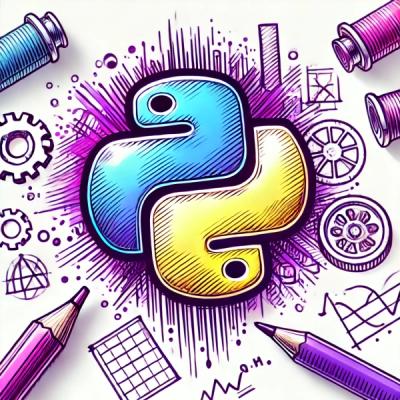
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
discord-fp
Advanced tools
A Beautiful Application Command Framework based on Discord.js
npm install discord-fp
Note
Example below uses commonjs + typescript, you may convert it into normal common js syntax yourself
Stop writing lots of interaction.options.get("name")
just for getting the value of an option
Let us handle everything!
import { options, slash } from "discord-fp";
export default slash({
description: "Say Hello to you",
options: {
name: options.string({
description: "Your name",
}),
},
execute: async ({ event, options }) => {
await event.reply(`Hello, ${options.name}`);
},
});
Tired of finding your command all the place? All commands are file-system based!
Search file by name, you are able to find your command instantly
For slash command: test hello
commands/test/_meta.ts
import { group } from "discord-fp";
export default group({
description: "Your Command Group description",
});
commands/test/hello.ts
import { slash } from "discord-fp";
export default slash({
//...
});
Not just slash commands, you are able to create context menu commands with few lines of code
commands/Delete Message.ts
import { message } from "discord-fp";
export default message({
async execute(e) {
await e.reply("I don't wanna delete message!");
},
});
Wanted to run something before executing a command?
With middleware, you can control how an event handler being fired
commands/_meta.ts
import type { Middleware } from "discord-fp";
export middleware: Middleware = (e, handler) => {
if (something) {
handler(e)
} else {
//...
}
}
From config to options values, It's all type-safe!
export default slash({
description: "Say Hello to you",
options: {
enabled: options.boolean({
description: "Enabled",
required: false,
}),
number: options.number({
description: "Example number",
required: true,
}),
},
//...
});
Take a look at options
:
(parameter) options: {
enabled: boolean | null;
number: number;
}
Start Discord-FP after the bot is ready
import { start } from "discord-fp";
import { join } from "path";
client.on("ready", () => {
start(client, {
//where to load commands
dir: join(__dirname, "commands"),
});
});
Create a file inside the folder
commands/hello.ts
import { slash } from "discord-fp";
export default slash({
description: "Say Hello World",
execute: async ({ event, options }) => {
await event.reply(`Hello World`);
},
});
Start your bot, and run the slash command in Discord
hello
Then you should see the bot replied "Hello World"!
You may use command group & sub command for grouping tons of commands
Create a folder
Create a _meta.ts
/ meta.js
file
Define information for the command group in the _meta
file
import { group } from "discord-fp";
export default group({
description: "My Command group",
});
Create commands inside the folder
Inside the folder, Only slash commands are supported
import { options, slash } from "discord-fp";
export default slash({
description: "Say Hello World",
execute: async ({ event, options }) => {
await event.reply(`Hello World`);
},
});
You may create a _meta
file for controling how the folder being loaded
By defining middleware and loader, you can customize almost everything
For example, Command group is just a type of loader
import { Middleware, group } from "discord-fp";
//loader
export default group({
description: "My Command group"
})
//middleware
export middleware: Middleware = (e, handler) => {
return handler(e)
}
We provides type-safe options out-of-the-box
import { options } from "discord-fp";
options.string({
description: "Your name",
required: false,
});
Enable auto-complete easily
options.string({
description: "Your name",
required: false,
autoComplete(e) {
const items = ["hello", "world"];
const v = e.options.getFocused();
e.respond(result);
},
});
Make your code even better with transform
options.string({
description: "Your name",
require: true,
}).transform((v) => {
return `Mr.${v}`;
}),
//Transform "Henry" -> "Mr.Henry"
ESM has been supported since v0.2.1, notice that we need absolute path for the dir
property
import { start } from "discord-fp";
import { join } from "path";
start(client, {
dir: [join(__dirname, "./commands")],
});
import { start } from "discord-fp";
import { join, dirname } from "path";
import { fileURLToPath } from "url";
start(client, {
//loads ./command folder
dir: ["commands"].map((v) =>
fileURLToPath(join(dirname(import.meta.url), v))
),
});
Feel free to open an issue!
Give this repo a star if you loved this library
FAQs
A Beautiful Application Command Framework based on Discord.js
The npm package discord-fp receives a total of 6 weekly downloads. As such, discord-fp popularity was classified as not popular.
We found that discord-fp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.