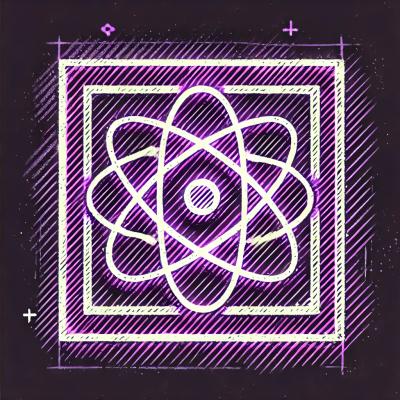
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Dispatches actions on history state changes (ie. push state or navigate).
Install dhistory using npm
:
npm install -s dhistory
Or via jest
:
yarn add dhistory
Define routes and connect to your store:
import { createStore } from 'redux';
import { createBrowserHistory } from 'dhistory';
const routes = {
'/posts/:id': 'VIEW_POST',
'/posts': 'VIEW_POSTS',
'/': 'VIEW_HOME',
};
const store = createStore(reducers, createBrowserHistory(routes));
Note You must not include dhistory in applyMiddleware
, otherwise you will not receive the initial dispatch for the current pathname. You may pass middleware alongside it like so:
import { composeWithBrowserHistory } from 'dhistory';
const store = createStore(reducers, composeWithBrowserHistory(routes)(applyMiddleware(...)));
Inside your reducer:
function reducer(state, action) {
switch (action.type) {
case 'VIEW_POST':
return { ...state, post_id: action.id, view: 'POST' };
case 'VIEW_POSTS':
return { ...state, view: 'POSTS' };
case 'VIEW_HOME':
return { ...state, view: 'HOME' };
...
}
}
To navigate simply dispatch the action, along with any parameters, as you normally would in your Redux app:
dispatch({ type: 'VIEW_POST', id: 1 });
Which will update the location to /posts/1
and dispatch the action. If the path changes outside your application then dhistory will dispatch the same action.
There are many routers and history management libraries but none of them seem to truly fitted with Redux. Navigations don't match with actions or follow the same flow and are treated separate from everything else.
Example with React Router:
import { Router, Route } from 'react-router';
<Router>
<Route to="/post/new" component={NewPostView}/>
</Router>
function createPost(data) {
// some logic to create post
history.push(`/posts/${post.id}`);
return { type: 'POST_CREATED', post };
}
function NewPostView({ store }) {
return (
<PostForm onSubmit={data => store.dispatch(createPost(data))}>
...
</PostForm>
);
}
Example with dhistory:
import { createStore } from 'redux';
import { createBrowserHistory } from 'dhistory';
function createPost(data) {
// some logic to create post
return { type: 'VIEW_POST', id: post.id };
}
const routes = {
'/posts/:id': 'VIEW_POST',
}
const store = createStore(reducers, createBrowserHistory(routes));
function reducer(state, action) {
switch (action.type) {
// This is called when the action is dispatched or when the URL is changed
case 'VIEW_POST':
return { ...state, view: 'POST' };
}
}
function NewPostView({ store }) {
return (
<PostForm onSubmit={data => store.dispatch(createPost(data))}>
...
</PostForm>
);
}
function Main({ view }) {
switch (view) {
case 'POST':
return <NewPostView/>;
...
}
}
createBrowserHistory(routes, options)
and createMemoryHistory(routes, options)
and createHashHistory(routes, options)
Returns a router object.
Returns a function to be passed to createStore
.
const routes = {
'/posts/:id': { type: 'VIEW_POST', extra: 'data' },
'/posts': 'VIEW_POSTS',
}
const store = createStore(reducer, createBrowserHistory(routes));
composeWithBrowserHistory(routes, options)
and composeWithMemoryHistory(routes, options)
and composeWithHashHistory(routes, options)
Same as above but to be used when additional enhancements or middleware.
Returns a compose function to extend the enhancement. ie. add middleware etc.
composeWithBrowserHistory(routes, options)(applyMiddleware(...));
Much of this work wouldn't be made possible without the efforts put to react-router.
FAQs
Dispatches actions on history state changes (ie. push state or navigate).
The npm package dhistory receives a total of 0 weekly downloads. As such, dhistory popularity was classified as not popular.
We found that dhistory demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.