Comparing version 2.2.7 to 2.2.8
@@ -6,6 +6,2 @@ import { DPoint } from './DPoint'; | ||
r: number; | ||
/** | ||
* @param [center=(0,0)] | ||
* @param [r=0] | ||
*/ | ||
constructor(center?: DPoint, r?: number); | ||
@@ -18,31 +14,7 @@ toString(): string; | ||
clone(): DCircle; | ||
/** | ||
* Find intersection points with other circle. | ||
* | ||
*  | ||
* @param c | ||
*/ | ||
findPoints(c: DCircle): DPoint[] | number; | ||
equal({ center, r }: DCircle): boolean; | ||
/** | ||
* Transform circle to polygon | ||
* | ||
*  | ||
* @param [pointCount=64] | ||
*/ | ||
findPolygonInside(pointCount?: number): DPolygon; | ||
/** | ||
* Transform circle to polygon on sphere. It would be different for different latitude. | ||
* | ||
* @remarks | ||
* Center should be Lng/Lat. | ||
* | ||
* @remarks | ||
* Radius should be in meters. | ||
* | ||
* 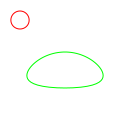 | ||
* @param [pointCount=64] | ||
*/ | ||
findPolygonInsideOnSphere(pointCount?: number): DPolygon; | ||
private sphereOffset; | ||
} |
@@ -8,9 +8,3 @@ "use strict"; | ||
const utils_1 = require("./utils"); | ||
// eslint-disable-next-line padded-blocks | ||
class DCircle { | ||
/** | ||
* @param [center=(0,0)] | ||
* @param [r=0] | ||
*/ | ||
// eslint-disable-next-line no-useless-constructor,no-empty-function | ||
constructor(center = DPoint_1.DPoint.zero(), r = 0) { | ||
@@ -29,8 +23,2 @@ this.center = center; | ||
} | ||
/** | ||
* Find intersection points with other circle. | ||
* | ||
*  | ||
* @param c | ||
*/ | ||
findPoints(c) { | ||
@@ -68,8 +56,2 @@ if (this.equal(c)) { | ||
} | ||
/** | ||
* Transform circle to polygon | ||
* | ||
*  | ||
* @param [pointCount=64] | ||
*/ | ||
findPolygonInside(pointCount = 64) { | ||
@@ -86,14 +68,2 @@ const preAngle = 2 * Math.PI / pointCount; | ||
} | ||
/** | ||
* Transform circle to polygon on sphere. It would be different for different latitude. | ||
* | ||
* @remarks | ||
* Center should be Lng/Lat. | ||
* | ||
* @remarks | ||
* Radius should be in meters. | ||
* | ||
* 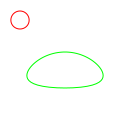 | ||
* @param [pointCount=64] | ||
*/ | ||
findPolygonInsideOnSphere(pointCount = 64) { | ||
@@ -100,0 +70,0 @@ (0, utils_1.checkFunction)('findPolygonInsideOnSphere') |
@@ -9,9 +9,2 @@ import { DPoint } from './DPoint'; | ||
end: DPoint; | ||
/** | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param [begin=DPoint.zero()] | ||
* @param [end=DPoint.zero()] | ||
*/ | ||
constructor(a: number, b: number, c: number, begin?: DPoint, end?: DPoint); | ||
@@ -21,22 +14,5 @@ clone(): DLine; | ||
perpendicularDistance(p: DPoint): number; | ||
/** | ||
* Find intersection of two lines segments. | ||
* For intersection of two lines use [[findPoint]] | ||
* @param l | ||
* @param [d=0] | ||
* @param [includeOnly=false] | ||
*/ | ||
intersection(l: DLine, d?: number, includeOnly?: boolean): DPoint | null; | ||
intersectionWithCircle(circle: DCircle): DPoint | [DPoint, DPoint] | null; | ||
/** | ||
* Check if point below to line segment | ||
* @param p | ||
* @param d | ||
*/ | ||
inRange(p: DPoint, d?: number): boolean; | ||
/** | ||
* Check if point below to line segment, but not equal star or end point. | ||
* @param p | ||
* @param [d=0] | ||
*/ | ||
insideRange(p: DPoint, d?: number): boolean; | ||
@@ -50,50 +26,14 @@ get center(): DPoint; | ||
getValue(): number[]; | ||
/** | ||
* Find `x` from `y` value of point | ||
* @param p | ||
*/ | ||
x(p: DPoint): DPoint; | ||
/** | ||
* Find `y` from `x` value of point | ||
* @param p | ||
*/ | ||
y(p: DPoint): DPoint; | ||
/** | ||
* Find intersection of two lines. | ||
* For intersection of two lines segments use [[intersection]] | ||
* @param l | ||
*/ | ||
findPoint(l: DLine): DPoint | null; | ||
/** | ||
* Check if line parallel to `x` or `y` axis | ||
*/ | ||
get isParallel(): boolean; | ||
get isParallelY(): boolean; | ||
get isParallelX(): boolean; | ||
/** | ||
* Get lines segment start and end points as array | ||
*/ | ||
get points(): [DPoint, DPoint]; | ||
/** | ||
* Get line segment direction (from start point to end point) | ||
*/ | ||
getFi(): number; | ||
toWKT(): string; | ||
/** | ||
* Move lines point to left or right | ||
* @param p | ||
* @param d | ||
*/ | ||
movePoint(p: DPoint, d: number): DPoint; | ||
/** | ||
* Find angle between current line and line in argument | ||
* @param l | ||
* @param delta | ||
*/ | ||
findFi({ a, b }: DLine, delta?: number): number; | ||
/** | ||
* [Cross product](https://en.wikipedia.org/wiki/Cross_product) | ||
* @param l {DLine} | ||
*/ | ||
vectorProduct({ a, b, c }: DLine): DLine; | ||
} |
@@ -6,15 +6,4 @@ "use strict"; | ||
const utils_1 = require("./utils"); | ||
