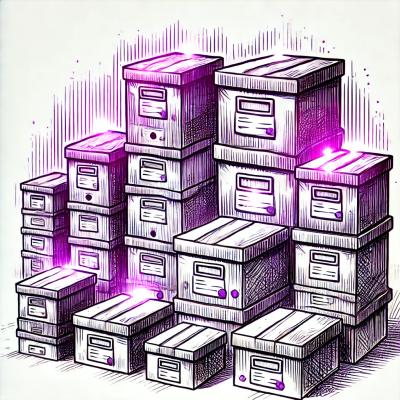
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
devrant-lite
Advanced tools
Tiny, fully-featured REST client for the unofficial devRant API.
I have built this library because existing ones have not been recently maintained, or don't support all existing endpoints.
yarn add devrant-lite
or
npm install devrant-lite
Then you can include the following at the top of your code:
import DevRant from 'devrant-lite';
const client = DevRant.withCredentials('username', 'password');
client.get(...)
client.post(...)
You will need to create an account on https://devrant.com/, if you haven't already.
Otherwise, you will only have access to some public endpoints, like devrant/rants
.
There are three ways of authentication:
No Authentication:
const client = new DevRant();
const { rants } = await client.get('devrant/rants');
With User Credentials:
I recommend grabbing the auth token with the
.getAuthToken()
method afterwards and using the.withAuthToken()
method next time.
const client = DevRant.withCredentials('username', 'password');
const { data } = await client.get('users/me/notif-feed', {
last_time: 0
});
With Auth Token:
const client = DevRant.withAuthToken({
key: '123abcdefghijklmnopqrstuvxyz',
id: 12345678,
user_id: 12345
});
const { data } = await client.get('users/me/notif-feed', {
last_time: 0
});
new DevRant(options)
The constructor accepts an options
object with the following options:
options.baseUrl
Sets the base url for the api.
Useful for proxying or testing.
Default: 'https://devrant.com/api'
options.app
Sets the app ID to use.
The app id will be sent with every request.
Default: 3
options.plat
Similar as the app ID, this sets the platform ID,
which will also be sent with every request.
Default: 3
DevRant.withCredentials(username, password, options)
A helper function which creates a new instance of the DevRant
class and then sends a request to the users/auth-token
endpoint to get an auth token.
When the request was successful it sets the auth token with the setAuthToken(auth_token)
method.
DevRant.withAuthToken(auth_token, options)
A helper function which creates a new instance of the DevRant
class and then sets the auth token with the setAuthToken(auth_token)
method.
client.get(endpoint, parameters)
Use the post
method for actions that get state.
Returns a Promise resolving to the API response object, or rejecting on error.
The response and error objects will also have a hidden headers
property with the HTTP response headers returned by
the devRant API.
const client = DevRant.withCredentials('username', 'password');
// Get todays top rants
const { rants } = await client.get("devrant/rants", {
sort: 'top',
range: 'day',
limit: 20,
skip: 0,
});
client.post(endpoint, parameters)
Same return as get()
.
Use the post
method for actions that change or create state.
For example, to post a new rant:
const client = DevRant.withCredentials('username', 'password');
// Post a rant with text "Hello World!"
await client.post('devrant/rants', {
rant: 'Hello World!',
tags: 'hello, world',
type: 6
});
client.delete(endpoint, query_parameters)
Same return as get()
and post()
.
Use the delete()
method for actions that delete state. For example, to delete a rant:
const client = DevRant.withCredentials('username', 'password');
// Delete rant with ID 12345
await client.delete('devrant/rants/12345');
client.setAuthToken(auth_token)
Sets the auth token to be used for authentication. An auth token object consists of the id
, key
and user_id
properties.
client.getAuthToken()
Returns the auth token used for authentication.
You can find many more examples for various resources/endpoints in the tests.
const response = await client.get('devrant/rants');
console.log(`Content Length: ${response.headers.get('Content-Type')}`);
console.log(response.rants[0]);
.get()
, .post()
and .delete()
reject on error, so you can use try/catch to handle errors. The error object contains an error
property with the error message and the default success
property,
which is false
, in this case. The error object will also have a hidden headers
property with the HTTP response headers returned by the devRant API.
try {
const response = await client.get("some/endpoint");
// ... use response here ...
} catch (e) {
if ('success' in e) {
// devRant API error
console.error(e.error);
} else {
// non-API error, e.g. network problem or invalid JSON in response
}
}
yarn/npm install
DEVRANT_USERNAME=...
DEVRANT_PASSWORD=...
yarn/npm test
and make sure all tests passgit push
and submit your PR!Authors:
FAQs
Tiny, fully-featured REST client for the unofficial devRant API
The npm package devrant-lite receives a total of 1 weekly downloads. As such, devrant-lite popularity was classified as not popular.
We found that devrant-lite demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.