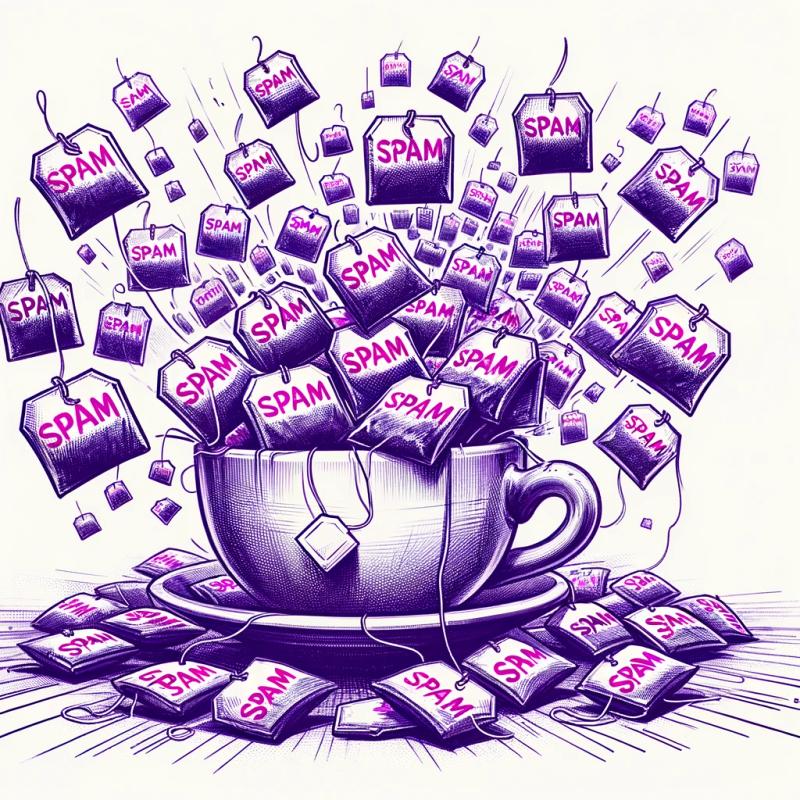
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
deprecate
Advanced tools
Readme
Mark a method as deprecated. Write a message to a stream the first time the deprecated method is called.
var deprecate = require('deprecate');
Call deprecate
within a function you are deprecating. It will spit out all the messages to the console the first time and only the first time the method is called.
var deprecate = require('deprecate');
var someDeprecatedFunction = function() {
deprecate('someDeprecatedFunction() is deprecated');
};
someDeprecatedFunction();
someDeprecatedFunction();
someDeprecatedFunction();
console.log('end');
//program output:
WARNING!!
someDeprecatedFunction() is deprecated
end
Set to false
to not output a color. Defaults to '\x1b[31;1m'
which is red.
Set to false
to do nothing at all when the deprecate method is called. Useful in tests of the library you're deprecating things within.
The stream to which output is written. Defaults to process.stderr
MIT
FAQs
Mark methods as deprecated and warn the user when they're called
The npm package deprecate receives a total of 151,014 weekly downloads. As such, deprecate popularity was classified as popular.
We found that deprecate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.