// eslint-disable-next-line padded-blocks | ||
class DLine { | ||
/** | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param [begin=DPoint.zero()] | ||
* @param [end=DPoint.zero()] | ||
*/ | ||
// eslint-disable-next-line no-useless-constructor | ||
constructor(a, b, c, begin = DPoint_1.DPoint.zero(), end = DPoint_1.DPoint.zero() | ||
// eslint-disable-next-line no-empty-function | ||
) { | ||
constructor(a, b, c, begin = DPoint_1.DPoint.zero(), end = DPoint_1.DPoint.zero()) { | ||
this.a = a; | ||
@@ -51,9 +40,2 @@ this.b = b; | ||
} | ||
/** | ||
* Find intersection of two lines segments. | ||
* For intersection of two lines use [[findPoint]] | ||
* @param l | ||
* @param [d=0] | ||
* @param [includeOnly=false] | ||
*/ | ||
intersection(l, d = 0, includeOnly = false) { | ||
@@ -85,3 +67,2 @@ const p = this.findPoint(l); | ||
const move = Math.sqrt(r * r - ct * ct); | ||
// Mean "|" x = const | ||
if (this.isParallelY) { | ||
@@ -93,3 +74,2 @@ t.x = this.begin.x; | ||
} | ||
// Mean "-" y = const | ||
if (this.isParallelX) { | ||
@@ -122,7 +102,2 @@ t.y = this.begin.y; | ||
} | ||
/** | ||
* Check if point below to line segment | ||
* @param p | ||
* @param d | ||
*/ | ||
inRange(p, d = 0) { | ||
@@ -135,7 +110,2 @@ const { minX, minY, maxX, maxY } = this; | ||
} | ||
/** | ||
* Check if point below to line segment, but not equal star or end point. | ||
* @param p | ||
* @param [d=0] | ||
*/ | ||
insideRange(p, d = 0) { | ||
@@ -176,6 +146,2 @@ const { begin, end } = this; | ||
} | ||
/** | ||
* Find `x` from `y` value of point | ||
* @param p | ||
*/ | ||
x(p) { | ||
@@ -190,6 +156,2 @@ if (this.isParallelY) { | ||
} | ||
/** | ||
* Find `y` from `x` value of point | ||
* @param p | ||
*/ | ||
y(p) { | ||
@@ -204,7 +166,2 @@ if (this.isParallelY) { | ||
} | ||
/** | ||
* Find intersection of two lines. | ||
* For intersection of two lines segments use [[intersection]] | ||
* @param l | ||
*/ | ||
findPoint(l) { | ||
@@ -245,5 +202,2 @@ if (this.isParallelY && l.isParallelY) { | ||
} | ||
/** | ||
* Check if line parallel to `x` or `y` axis | ||
*/ | ||
get isParallel() { | ||
@@ -258,11 +212,5 @@ return this.isParallelX || this.isParallelY; | ||
} | ||
/** | ||
* Get lines segment start and end points as array | ||
*/ | ||
get points() { | ||
return [this.begin, this.end]; | ||
} | ||
/** | ||
* Get line segment direction (from start point to end point) | ||
*/ | ||
getFi() { | ||
@@ -285,7 +233,2 @@ (0, utils_1.checkFunction)('getFi') | ||
} | ||
/** | ||
* Move lines point to left or right | ||
* @param p | ||
* @param d | ||
*/ | ||
movePoint(p, d) { | ||
@@ -307,7 +250,2 @@ const fi = this.findFi(new DLine(1, 0, 0)); | ||
} | ||
/** | ||
* Find angle between current line and line in argument | ||
* @param l | ||
* @param delta | ||
*/ | ||
findFi({ a, b }, delta = 1.0001) { | ||
@@ -324,6 +262,2 @@ const { a: q, b: w } = this; | ||
} | ||
/** | ||
* [Cross product](https://en.wikipedia.org/wiki/Cross_product) | ||
* @param l {DLine} | ||
*/ | ||
vectorProduct({ a, b, c }) { | ||
@@ -330,0 +264,0 @@ const { a: q, b: w, c: e } = this; |
@@ -22,23 +22,5 @@ import { DLine } from './DLine'; | ||
}; | ||
/** | ||
* Create point with zero coords `(0, 0)` | ||
*/ | ||
constructor(); | ||
/** | ||
* Create point | ||
* @param xy - `x` and `y` value | ||
*/ | ||
constructor(xy: number); | ||
/** | ||
* Create point | ||
* @param x - lng, meters to East, radians to East or width | ||
* @param y - lat, meters to North, radians to North or height | ||
*/ | ||
constructor(x: number, y: number); | ||
/** | ||
* Create point | ||
* @param x - lng, meters to East, radians to East or width | ||
* @param y - lat, meters to North, radians to North or height | ||
* @param z - height | ||
*/ | ||
constructor(x: number, y: number, z?: number); | ||
@@ -49,23 +31,5 @@ static zero(): DPoint; | ||
static random(): DPoint; | ||
/** | ||
* @remark Point should be Lng/Lat. | ||
* | ||
* @remark `z` value default for `zoom` argument. | ||
* | ||
* @param [zoom=this.z] | ||
*/ | ||
getTileFromCoords(zoom?: number): DPoint; | ||
/** | ||
* Result would be Lng/Lat. | ||
* | ||
* @remark `z` value default for `zoom` argument. | ||
* | ||
* @param [zoom=this.z] | ||
*/ | ||
getCoordsFromTile(zoom?: number): DPoint; | ||
toCoords(): DCoord; | ||
/** | ||
* Find line between two points. | ||
* @param p | ||
*/ | ||
findLine(p: DPoint): DLine; | ||
@@ -78,31 +42,7 @@ findInnerAngle(p1: DPoint, p3: DPoint): number; | ||
distance(p: DPoint): number; | ||
/** | ||
* Set `x` value | ||
* @param x | ||
*/ | ||
setX(x: number): DPoint; | ||
/** | ||
* Transform `x` value by function | ||
* @param f | ||
*/ | ||
setX(f: SetterFunction): DPoint; | ||
/** | ||
