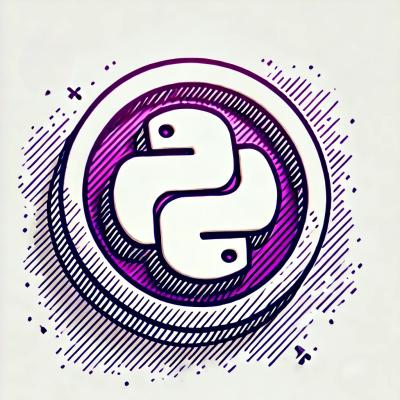
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
discord-slim
Advanced tools
Lightweight Discord client for Node.js.
Provides access to Discord client gateway and API for bots.
Very minimalistic way without excessive abstractions and dependencies. Also with very low resources usage.
New version (V2) is under development.
This version (V1) is now legacy and will be deprecated.
Make sure you have some understaning of Discord API.
Client
- main client class.Methods
Auth(token)
- set up token for the client.Connect(intents?)
- connect to the gateway to receive intents.Disconnect()
- disconnect from the gateway.Request(method, route, data?, auth?)
- send a request to Discord API.WsSend(data)
- send data to the gateway directly.?
means optional arg.
Events
connect
- client established a connection to the gateway.disconnect
- client disconnected.packet
- intent packet received.warn
- other noticeable information.error
- error appeared, client will continue to work.fatal
- fatal error, client will shutdown.Host
- contains current Discord domain.
API
- contains current Discord API address.
CDN
- contains current Discord CDN address.
Routes
- base API route constructors.
Permissions
- list of known permissions.
Intents
- list of known intents.
OPCode
- list of the gateway opcodes.
Other exports from API: AuditLogEvents
, ChannelTypes
, MessageTypes
, MessageActivityTypes
, MessageFlags
, MessageStickerFormatTypes
, DefaultMessageNotificationLevel
, ExplicitContentFilterLevel
, MFA_Level
, VerificationLevel
, PremiumTier
, TargetUserTypes
, ActivityTypes
.
npm i discord-slim
const Discord = require('discord-slim');
const client = new Discord.Client();
client.Auth('BOT_TOKEN');
client.Connect(Discord.Intents.GUILDS | Discord.Intents.GUILD_MESSAGES);
// Listen for intent packets
client.on('packet', packet => {
// packet.t contains intent type
// packet.d contains intent data
if(packet.t == 'MESSAGE_CREATE')
HandleMessage(packet.d);
});
You can read about possible intents here and here.
Auth
and Connect
functions are separated because API requests doesn't actually require connection to the gateway. This is useful if you want only a script that just sends some requests without actual client connection.
const Discord = require('discord-slim');
const client = new Discord.Client();
client.Auth('BOT_TOKEN');
// client.Connect() is not required if you don't need to listen intents
const Routes = Discord.Routes;
// Post a message in some channel
client.Request('POST', Routes.Channel('CHANNEL_ID') + '/messages', { content: 'Hello!' });
Install typescript
package from npm
and run tsc
.
FAQs
Lightweight Discord API library for Node.js.
We found that discord-slim demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.