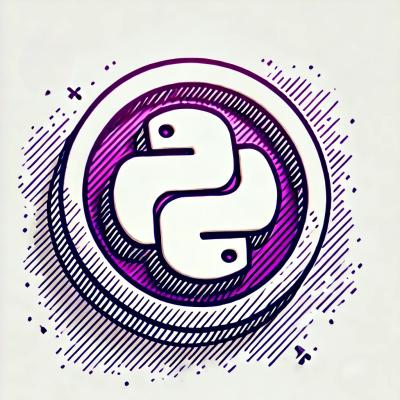
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
discord-slim
Advanced tools
Lightweight Discord client for Node.js.
Provides access to Discord client gateway and API for bots.
Very minimalistic way without excessive abstractions and dependencies. Also with very low resources usage.
Contains breaking changes and incompatible with V1.
Dev version is unstable and can have bugs!
API is unfinished and may be changed with further updates.
Make sure you have some understaning of Discord API.
npm i discord-slim@dev
const { Client, ClientEvents, Authorization, Events, Actions, Helpers } = require('discord-slim');
// Basic setup to control client operation.
// You probably want to use such code for every bot.
const client = new Client();
client.on(ClientEvents.CONNECT, () => console.log('Connection established.'));
client.on(ClientEvents.DISCONNECT, (code) => console.error(`Disconnect. (${code})`));
client.on(ClientEvents.WARN, console.warn);
client.on(ClientEvents.ERROR, console.error);
client.on(ClientEvents.FATAL, (e) => { console.error(e); process.exit(1); });
// Authorization object. Required for client and actions.
const authorization = new Authorization('token');
// Request options for actions
const requestOptions = {
// Include authorization, it is required for most actions.
authorization,
// Rate limit behavior configuration.
// This options is not required, but you probably want to care about the rate limit.
rateLimit: {
// Set how many attempts to make due to the rate limit. Default: 5.
retryCount: 5,
// Rate limit hit callback
callback: (response, attempts) => console.log(`${response.message} Global: ${response.global}. Cooldown: ${response.retry_after} sec. Attempt: ${attempts}.`),
},
};
...
// Start the client connection.
client.Connect(authorization, Helpers.Intents.GUILDS | Helpers.Intents.GUILD_MESSAGES);
You can read about intents here.
client.events.on(Events.MESSAGE_CREATE, (message) => {
if(message.author.id == client.user.id) return;
// Check that the message contains phrases like "hello bot" or "hi bot"
if(message.content.search(/(^|\s)h(ello|i)(\s|\s.*\s)bot($|\s)/i) < 0) return;
Actions.Message.Create(message.channel_id, {
content: `Hi, <@${message.author.id}>!`,
message_reference: {
channel_id: message.channel_id,
message_id: message.id,
},
}, requestOptions);
});
client.events.on(Events.READY, () => {
client.UpdateStatus({
since: 0,
activities: [
{
name: 'YOU',
type: Helpers.ActivityTypes.WATCHING,
}
],
afk: false,
status: Helpers.StatusTypes.ONLINE,
});
});
Note: slash commands requires applications.commands
scope. Read details in docs.
// Create a command in your guild(s).
client.events.on(Events.GUILD_CREATE, (guild) => {
Actions.Application.CreateGuildCommand(client.user.id, guild.id, {
name: 'echo',
description: 'Test slash command.',
options: [
{
type: Helpers.ApplicationCommandOptionTypes.STRING,
name: 'text',
description: 'Echo message text.',
required: true,
},
],
}, requestOptions);
});
// Respond to interaction event.
client.events.on(Events.INTERACTION_CREATE, (interaction) => {
if(!(interaction.data && interaction.data.name == 'echo')) return;
Actions.Application.CreateInteractionResponse(interaction.id, interaction.token, {
type: Helpers.InteractionResponseTypes.CHANNEL_MESSAGE_WITH_SOURCE,
data: {
content: interaction.data.options[0].value,
flags: Helpers.InteractionResponseFlags.EPHEMERAL,
},
}, requestOptions);
});
Install typescript
package from npm
and run tsc
.
FAQs
Lightweight Discord API library for Node.js.
The npm package discord-slim receives a total of 0 weekly downloads. As such, discord-slim popularity was classified as not popular.
We found that discord-slim demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.