* Set `z` value | ||
* @param z | ||
*/ | ||
setZ(z: number): DPoint; | ||
/** | ||
* Transform `z` value by function | ||
* @param f | ||
*/ | ||
setZ(f: SetterFunction): DPoint; | ||
/** | ||
* Set `y` value | ||
* @param y | ||
*/ | ||
setY(y: number): DPoint; | ||
/** | ||
* Transform `y` value by function | ||
* @param f | ||
*/ | ||
setY(f: SetterFunction): DPoint; | ||
@@ -114,22 +54,5 @@ clone(): DPoint; | ||
ltOrEqual(p: DPoint): boolean; | ||
/** | ||
* Clockwise rotation | ||
* @param a radians | ||
*/ | ||
rotate(a: number): DPoint; | ||
/** | ||
* Add `v` to `x` and `y` | ||
* @param v | ||
*/ | ||
move(v: number): DPoint; | ||
/** | ||
* Add `p.x` to `x` field and `p.y` to `y` field. | ||
* @param p | ||
*/ | ||
move(p: DPoint): DPoint; | ||
/** | ||
* Add `x` to `x` field and `y` to `y` field. | ||
* @param x | ||
* @param y | ||
*/ | ||
move(x: number, y: number): DPoint; | ||
@@ -145,66 +68,16 @@ degreeToMeters(): DPoint; | ||
floor(): DPoint; | ||
/** | ||
* @param [n=2] | ||
*/ | ||
toFixed(n?: number): DPoint; | ||
abs(): DPoint; | ||
/** | ||
* Multiply `v` to `x` and `y` | ||
* @param v | ||
*/ | ||
scale(v: number): DPoint; | ||
/** | ||
* Multiply `p.x` to `x` field and `p.y` to `y` field. | ||
* @param p | ||
*/ | ||
scale(p: DPoint): DPoint; | ||
/** | ||
* Multiply `x` to `x` field and `y` to `y` field. | ||
* @param x | ||
* @param y | ||
*/ | ||
scale(x: number, y: number): DPoint; | ||
/** | ||
* Divide `x` and `y` to `v` | ||
* @param v | ||
*/ | ||
divide(v: number): DPoint; | ||
/** | ||
* Divide `x` field to `p.x` and `y` field to `p.y`. | ||
* @param p | ||
*/ | ||
divide(p: DPoint): DPoint; | ||
/** | ||
* Divide `x` field to `x` and `y` field to `y`. | ||
* @param x | ||
* @param y | ||
*/ | ||
divide(x: number, y: number): DPoint; | ||
equal(p: DPoint): boolean; | ||
/** | ||
* @param p | ||
* @param [d=0.001] | ||
*/ | ||
like(p: DPoint, d?: number): boolean; | ||
/** | ||
* Flip vertically | ||
* @param size canvas size | ||
*/ | ||
flipVertically(size: DPoint): DPoint; | ||
/** | ||
* Flip vertically | ||
* @param height canvas height | ||
*/ | ||
flipVertically(height: number): DPoint; | ||
/** | ||
* Check if point looks like radians | ||
*/ | ||
get likeRadians(): boolean; | ||
/** | ||
* Check if point looks like `EPSG:4326` (degrees) | ||
*/ | ||
get likeWorldGeodeticSystem(): boolean; | ||
/** | ||
* Check if point looks like `EPSG:3857` (meters) | ||
*/ | ||
get likePseudoMercator(): boolean; | ||
@@ -229,13 +102,3 @@ get w(): number; | ||
minus(): DPoint; | ||
/** | ||
* Find [orthodromic path](https://en.wikipedia.org/wiki/Great-circle_navigation) between to points. | ||
* | ||
* @remark Points should be Lng/Lat. | ||
* | ||
*  | ||
* | ||
* @param point | ||
* @param [pointsCount=360] | ||
*/ | ||
orthodromicPath(point: DPoint, pointsCount?: number): DPolygon; | ||
} |
@@ -22,3 +22,2 @@ "use strict"; | ||
class DPoint { | ||
// eslint-disable-next-line no-empty-function,no-useless-constructor | ||
constructor(x = 0, y = x, z) { | ||
@@ -28,3 +27,2 @@ this.x = x; | ||
this.z = z; | ||
// eslint-disable-next-line @typescript-eslint/no-explicit-any | ||
this.properties = {}; | ||
@@ -53,9 +51,2 @@ } | ||
} | ||
/** | ||
* @remark Point should be Lng/Lat. | ||
* | ||
* @remark `z` value default for `zoom` argument. | ||
* | ||
* @param [zoom=this.z] | ||
*/ | ||
getTileFromCoords(zoom = this.z) { | ||
@@ -69,9 +60,2 @@ (0, utils_1.checkFunction)('getTileFromCoords') | ||
} | ||
/** | ||
* Result would be Lng/Lat. | ||
* | ||
* @remark `z` value default for `zoom` argument. | ||
* | ||
* @param [zoom=this.z] | ||
*/ | ||
getCoordsFromTile(zoom = this.z) { | ||
@@ -92,6 +76,2 @@ (0, utils_1.checkFunction)('getCoordsFromTile') | ||
} | ||
/** | ||
* Find line between two points. | ||
* @param p | ||
*/ | ||
findLine(p) { | ||
@@ -185,6 +165,2 @@ (0, utils_1.checkFunction)('findLine') | ||
} | ||
/** | ||
* Clockwise rotation | ||
* @param a radians | ||
*/ | ||
rotate(a) { | ||
@@ -273,5 +249,2 @@ const x = this.x * Math.cos(a) - this.y * Math.sin(a); | ||
} | ||
/** | ||
* @param [n=2] | ||
*/ | ||
toFixed(n = 2) { | ||
@@ -320,6 +293,2 @@ this.x = parseFloat(this.x.toFixed(n)); | ||
} | ||
/** | ||
* @param p | ||
* @param [d=0.001] | ||
*/ | ||
like(p, d = 0.001) { | ||
@@ -342,5 +311,2 @@ if (this.equal(p)) { | ||
} | ||
/** | ||
* Check if point looks like radians | ||
*/ | ||
get likeRadians() { | ||
@@ -352,5 +318,2 @@ if (radiansPolygon.length === 0) { | ||
} | ||
/** | ||
* Check if point looks like `EPSG:4326` (degrees) | ||
*/ | ||
get likeWorldGeodeticSystem() { | ||
@@ -362,5 +325,2 @@ if (worldGeodeticPolygon.length === 0) { | ||
} | ||
/** | ||
