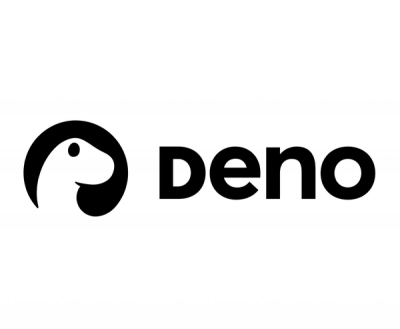
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
discord-player
Advanced tools
Note: this module uses recent discordjs features and requires discord.js version 12.
Discord Player is a powerful Node.js module that allows you to easily implement music commands. Everything is customizable, and everything is done to simplify your work without limiting you!
npm install --save discord-player
Install opusscript or node-opus:
npm install --save opusscript
Install FFMPEG and you're done!
const Discord = require("discord.js"),
client = new Discord.Client(),
settings = {
prefix: "!",
token: "Your Discord Token"
};
const { Player } = require('discord-player');
// Create a new Player (Youtube API key is your Youtube Data v3 key)
const player = new Player(client, "YOUTUBE API KEY");
// To easily access the player
client.player = player;
client.on("ready", () => {
console.log("I'm ready !");
});
client.login(settings.token);
You can pass a third parameter when instantiating the class Player: the options object:
options.leaveOnEnd: whether the bot should leave the voice channel when there is no more song in the queue.
options.leaveOnStop: whether the bot should leave the voice channel when the stop()
function is used.
To play a song, use the client.manager.play()
function.
Usage:
client.player.play(voiceChannel, songName);
Example:
client.on('message', async (message) => {
const args = message.content.slice(settings.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();
// !play Despacito
// will play "Despacito" in the member voice channel
if(command === 'play'){
let song = await client.player.play(message.member.voice.channel, args[0])
message.channel.send(`Currently playing ${song.name}!`);
}
To pause the current song, use the client.manager.pause()
function.
Usage:
client.player.pause(guildID);
Example:
client.on('message', async (message) => {
const args = message.content.slice(settings.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();
if(command === 'pause'){
let song = await client.player.pause(message.guild.id);
message.channel.send(`${song.name} paused!`);
}
});
To resume the current song, use the client.manager.resume()
function.
Usage:
client.player.resume(guildID);
Example:
client.on('message', async (message) => {
const args = message.content.slice(settings.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();
if(command === 'resume'){
let song = await client.player.resume(message.guild.id);
message.channel.send(`${song.name} resumed!`);
}
});
To stop the music, use the client.manager.stop()
function.
Usage:
client.player.stop(guildID);
Example:
client.on('message', (message) => {
const args = message.content.slice(settings.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();
if(command === 'stop'){
client.player.stop(message.guild.id);
message.channel.send('Music stopped!');
}
});
To update the volume, use the client.manager.setVolume()
function.
Usage:
client.player.setVolume(guildID, percent);
Example:
client.on('message', (message) => {
const args = message.content.slice(settings.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();
if(command === 'setvolume'){
client.player.setVolume(message.guild.id, parseInt(args[0]));
message.channel.send(`Volume set to ${args[0]} !`);
}
});
To add a song to the queue, use the client.player.addToQueue()
function.
Usage:
client.player.addToQueue(guildID, songName);
Example:
In this example, you will see how to add a song to the queue if one is already playing.
client.on('message', async (message) => {
const args = message.content.slice(settings.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();
if(command === 'play'){
let aSongIsAlreadyPlaying = client.player.isPlaying(message.guild.id);
// If there's already a song playing
if(aSongIsAlreadyPlaying){
// Add the song to the queue
let song = await client.player.addToQueue(message.guild.id, args[0]);
message.channel.send(`${song.name} added to queue!`);
} else {
// Else, play the song
let song = await client.player.play(message.member.voice.channel, args[0]);
message.channel.send(`Currently playing ${song.name}!`);
}
}
});
To clear the queue, use the client.player.clearQueue()
function.
Usage:
client.player.clearQueue(guildID);
Example:
client.on('message', (message) => {
const args = message.content.slice(settings.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();
if(command === 'clear-queue'){
client.player.clearQueue(message.guild.id);
message.channel.send('Queue cleared!');
}
});
To get the server queue, use the client.player.getQueue()
function.
Usage:
client.player.getQueue(guildID);
Example:
client.on('message', (message) => {
const args = message.content.slice(settings.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();
if(command === 'queue'){
let queue = await client.player.getQueue(message.guild.id);
message.channel.send('Server queue:\n'+(queue.songs.map((song, i) => {
return `${i === 0 ? 'Current' : `#${i+1}`} - ${song.name} | ${song.author}`
}).join('\n')));
}
/**
* Output:
*
* Server queue:
* Current - Despacito | Luis Fonsi
* #2 - Memories | Maroon 5
* #3 - Dance Monkey | Tones And I
* #4 - Circles | Post Malone
*/
});
You can send a message when the queue ends or when the song changes:
client.on('message', (message) => {
const args = message.content.slice(settings.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();
if(command === 'play'){
let song = await client.player.play(message.member.voice.channel, args[0]);
song.queue.on('end', () => {
message.channel.send('The queue is empty, please add new songs!');
});
song.queue.on('songChanged', (oldSong, newSong) => {
message.channel.send(`Now playing ${newSong}...`);
});
}
There are 2 main errors that you can handle like this:
client.on('message', (message) => {
const args = message.content.slice(settings.prefix.length).trim().split(/ +/g);
const command = args.shift().toLowerCase();
// Error 1:
// Song not found
if(command === 'play'){
client.player.play(message.member.voice.channel, args[0]).then((song) => {
message.channel.send(`Currently playing ${song.name}!`);
}).catch(() => {
message.channel.send(`No song found for ${args[0]}`);
});
}
// Error 2:
// Not playing
if(command === 'queue'){
let playing = client.player.isPlaying(message.guild.id);
if(!playing) return message.channel.send(':x: No songs currently playing!');
// you are sure it works:
client.player.getQueue(message.guild.id);
}
});
FAQs
Complete framework to facilitate music commands using discord.js
The npm package discord-player receives a total of 2,606 weekly downloads. As such, discord-player popularity was classified as popular.
We found that discord-player demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.