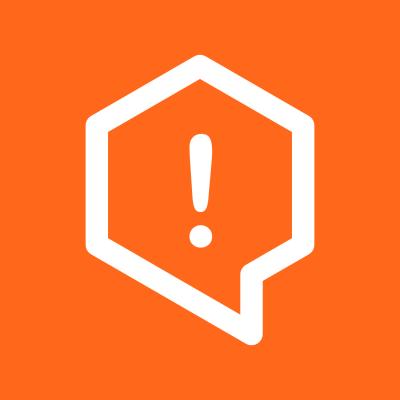
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
directory-import
Advanced tools
Module will allow you to synchronously or asynchronously import (requires) all modules from the folder you specify
Module for automatic import of files from a directory and subdirectories (sync and async). You can use imported modules either from the returned object or in the callback function.
npm install directory-import
After installation, you can use the module in your project:
const { directoryImport } = require('directory-import');
const importedModules = directoryImport('./path/to/directory');
// Will output an object with imported modules
// For example: { modulePath1: module1, modulePath2: module2, ... }
console.log(importedModules);
or:
import { directoryImport } from 'directory-import';
const importedModules = directoryImport('./path/to/directory');
// Will output an object with imported modules
// For example: { modulePath1: module1, modulePath2: module2, ... }
console.log(importedModules);
This is one simple example of how to use the library and how it works under the hood:
const { directoryImport } = require('directory-import');
const importedModules = directoryImport('./sample-directory');
console.info(importedModules);
This can be useful when, for example, you need to do some action depending on the imported file.
const { directoryImport } = require('directory-import');
directoryImport('./sample-directory', (moduleName, modulePath, moduleData) => {
console.info({ moduleName, modulePath, moduleData });
});
Property | Type | Description |
---|---|---|
name | String | Module name based on file name |
path | String | Relative module path |
data | String | Exported data of the module. (Ex: "module.exports = 'test'") |
index | Number | Imported module index |
Property | Type | Description |
---|---|---|
includeSubdirectories | Boolean | If true, the module will import files from subdirectories |
targetDirectoryPath | String | The path to the directory to import modules from |
importPattern | RegExp | RegExp pattern to filter files |
importMode | String | The import mode. Can be 'sync' or 'async' |
limit | Number | Limit the number of imported modules |
Minimum code to use:
const { directoryImport } = require('directory-import');
// Will synchronously import all files in the same directory as the code was called
directoryImport();
Asynchronously import files from the specified directory:
const { directoryImport } = require('directory-import');
const result = directoryImport('./path/to/directory', 'async');
// Promise { <pending> }
console.log(result);
Put result in a variable and invoce callback on each file:
const { directoryImport } = require('directory-import');
const importedModules = directoryImport('./path/to/directory', (moduleName, modulePath, moduleData) => {
// {
// moduleName: 'sample-file-1',
// modulePath: '/sample-file-1.js',
// moduleData: 'This is first sampleFile'
// }
// ...
console.info({ moduleName, modulePath, moduleData });
});
// {
// '/sample-file-1.js': 'This is first sampleFile',
// ...
// }
console.info(importedModules);
const { directoryImport } = require('directory-import');
/**
* Import modules from the current directory synchronously
* @returns {Object} An object containing all imported modules.
*/
const importedModules = directoryImport();
// {
// '/sample-file-1.js': 'This is first sampleFile',
// ...
// }
console.log(importedModules);
const { directoryImport } = require('directory-import');
/**
* Import modules from the current directory synchronously and call the provided callback for each imported module.
* @param {Function} callback - The callback function to call for each imported module.
* @returns {Object} An object containing all imported modules.
*/
directoryImport((moduleName, modulePath, moduleData) => {
// {
// moduleName: 'sample-file-1',
// modulePath: '/sample-file-1.js',
// moduleData: 'This is first sampleFile'
// }
// ...
console.info({ moduleName, modulePath, moduleData });
});
const { directoryImport } = require('directory-import');
/**
* Import modules from the specified directory synchronously
* @param {String} directoryPath - The path to the directory from which you want to import modules.
* @returns {Object} An object containing all imported modules.
*/
const importedModules = directoryImport('./path/to/directory');
// {
// '/sample-file-1.js': 'This is first sampleFile',
// ...
// }
console.log(importedModules);
const { directoryImport } = require('directory-import');
/**
* Import modules from the specified directory synchronously and call the provided callback for each imported module.
* @param {String} directoryPath - The path to the directory from which you want to import modules.
* @param {Function} callback - The callback function to call for each imported module.
* @returns {Object} An object containing all imported modules.
*/
directoryImport('./path/to/directory', (moduleName, modulePath, moduleData) => {
// {
// moduleName: 'sample-file-1',
// modulePath: '/sample-file-1.js',
// moduleData: 'This is first sampleFile'
// }
// ...
console.info({ moduleName, modulePath, moduleData });
});
const { directoryImport } = require('directory-import');
/**
* Import all modules from the specified directory synchronously or asynchronously.
* @param {string} targetDirectoryPath - The path to the directory to import modules from.
* @param {'sync'|'async'} mode - The import mode. Can be 'sync' or 'async'.
* @returns {Object} An object containing all imported modules.
*/
const importModules = directoryImport('./path/to/directory', 'sync');
// {
// '/sample-file-1.js': 'This is first sampleFile',
// ...
// }
console.log(importedModules);
const { directoryImport } = require('directory-import');
/**
* Import all modules from the specified directory synchronously or asynchronously and call the provided callback for each imported module.
* @param {string} targetDirectoryPath - The path to the directory to import modules from.
* @param {'sync'|'async'} mode - The import mode. Can be 'sync' or 'async'.
* @param {Function} callback - The callback function to call for each imported module.
* @returns {Object} An object containing all imported modules.
*/
directoryImport('./path/to/directory', 'sync', (moduleName, modulePath, moduleData) => {
// {
// moduleName: 'sample-file-1',
// modulePath: '/sample-file-1.js',
// moduleData: 'This is first sampleFile'
// }
// ...
console.info({ moduleName, modulePath, moduleData });
});
const { directoryImport } = require('directory-import');
const options = {
includeSubdirectories: true,
targetDirectoryPath: './path/to/directory',
importPattern: /\.js/,
importMode: 'sync',
limit: 2,
};
/**
* Import all modules from the specified directory
* @param {Object} targetDirectoryPath - options - The options object.
* @returns {Object} An object containing all imported modules.
*/
const importModules = directoryImport(options);
// {
// '/sample-file-1.js': 'This is first sampleFile',
// ...
// }
console.log(importedModules);
const { directoryImport } = require('directory-import');
const options = {
includeSubdirectories: true,
targetDirectoryPath: './path/to/directory',
importPattern: /\.js/,
importMode: 'sync',
limit: 2,
};
/**
* Import all modules from the specified directory and call the provided callback for each imported module.
* @param {Object} targetDirectoryPath - options - The options object.
* @param {Function} callback - The callback function to call for each imported module.
* @returns {Object} An object containing all imported modules.
*/
directoryImport(options, (moduleName, modulePath, moduleData) => {
// {
// moduleName: 'sample-file-1',
// modulePath: '/sample-file-1.js',
// moduleData: 'This is first sampleFile'
// }
// ...
console.info({ moduleName, modulePath, moduleData });
});
FAQs
Module will allow you to synchronously or asynchronously import (requires) all modules from the folder you specify
The npm package directory-import receives a total of 27,240 weekly downloads. As such, directory-import popularity was classified as popular.
We found that directory-import demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.