* Check if point looks like `EPSG:3857` (meters) | ||
*/ | ||
get likePseudoMercator() { | ||
@@ -434,12 +394,2 @@ if (pseudoMercatorPolygon.length === 0) { | ||
} | ||
/** | ||
* Find [orthodromic path](https://en.wikipedia.org/wiki/Great-circle_navigation) between to points. | ||
* | ||
* @remark Points should be Lng/Lat. | ||
* | ||
*  | ||
* | ||
* @param point | ||
* @param [pointsCount=360] | ||
*/ | ||
orthodromicPath(point, pointsCount = 360) { | ||
@@ -446,0 +396,0 @@ (0, utils_1.checkFunction)('orthodromicPath') |
@@ -14,49 +14,8 @@ /// <reference types="offscreencanvas" /> | ||
constructor(pPoints?: DPoint[]); | ||
/** | ||
* Specifies a round line buffer end cap style. | ||
*/ | ||
static CAP_ROUND: number; | ||
/** | ||
* Specifies a flat line buffer end cap style. | ||
*/ | ||
static CAP_FLAT: number; | ||
/** | ||
* Specifies a square line buffer end cap style. | ||
*/ | ||
static CAP_SQUARE: number; | ||
/** | ||
* Transform array of triangles to Three.JS vertices | ||
* | ||
* ``` | ||
* const geometry = new THREE.BufferGeometry(); | ||
* // create a simple square shape. We duplicate the top left and bottom right | ||
* // vertices because each vertex needs to appear once per triangle. | ||
* const vertices = new Float32Array( DPolygon.arrayOfTrianglesToVertices(triangles, 10) ); | ||
* | ||
* // itemSize = 3 because there are 3 values (components) per vertex | ||
* geometry.setAttribute( 'position', new THREE.BufferAttribute( vertices, 3 ) ); | ||
* const material = new THREE.MeshBasicMaterial( { color: 0xff0000 } ); | ||
* mesh = new THREE.Mesh( geometry, material ); | ||
* | ||
* scene.add( mesh ); | ||
* ``` | ||
* | ||
* @param triangles | ||
* @param [height=0] | ||
*/ | ||
static arrayOfTrianglesToVertices(triangles: DPolygon[], height?: number): number[]; | ||
/** | ||
* Get size of min area rectangle. | ||
* @param poly should be `minAreaRectangle` | ||
*/ | ||
static minAreaRectangleSize(poly: DPolygon): DPoint; | ||
/** | ||
* Slice line string to dashes. | ||
* @param poly should be `divideToPieces` at first | ||
*/ | ||
static toDash(poly: DPolygon): DPolygon[]; | ||
/** | ||
* Get min area rectangle direction | ||
* @param poly should be `minAreaRectangle` | ||
*/ | ||
static minAreaRectangleDirection(poly: DPolygon): number; | ||
@@ -72,115 +31,30 @@ static parseFromWKT(wkt: string): DPolygon; | ||
get minY(): number; | ||
/** | ||
* Get center coordinates | ||
*/ | ||
get center(): DPoint; | ||
/** | ||
* Difference between `maxY` and `minY`. Equal `ΔY` (`dY`) | ||
*/ | ||
get h(): number; | ||
/** | ||
* Difference between `maxX` and `minX`. Equal `ΔX` (`dX`) | ||
*/ | ||
get w(): number; | ||
/** | ||
* Difference between `maxY` and `minY`. Equal `height` (`h`) | ||
*/ | ||
get dY(): number; | ||
/** | ||
* Difference between `maxX` and `minX`. Equal `width` (`w`) | ||
*/ | ||
get dX(): number; | ||
/** | ||
* Get closed extend polygon | ||
*/ | ||
get extend(): DPolygon; | ||
/** | ||
* Point with `width` value as `x` and `height` value as `y` | ||
*/ | ||
get size(): DPoint; | ||
/** | ||
* Point with minimal `x` and `y` | ||
*/ | ||
get leftTop(): DPoint; | ||
/** | ||
* Point with maximal `x` and `y` | ||
*/ | ||
get rightBottom(): DPoint; | ||
/** | ||
* Next point index | ||
*/ | ||
get length(): number; | ||
/** | ||
* Get length of line string. | ||
*/ | ||
get fullLength(): number; | ||
/** | ||
* Get perimeter. | ||
*/ | ||
get perimeter(): number; | ||
/** | ||
* Get polygon area | ||
*/ | ||
get area(): number; | ||
/** | ||
* Get deintesected polygon. | ||
*/ | ||
get deintersection(): DPolygon; | ||
/** | ||
* Check if polygon contain more than three points | ||
*/ | ||
get valid(): boolean; | ||
/** | ||
* Get first point | ||
*/ | ||
get first(): DPoint; | ||
/** | ||
* Get second point | ||
*/ | ||
get second(): DPoint; | ||
/** | ||
* Get last point | ||
*/ | ||
get last(): DPoint; | ||
/** | ||
* Get min area rectangle | ||
*/ | ||
get minAreaRectangle(): DPolygon; | ||
/** | ||
* Get convex polygon | ||
*/ | ||
get convex(): DPolygon; | ||
/** | ||
* Check polygon direction | ||
*/ | ||
get isClockwise(): boolean; | ||
/** | ||
* Get clockwise polygon | ||
*/ | ||
get clockWise(): DPolygon; | ||
/** | ||
* Get polygon clone without holes | ||
*/ | ||
get noHoles(): DPolygon; | ||
/** | ||
* Check polygon intersection with line | ||
* @param l | ||
* @param [includeOnly=false] | ||
*/ | ||
intersection(l: DLine, includeOnly?: boolean): DPoint[]; | ||
/** | ||
* Set polygon center | ||
* @param newCenter | ||
*/ | ||
setCenter(newCenter: DPoint): DPolygon; | ||
static WKT_LINESTRING: string; | ||
static WKT_POLYGON: string; | ||
/** | ||
