containing html
file.
- Read more about the err, dot, csv and html reporters in the
command line interface
documentation.
- dependency-cruiser uses itself to check on itself in its own build process;
see the
depcruise
script in the
package.json
I want to know more!
You've come to the right place :-) :
- Usage
- Hacking on dependency-cruiser
- Other things
License
MIT
Thanks
- Marijn Haverbeke and other people who
collaborated on acorn -
the excellent JavaScript parser dependency-cruiser uses to infer
dependencies.
- Katerina Limpitsouni of unDraw
for the ollie in dependency-cruiser's
social media image.
- All members of the open source community who have been kind enough to raise issues,
ask questions and make pull requests to get dependency-cruiser to be a better
tool.
Build status

Made with :metal: in Holland.