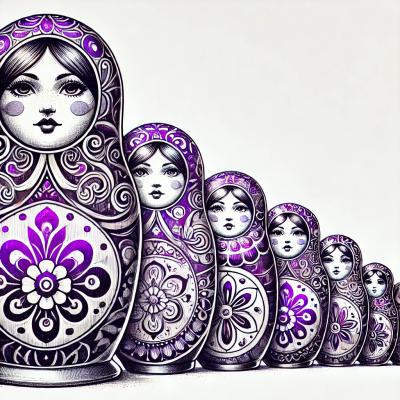
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
This is a JavaScript library for the Dialoqbase API. It is a simple wrapper around the API, providing a more convenient way to interact with it.
First of all, you need to install the library:
npm install dialoqbase
Then you're ready to use it:
import { createClient } from 'dialoqbase';
const dialoqbase = createClient(
'http://localhost:3000',
'your-api-key'
)
const {data, error} = await dialoqbase.bot.create({
name: "Test Bot 2",
model: "claude-3-opus-20240229",
embedding: "nomic-ai/nomic-embed-text-v1.5"
})
const formData = new FormData();
formData.append("file", pdfFile, "test.pdf")
formData.append("file", mp3File, "test.mp3")
const {data, error} = await dialoqbase.bot.source.addFile(botId, formData)
const {data, error} = await dialoqbase.bot.source.add(botId, [
{
content: "https://n4ze3m.com",
type: "website",
},
{
content: "https://www.youtube.com/watch?v=BLmsVvcUxmY",
type: "youtube",
options: {
youtube_mode: "transcript"
}
},
{
content: "Hello World!",
type: "text",
},
])
const chat = await dialoqbase.bot.chat(botId, {
message: "Hello tell me a joke",
stream: true,
history: []
})
for await (const message of chat) {
console.log(message.bot.text)
}
const response = await dialoqbase.bot.chat(botId, {
message: "Hello tell me a joke",
stream: false,
history: []
})
console.log(response.bot.text)
const {data, error} = await dialoqbase.bot.delete(botId)
MIT
FAQs
A client library for Dialoqbase
The npm package dialoqbase receives a total of 0 weekly downloads. As such, dialoqbase popularity was classified as not popular.
We found that dialoqbase demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.