* @param [type = DPolygon.WKT_POLYGON] Available values `DPolygon.WKT_POLYGON`, `DPolygon.WKT_LINESTRING` | ||
*/ | ||
toWKT(type?: string): string; | ||
/** | ||
* Filter points | ||
* @param f | ||
*/ | ||
filter(f: (p: DPoint) => boolean): DPolygon; | ||
@@ -197,98 +71,28 @@ map(f: (r: DPoint) => DPoint): DPolygon; | ||
toString(): string; | ||
/** | ||
* Add to the end of polygon point equal to first point if it not exist | ||
*/ | ||
close(): DPolygon; | ||
/** | ||
* Remove from the end of polygon point equal to first point if it exist | ||
*/ | ||
open(): DPolygon; | ||
add(poly: DPolygon): DPolygon; | ||
/** | ||
* Check if has point in list of points | ||
* @param p | ||
*/ | ||
has(p: DPoint): boolean; | ||
clone(): DPolygon; | ||
/** | ||
* Check is it fully equal. | ||
* @param p | ||
*/ | ||
equal(p: DPolygon | null): boolean; | ||
/** | ||
* Check is polygons are same. They can be with different directions and different start points. | ||
* @param p | ||
*/ | ||
same(p: DPolygon): boolean; | ||
findIndex(p: DPoint): number; | ||
/** | ||
* Get polygon approximation by | ||
* [Ramer–Douglas–Peucker algorithm](https://en.wikipedia.org/wiki/Ramer%E2%80%93Douglas%E2%80%93Peucker_algorithm) | ||
* @param [e=Math.sqrt(this.perimeter)*APPROXIMATION_VALUE] | ||
*/ | ||
approximation(e?: number): DPolygon; | ||
insertAfter(index: number, ...points: DPoint[]): void; | ||
removePart(index: number, count: number): DPoint[]; | ||
/** | ||
* Check intersection with other polygon | ||
* @param p | ||
*/ | ||
hasSimpleIntersection(p: DPolygon): boolean; | ||
/** | ||
* Check if it possible to include point | ||
* @param p | ||
*/ | ||
simpleInclude(p: DPoint): boolean; | ||
drawPolygonOnCanvas(canvas: HTMLCanvasElement | OffscreenCanvas, fillColor?: string, strokeColor?: string, shadowColor?: string, lineWidth?: number, steps?: number): void; | ||
clearPolygonOnCanvas(canvas: HTMLCanvasElement | OffscreenCanvas): void; | ||
/** | ||
* Check if contain point | ||
* @param p | ||
* @param [isBorderInside=false] | ||
* @param [move=(0,0)] Ignore this parameter | ||
*/ | ||
contain(p: DPoint, isBorderInside?: boolean, move?: DPoint): boolean; | ||
/** | ||
* Check if point on border | ||
* @param p | ||
*/ | ||
onBorder(p: DPoint): boolean; | ||
/** | ||
* Change start point to second point | ||
*/ | ||
nextStart(): DPolygon; | ||
/** | ||
* Remove duplicates points | ||
*/ | ||
removeDuplicates(): DPolygon; | ||
/** | ||
* Parse from [OpenLayers](https://openlayers.org/) coordinates | ||
* @param a | ||
*/ | ||
static parse(a: LatLng[]): DPolygon; | ||
/** | ||
* Parse from [GeoJSON](https://en.wikipedia.org/wiki/GeoJSON) coordinates | ||
* @param a | ||
*/ | ||
static parse(a: number[][]): DPolygon; | ||
/** | ||
* Parse from [OpenLayers](https://openlayers.org/) coordinates | ||
* @param a | ||
*/ | ||
static parse(a: DCoord[]): DPolygon; | ||
/** | ||
* Transform to array of coordinates for [OpenLayers](https://openlayers.org/) or | ||
* [GeoJSON](https://en.wikipedia.org/wiki/GeoJSON) | ||
*/ | ||
toArrayOfCoords(): DCoord[]; | ||
/** | ||
* Divide line string to pieces by length | ||
* @param piecesCount | ||
*/ | ||
divideToPieces(piecesCount: number): DPolygon; | ||
prepareToFastSearch(): void; | ||
fastHas({ x, y, z }: DPoint): boolean; | ||
/** | ||
* Get line string as line string with growing length. For animation. | ||
*/ | ||
get growingPiecesGenerator(): () => Generator<DPolygon, DPolygon>; | ||
@@ -299,18 +103,5 @@ simpleUnion(p: DPolygon): DPolygon | null; | ||
smartUnion(p: DPolygon): DPolygon | null; | ||
/** | ||
* Divide polygon to triangles | ||
* | ||
*  | ||
*/ | ||
toTriangles(): DPolygon[]; | ||
/** | ||
* Divide polygon to triangles and return points indexes | ||
*/ | ||
getTrianglesPointIndexes(): number[]; | ||
get closed(): boolean; | ||
/** | ||
* @param v | ||
* @param [quadrantSegments=64] | ||
* @param [type=DPolygon.CAP_ROUND] DPolygon.CAP_ROUND || DPolygon.CAP_FLAT || DPolygon.CAP_SQUARE | ||
*/ | ||
buffer(v: number, quadrantSegments?: number, type?: number): DPolygon; | ||
@@ -317,0 +108,0 @@ private simpleIncludeX; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.DPolygon = exports.MIN_POINTS_IN_VALID_POLYGON = void 0; | ||
/* eslint-disable max-lines */ | ||
const DPoint_1 = require("./DPoint"); | ||
@@ -17,6 +16,4 @@ const DLine_1 = require("./DLine"); | ||
class DPolygon { | ||
// eslint-disable-next-line no-useless-constructor,no-empty-function | ||
constructor(pPoints = []) { | ||
this.pPoints = pPoints; | ||
// eslint-disable-next-line @typescript-eslint/no-explicit-any | ||
this.properties = {}; | ||
@@ -26,22 +23,2 @@ this.holes = []; | ||
} | ||
/** | ||
* Transform array of triangles to Three.JS vertices | ||
