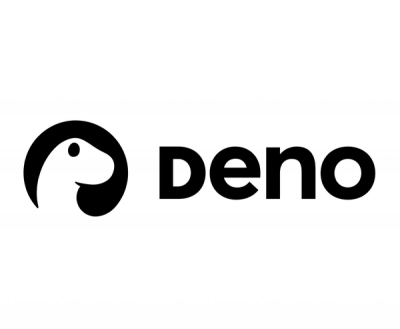
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
discord-modal
Advanced tools
The package helps you to create a modal, which is a new feature of Disocrd, but it is not supported at the moment in djs, so this package will make it easier for you to do it with discord.js v13
You can start install the package on your project:
npm install discord-modal
yarn add discord-modal
pnpm add discord-modal
const DiscordModal = require('discord-modal')
import DiscordModal from 'discord-modal';
DiscordModal(client)
import {DiscordModal,ModalBuilder,ModalField} from 'discord-modal';
const client = new Discord.Client({
intents: ['GUILDS', 'GUILD_MESSAGES']//Set the intentions you want
});
client.on('ready', () => console.log(`Logged in as ${client.user.tag} (${client.user.id})`));
//This action is mandatory in order to be able to connect the bot with the package
DiscordModal(client)
client.on(`interactionCreate`,(interaction)=>{
if(interaction.isCommand()){
if(interaction.commandName == "ping"){
const modal_data = new ModalBuilder()
.setCustomId("submit_a_support_rank")
.setTitle("Submit a support rank")
.addComponents(
new ModalField()
.setLabel("what is your real name?")
.setStyle("short")
.setPlaceholder("Enter your real name here")
.setCustomId("name")
.setRequired(true),//Its default value is false,
new ModalField()
.setLabel("What is your favorite identity?")
.setStyle("short")
.setDefaultValue("JavaScript")
.setMin(10)
.setMax(55)
.setCustomId("favorite"),
new ModalField()
.setLabel("Write a story from your life")
.setStyle("paragraph")
.setCustomId("story")
.setPlaceholder("write here")
)
client.modal.open(interaction, modal_data)
}
}
})
client.on("modalSubmitInteraction",async(interaction)=>{
if(interaction.customId == 'submit_a_support_rank1'){
await interaction.deferReply()
let embed = new Discord.MessageEmbed()
.setColor('GREEN')
.setTitle('Submit a support rank')
.addField('Your real name', '\`\`\`' + interaction.fields.getTextInputValue("name") + '\`\`\`')
.addField('Your favorite identity', '\`\`\`' + interaction.fields.getTextInputValue("favorite") + '\`\`\`')
.addField('Story of your life', '\`\`\`' +interaction.fields.getTextInputValue("story")+ '\`\`\`')
await interaction.editReply({embeds:[embed]})
}
})
client.login("your bot token")
To send the Modal you need to execute an interaction before and the Modal to send. The client is automatically assigned a property which will allow you to send the Modal
Following the previous example where a Modal was created, we would send the Modal as follows:
client.modal.send(interaction, modal_data)
Shuruhatik#2443
Refer to the LICENSE file.
FAQs
The package helps you to create a modal, which is a new feature of Disocrd, but it is not supported at the moment in djs, so this package will make it easier for you to do it with discord.js v13
The npm package discord-modal receives a total of 62 weekly downloads. As such, discord-modal popularity was classified as not popular.
We found that discord-modal demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.