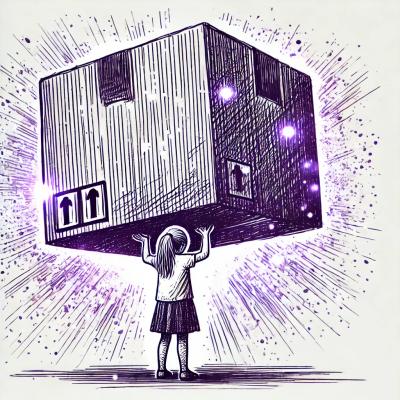
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
diff-ymd-package
Advanced tools
A javascript library for calculating the difference between two dates in formatted ways like (aY bM cD)(aYears bMonths cDays) or customized desired formats like aY-bM-cD or aYears-bMonths-cDays or kDays or mWeeks or nMonths etc.
diff-ymd-package
ajavascript library
provides APIs to difference dates in formatted ways(like (aYears bMonths cDays) or (aY bM cD) etc., eg. age = 20Y 2M 23D or datesDifference = 2Years 11Months 20Days) or customized desired formats like aY-bM-cD or aYears-bMonths-cDays or kDays or mWeeks or nMonths etc.
npm registry
npm install diff-ymd-package
Github Packages registry
npm install @farhan7reza7/diff-ymd-package
Initialize
//const DatesYMD = require('@farhan7reza7/diff-ymd-package'); or
const DatesYMD = require('diff-ymd-package'); // can use any
Create an instance
const date1 = '2022-01-01';
const date2 = '2023-12-31';
const Formatter = new DatesYMD(date1, date2);
OR
for version 2.x.x and above
Can use simplified function diffDates
on module-object(DatesYMD here)
const date1 = '2022-01-01';
const date2 = '2023-12-31';
const Formatter = DatesYMD.diffDates(date1, date2); // can use any
Use methods to format difference
// format output in aY bM cD format
const result = Formatter.formattedYMD(); // Output: "1Y 11M 30D"
// create format of your choice
const customizedFormat = Formatter.customizeFormat('Y', 'Ms', 'Ds', '-'); // Output: "1Y-11Ms-30Ds"
// return an array having years, months, days, and the formatted difference
const resultArray = Formatter.diffArray(); // Output: [1, 11, 30, '1Y 11M 30D']
// Calculate the difference in months
const monthsDifference = Formatter.diffInMonths(); // Output: 23
// Calculate the difference in weeks
const weeksDifference = Formatter.diffInWeeks(); // Output: 164
// Calculate the difference in days
const daysDifference = Formatter.diffInDays(); // Output: 1143
// Calculate the difference in years
const yearsDifference = Formatter.diffInYears(); // Output: 1
// Calculate the difference in hours
const hoursDifference = Formatter.diffInHours(); // Output: 27432
// Calculate the difference in minutes
const minutesDifference = Formatter.diffInMinutes(); // Output: 1645920
// Calculate the difference in seconds
const secondsDifference = Formatter.diffInSeconds(); // Output: 98755200
Formatted Outputs
console.log(result); // Output: "1Y 11M 30D"
// formatted output in aY bM cD format
console.log(customizedFormat); // Output: "1Y-11Ms-30Ds"
// you can use this method for creating format of your choice
console.log(resultArray); // Output: [1, 11, 30, '1Y 11M 30D']
/* you can access each of Y, M, D separately from output array and can format as per your choice like aY-bM-cD or aYears-bMonths-cDays etc.*/
/*example:
let Y, M, D;
Y = resultArray[0];
M = resultArray[1];
D = resultArray[2];
const customFormat = Y + 'year ' + M + 'months ' + D + 'days';
console.log(customFormat); // output: 1year 11months 30days
*/
console.log(monthsDifference); // Output: 23
// Calculate the difference in months
console.log(weeksDifference); // Output: 164
// Calculate the difference in weeks
console.log(daysDifference); // Output: 1143
// Calculate the difference in days
console.log(yearsDifference); // Output: 1
// Calculate the difference in years
console.log(hoursDifference); // Output: 27432
// Calculate the difference in hours
console.log(minutesDifference); // Output: 1645920
// Calculate the difference in minutes
console.log(secondsDifference); // Output: 98755200
// Calculate the difference in seconds
DatesYMD
Represents a utility class for calculating the formatted and desired customized difference between two dates in all cases.
DatesYMD
:const Formatter = new DatesYMD(firstDate, secondDate);
OR
for version 2.x.x and aboveCan use simplified function diffDates
on module-object
//const DatesYMD = require('@farhan7reza7/diff-ymd-package'); or
const DatesYMD = require('diff-ymd-package');
const Formatter = DatesYMD.diffDates(firstDate, secondDate); // can use any
firstDate
: The first date in the format 'yyyy-mm-dd' or 'yyyy/mm/dd' or 'yyyy.mm.dd' or dateString or dateObject
or Timestamp(epoch).
secondDate
: The second date in the format 'yyyy-mm-dd' or 'yyyy/mm/dd' or 'yyyy.mm.dd' or dateString or dateObject
or Timestamp(epoch).
Special case: empty string("" or '') is by default to current-date(today) for the parameters
Returns:
An instance of DatesYMD class.
diffArray()
Calculates the difference between two dates and returns an array containing Y(years), M(months), D(days), and a formatted 'aY bM cD' difference string.
const result = Formatter.diffArray();
Returns:
An array containing the calculated years, months, days, and the formatted difference.formattedYMD()
Returns the formatted difference between two dates in aY bM cD(aYears bMonths cDays) format.
const result = Formatter.formattedYMD();
Returns:
A string in the format 'aY bM cD'.customizeFormat(yearUnit, monthUnit, dayUnit, partSeparator)
Customizes the difference using specified units and separators
const result = Formatter.customizeFormat(
yearUnit,
monthUnit,
dayUnit,
partSeparator,
);
Returns:
A customized formatted difference string of form (a + yearUnit + partSeparator + b + monthUnit + partSeparator + c + dayUnit), eg. aYs-bMs-cDs etc.diffInMonths()
Calculates the difference in months between two dates.
const monthsDifference = Formatter.diffInMonths();
diffInWeeks()
Calculates the difference in weeks between two dates.
const weeksDifference = Formatter.diffInWeeks();
diffInDays()
Calculates the difference in days between two dates.
const daysDifference = Formatter.diffInDays();
diffInYears()
Calculates the difference in years between two dates.
const yearsDifference = Formatter.diffInYears();
diffInHours()
Calculates the difference in hours between two dates.
const hoursDifference = Formatter.diffInHours();
diffInMinutes()
Calculates the difference in minutes between two dates.
const minutesDifference = Formatter.diffInMinutes();
diffInSeconds()
Calculates the difference in seconds between two dates.
const secondsDifference = Formatter.diffInSeconds();
For more informations, See diff-ymd-package documentation
If you find any issues or have suggestions for improvement, please open an issue or create a pull request on the GitHub repository.
See CONTRIBUTING.md for more informations.
This project is licensed under the MIT License - see the LICENSE file for details.
For more details about what has changed in each version of this project.
See CHANGELOG.md.
FAQs
A javascript library for calculating the difference between two dates in formatted ways like (aY bM cD)(aYears bMonths cDays) or customized desired formats like aY-bM-cD or aYears-bMonths-cDays or kDays or mWeeks or nMonths etc.
The npm package diff-ymd-package receives a total of 14 weekly downloads. As such, diff-ymd-package popularity was classified as not popular.
We found that diff-ymd-package demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.