* | ||
* ``` | ||
* const geometry = new THREE.BufferGeometry(); | ||
* // create a simple square shape. We duplicate the top left and bottom right | ||
* // vertices because each vertex needs to appear once per triangle. | ||
* const vertices = new Float32Array( DPolygon.arrayOfTrianglesToVertices(triangles, 10) ); | ||
* | ||
* // itemSize = 3 because there are 3 values (components) per vertex | ||
* geometry.setAttribute( 'position', new THREE.BufferAttribute( vertices, 3 ) ); | ||
* const material = new THREE.MeshBasicMaterial( { color: 0xff0000 } ); | ||
* mesh = new THREE.Mesh( geometry, material ); | ||
* | ||
* scene.add( mesh ); | ||
* ``` | ||
* | ||
* @param triangles | ||
* @param [height=0] | ||
*/ | ||
static arrayOfTrianglesToVertices(triangles, height = 0) { | ||
@@ -55,6 +32,2 @@ return triangles.map((v) => v | ||
} | ||
/** | ||
* Get size of min area rectangle. | ||
* @param poly should be `minAreaRectangle` | ||
*/ | ||
static minAreaRectangleSize(poly) { | ||
@@ -64,6 +37,2 @@ const { first, second, last } = poly.clone().open(); | ||
} | ||
/** | ||
* Slice line string to dashes. | ||
* @param poly should be `divideToPieces` at first | ||
*/ | ||
static toDash(poly) { | ||
@@ -88,6 +57,2 @@ let p = new DPolygon(); | ||
} | ||
/** | ||
* Get min area rectangle direction | ||
* @param poly should be `minAreaRectangle` | ||
*/ | ||
static minAreaRectangleDirection(poly) { | ||
@@ -153,35 +118,17 @@ const { first, second, last } = poly.clone().open(); | ||
} | ||
/** | ||
* Get center coordinates | ||
*/ | ||
get center() { | ||
return this.leftTop.move(this.size.divide(2)); | ||
} | ||
/** | ||
* Difference between `maxY` and `minY`. Equal `ΔY` (`dY`) | ||
*/ | ||
get h() { | ||
return this.maxY - this.minY; | ||
} | ||
/** | ||
* Difference between `maxX` and `minX`. Equal `ΔX` (`dX`) | ||
*/ | ||
get w() { | ||
return this.maxX - this.minX; | ||
} | ||
/** | ||
* Difference between `maxY` and `minY`. Equal `height` (`h`) | ||
*/ | ||
get dY() { | ||
return this.h; | ||
} | ||
/** | ||
* Difference between `maxX` and `minX`. Equal `width` (`w`) | ||
*/ | ||
get dX() { | ||
return this.w; | ||
} | ||
/** | ||
* Get closed extend polygon | ||
*/ | ||
get extend() { | ||
@@ -197,5 +144,2 @@ const { minX, minY, maxX, maxY } = this; | ||
} | ||
/** | ||
* Point with `width` value as `x` and `height` value as `y` | ||
*/ | ||
get size() { | ||
@@ -205,5 +149,2 @@ const { w, h } = this; | ||
} | ||
/** | ||
* Point with minimal `x` and `y` | ||
*/ | ||
get leftTop() { | ||
@@ -213,5 +154,2 @@ const { minX, minY } = this; | ||
} | ||
/** | ||
* Point with maximal `x` and `y` | ||
*/ | ||
get rightBottom() { | ||
@@ -221,17 +159,8 @@ const { maxX, maxY } = this; | ||
} | ||
/** | ||
* Next point index | ||
*/ | ||
get length() { | ||
return this.pPoints.length; | ||
} | ||
/** | ||
* Get length of line string. | ||
*/ | ||
get fullLength() { | ||
return this.clone().open().perimeter; | ||
} | ||
/** | ||
* Get perimeter. | ||
*/ | ||
get perimeter() { | ||
@@ -244,5 +173,2 @@ let p = 0; | ||
} | ||
/** | ||
* Get polygon area | ||
*/ | ||
get area() { | ||
@@ -258,5 +184,2 @@ const closed = this.deintersection; | ||
} | ||
/** | ||
* Get deintesected polygon. | ||
*/ | ||
get deintersection() { | ||
@@ -280,29 +203,14 @@ const p = this.clone().close(); | ||
} | ||
/** | ||
* Check if polygon contain more than three points | ||
*/ | ||
get valid() { | ||
return this.length > exports.MIN_POINTS_IN_VALID_POLYGON; | ||
} | ||
/** | ||
* Get first point | ||
*/ | ||
get first() { | ||
return this.at(0); | ||
} | ||
/** | ||
* Get second point | ||
*/ | ||
get second() { | ||
return this.at(1); | ||
} | ||
/** | ||
* Get last point | ||
*/ | ||
get last() { | ||
return this.at(this.length - 1); | ||
} | ||
/** | ||
* Get min area rectangle | ||
*/ | ||
get minAreaRectangle() { | ||
@@ -355,5 +263,2 @@ const p = this.convex; | ||
} | ||
/** | ||
* Get convex polygon | ||
*/ | ||
get convex() { | ||
@@ -399,5 +304,2 @@ let p = this.clone().open(); | ||
} | ||
/** | ||
* Check polygon direction | ||
*/ | ||
get isClockwise() { | ||
@@ -413,5 +315,2 @@ let sum = 0; | ||
} | ||
/** | ||
* Get clockwise polygon | ||
*/ | ||
get clockWise() { | ||
@@ -423,5 +322,2 @@ if (this.isClockwise) { | ||
} | ||
/** | ||
* Get polygon clone without holes | ||
*/ | ||
get noHoles() { | ||
@@ -432,7 +328,2 @@ const res = this.clone(); | ||
} | ||
/** | ||
* Check polygon intersection with line | ||
* @param l | ||
* @param [includeOnly=false] | ||
*/ | ||
intersection(l, includeOnly = false) { | ||
@@ -451,6 +342,2 @@ const res = []; | ||
} | ||
/** | ||
* Set polygon center | ||
* @param newCenter | ||
*/ | ||
setCenter(newCenter) { | ||
@@ -461,5 +348,2 @@ return this.loop() | ||
} | ||
/** | ||
* @param [type = DPolygon.WKT_POLYGON] Available values `DPolygon.WKT_POLYGON`, `DPolygon.WKT_LINESTRING` | ||
*/ | ||
toWKT(type = DPolygon.WKT_POLYGON) { | ||
@@ -478,6 +362,2 @@ if (type === DPolygon.WKT_POLYGON) { | ||
} | ||
/** | ||
* Filter points | ||
* @param f | ||
*/ | ||
filter(f) { | ||
@@ -520,5 +400,2 @@ this.pPoints = this.pPoints.filter(f); | ||
} | ||
/** | ||
* Add to the end of polygon point equal to first point if it not exist | ||
*/ | ||
close() { | ||
@@ -531,5 +408,2 @@ const p0 = this.first; | ||
} | ||
/** | ||
* Remove from the end of polygon point equal to first point if it exist | ||
*/ | ||
open() { | ||
@@ -547,6 +421,2 @@ const p = this.first; | ||
} | ||
/** | ||
* Check if has point in list of points | ||
* @param p | ||
*/ | ||
has(p) { | ||
@@ -561,6 +431,2 @@ return this.pPoints.some((q) => q.equal(p)); | ||
} | ||
/** | ||
* Check is it fully equal. | ||
* @param p | ||
*/ | ||
equal(p) { | ||
@@ -576,6 +442,2 @@ if (!(p instanceof DPolygon)) { | ||
} | ||
/** | ||
* Check is polygons are same. They can be with different directions and different start points. | ||
* @param p | ||
*/ | ||
same(p) { | ||
@@ -598,7 +460,2 @@ const pClone = p.clone().close(); | ||
} | ||
/** | ||
* Get polygon approximation by | ||
* [Ramer–Douglas–Peucker algorithm](https://en.wikipedia.org/wiki/Ramer%E2%80%93Douglas%E2%80%93Peucker_algorithm) | ||
* @param [e=Math.sqrt(this.perimeter)*APPROXIMATION_VALUE] | ||
*/ | ||
approximation(e = Math.sqrt(this.perimeter) * APPROXIMATION_VALUE) { | ||
@@ -613,6 +470,2 @@ return new DPolygon(this.clone().douglasPeucker(this.pPoints, e)); | ||
} | ||
/** | ||
* Check intersection with other polygon | ||
* @param p | ||
*/ | ||
hasSimpleIntersection(p) { | ||
@@ -627,6 +480,2 @@ const extend1 = this.extend; | ||
} | ||
/** | ||
* Check if it possible to include point | ||
* @param p | ||
*/ | ||
simpleInclude(p) { | ||
@@ -675,8 +524,2 @@ return this.simpleIncludeX(p) && this.simpleIncludeY(p); | ||
} | ||
/** | ||
* Check if contain point | ||
* @param p | ||
* @param [isBorderInside=false] | ||
* @param [move=(0,0)] Ignore this parameter | ||
*/ | ||
contain(p, isBorderInside = false, move = DPoint_1.DPoint.zero()) { | ||
@@ -707,6 +550,2 @@ const simpleInclude = this.simpleInclude(p); | ||
} | ||
/** | ||
* Check if point on border | ||
* @param p | ||
*/ | ||
onBorder(p) { | ||
@@ -732,5 +571,2 @@ const simpleInclude = this.simpleInclude(p); | ||
} | ||
/** | ||
* Change start point to second point | ||
*/ | ||
nextStart() { | ||
@@ -742,5 +578,2 @@ this.open(); | ||
} | ||
/** | ||
* Remove duplicates points | ||
*/ | ||
removeDuplicates() { | ||
@@ -760,13 +593,5 @@ for (let i = 0; i < this.length - 1; i++) { | ||
} | ||
/** | ||
* Transform to array of coordinates for [OpenLayers](https://openlayers.org/) or | ||
* [GeoJSON](https://en.wikipedia.org/wiki/GeoJSON) | ||
*/ | ||
toArrayOfCoords() { | ||
return this.pPoints.map((r) => r.toCoords()); | ||
} | ||
/** | ||
* Divide line string to pieces by length | ||
* @param piecesCount | ||
*/ | ||
divideToPieces(piecesCount) { | ||
@@ -824,9 +649,4 @@ const { fullLength } = this; | ||
} | ||
/** | ||
* Get line string as line string with growing length. For animation. | ||
*/ | ||
get growingPiecesGenerator() { | ||
// eslint-disable-next-line @typescript-eslint/no-this-alias,consistent-this | ||
const polygon = this; | ||
// eslint-disable-next-line func-names | ||
return function* () { | ||
@@ -884,7 +704,2 @@ const r = new DPolygon(); | ||
} | ||
/** | ||
* Divide polygon to triangles | ||
* | ||
*  | ||
*/ | ||
toTriangles() { | ||
@@ -943,5 +758,2 @@ const innerAndNotIntersect = (poly, p1, p2) => { | ||
} | ||
/** | ||
* Divide polygon to triangles and return points indexes | ||
*/ | ||
getTrianglesPointIndexes() { | ||
@@ -1013,7 +825,2 @@ const innerAndNotIntersect = (poly, p1, p2) => { | ||
} | ||
/** | ||
* @param v | ||
* @param [quadrantSegments=64] | ||
* @param [type=DPolygon.CAP_ROUND] DPolygon.CAP_ROUND || DPolygon.CAP_FLAT || DPolygon.CAP_SQUARE | ||
*/ | ||
buffer(v, quadrantSegments = 64, type = DPolygon.CAP_ROUND) { | ||
@@ -1088,3 +895,2 @@ const reader = new jsts_1.io.WKTReader(); | ||
} | ||
// eslint-disable-next-line no-magic-numbers | ||
const eps = Math.PI / 10000; | ||
@@ -1190,15 +996,6 @@ let result = false; | ||
exports.DPolygon = DPolygon; | ||
/** | ||
* Specifies a round line buffer end cap style. | ||
*/ | ||
DPolygon.CAP_ROUND = CAP_ROUND; | ||
/** | ||
* Specifies a flat line buffer end cap style. | ||
*/ | ||
DPolygon.CAP_FLAT = CAP_FLAT; | ||
/** | ||
* Specifies a square line buffer end cap style. | ||
*/ | ||
DPolygon.CAP_SQUARE = CAP_SQUARE; | ||
DPolygon.WKT_LINESTRING = 'LINESTRING'; | ||
DPolygon.WKT_POLYGON = 'POLYGON'; |
@@ -9,61 +9,15 @@ import { DPolygon } from './DPolygon'; | ||
private getLoopFunction; | ||
/** | ||
* Run loop | ||
*/ | ||
run(): DPolygon; | ||
/** | ||
* @param zoom default value would be `z` of point | ||
*/ | ||
getTileFromCoords(zoom?: number): DPolygonLoop; | ||
/** | ||
* @param zoom default value would be `z` of point | ||
*/ | ||
getCoordsFromTile(zoom?: number): DPolygonLoop; | ||
height(z: number): DPolygonLoop; | ||
/** | ||
* Set `x` value | ||
* @param x | ||
*/ | ||
setX(x: number): DPolygonLoop; | ||
/** | ||
* Transform `x` value by function | ||
* @param f | ||
*/ | ||
setX(f: SetterFunction): DPolygonLoop; | ||
/** | ||
* Set `y` value | ||
* @param y | ||
*/ | ||
setY(y: number): DPolygonLoop; | ||
/** | ||
* Transform `y` value by function | ||
* @param f | ||
*/ | ||
setY(f: SetterFunction): DPolygonLoop; | ||
/** | ||
* Set `z` value | ||
* @param z | ||
*/ | ||
setZ(z: number): DPolygonLoop; | ||
/** | ||
* Transform `z` value by function | ||
* @param f | ||
*/ | ||
setZ(f: SetterFunction): DPolygonLoop; | ||
rotate(a: number): DPolygonLoop; | ||
/** | ||
* Add `v` to `x` and `y` | ||
* @param v | ||
*/ | ||
move(v: number): DPolygonLoop; | ||
/** | ||
* Add `p.x` to `x` field and `p.y` to `y` field. | ||
* @param p | ||
*/ | ||
move(p: DPoint): DPolygonLoop; | ||
/** | ||
* Add `x` to `x` field and `y` to `y` field. | ||
* @param x | ||
* @param y | ||
*/ | ||
move(x: number, y: number): DPolygonLoop; | ||
@@ -75,33 +29,7 @@ round(): DPolygonLoop; | ||
abs(): DPolygonLoop; | ||
/** | ||
* Multiply `v` to `x` and `y` | ||
* @param v | ||
*/ | ||
scale(v: number): DPolygonLoop; | ||
/** | ||
* Multiply `p.x` to `x` field and `p.y` to `y` field. | ||
* @param p | ||
*/ | ||
scale(p: DPoint): DPolygonLoop; | ||
/** | ||
* Multiply `x` to `x` field and `y` to `y` field. | ||
* @param x | ||
* @param y | ||
*/ | ||
scale(x: number, y: number): DPolygonLoop; | ||
/** | ||
* Divide `x` and `y` to `v` | ||
* @param v | ||
*/ | ||
divide(v: number): DPolygonLoop; | ||
/** | ||
* Divide `x` field to `p.x` and `y` field to `p.y`. | ||
* @param p | ||
*/ | ||
divide(p: DPoint): DPolygonLoop; | ||
/** | ||
* Divide `x` field to `x` and `y` field to `y`. | ||
* @param x | ||
* @param y | ||
*/ | ||
divide(x: number, y: number): DPolygonLoop; | ||
@@ -121,12 +49,4 @@ degreeToRadians(): DPolygonLoop; | ||
metersToDegree(): DPolygonLoop; | ||
/** | ||
* Flip vertically | ||
* @param size canvas size | ||
*/ | ||
flipVertically(size: DPoint): DPolygonLoop; | ||
/** | ||
* Flip vertically | ||
* @param height canvas height | ||
*/ | ||
flipVertically(height: number): DPolygonLoop; | ||
} |
@@ -36,6 +36,4 @@ "use strict"; | ||
})(LoopFunctions || (LoopFunctions = {})); | ||
// eslint-disable-next-line complexity | ||
const decodePoolRecord = (a, { functionName, pointArg, numberPointArg, numberArg, setterArg }) => { | ||
let res = a; | ||
// eslint-disable-next-line default-case | ||
switch (functionName) { | ||
@@ -162,3 +160,2 @@ case LoopFunctions.getTileFromCoords: | ||
class DPolygonLoop { | ||
// eslint-disable-next-line no-empty-function,no-useless-constructor | ||
constructor(parent) { | ||
@@ -171,11 +168,5 @@ this.parent = parent; | ||
} | ||
/** | ||
* Run loop | ||
*/ | ||
run() { | ||
return this.parent.map(this.getLoopFunction()); | ||
} | ||
/** | ||
* @param zoom default value would be `z` of point | ||
*/ | ||
getTileFromCoords(zoom) { | ||
@@ -188,5 +179,2 @@ this.pool.push({ | ||
} | ||
/** | ||
* @param zoom default value would be `z` of point | ||
*/ | ||
getCoordsFromTile(zoom) { | ||
@@ -193,0 +181,0 @@ this.pool.push({ |
@@ -99,3 +99,2 @@ "use strict"; | ||
while (!p.equal(group.at(0)) || points.length < 2) { | ||
// eslint-disable-next-line no-constant-condition | ||
while (true) { | ||
@@ -102,0 +101,0 @@ const nextValue = getByPosition(m, p.clone().move(traceDirections[direction])); |
@@ -6,6 +6,4 @@ "use strict"; | ||
const DPoint_1 = require("./DPoint"); | ||
// eslint-disable-next-line @typescript-eslint/no-explicit-any | ||
const warn = (...args) => { | ||
if (index_1.DGeo.DEBUG) { | ||
// eslint-disable-next-line no-console | ||
console.warn(...args); | ||
@@ -49,3 +47,2 @@ } | ||
const checkFunction = (funcName) => ({ | ||
// eslint-disable-next-line func-names, object-shorthand | ||
checkArgument: function (argName) { | ||
@@ -52,0 +49,0 @@ if (!index_1.DGeo.DEBUG) { |
{ | ||
"name": "dgeoutils", | ||
"version": "2.2.7", | ||
"version": "2.2.8", | ||
"description": "", | ||
@@ -5,0 +5,0 @@ "scripts": { |
97008
2788