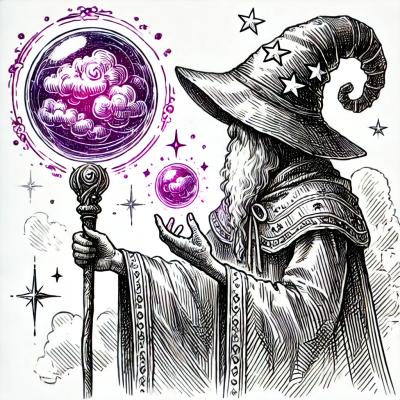
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
This module is an extension of discord.js, so the internal behavior (methods, properties, ...) is the same.
This library allows you to use TypeScript decorators on discord.js, it simplifies your code and improves the readability!
Version 16.6.0 or newer of Node.js is required
npm install discordx
yarn add discordx
discordx-templates (starter repo)
Shana from @VictoriqueMoe
With discordx
, we intend to provide the latest up-to-date package to easily build feature-rich bots with multi-bot compatibility, simple commands, pagination, music, and much more. Updated daily with discord.js changes.
Try discordx now with CodeSandbox
If you have any issues or feature requests, Please open an issue at GitHub or join discord server
@Bot
)@SimpleCommand
to use old fashioned command, such as !hello world
@SimpleCommandOption
parse and define command options like @SlashOption
client.initApplicationCommands
to create/update/remove discord application commandsHere are more packages from us to extend the functionality of your Discord bot.
Package | Description |
---|---|
create-discordx | Create discordx apps with one command |
discordx | Create a discord bot with TypeScript and Decorators! |
@discordx/changelog | Changelog generator, written in TypeScript with Node.js |
@discordx/di | Dependency injection service with TSyringe support |
@discordx/importer | Import solution for ESM and CJS |
@discordx/internal | discordx internal methods, can be used for external projects |
@discordx/koa | Create rest api server with Typescript and Decorators |
@discordx/lava-player | Create lavalink player |
@discordx/lava-queue | Create queue system for lavalink player |
@discordx/music | Create discord music player easily |
@discordx/pagination | Add pagination to your discord bot |
@discordx/socket.io | Create socket.io server with Typescript and Decorators |
@discordx/utilities | Create own group with @Category and guards |
discord-spams | Tiny but powerful discord spam protection library |
There is a whole system that allows you to implement complex slash/simple commands and handle interactions like button, select-menu, context-menu etc.
Discord has it's own command system now, you can simply declare commands and use Slash commands this way
@Discord()
class Example {
@Slash({ name: "hello" })
hello(
@SlashOption({ name: "message" }) message: string,
interaction: CommandInteraction
): void {
interaction.reply(`:wave: from ${interaction.user}: ${message}`);
}
}
Create discord button handler with ease!
@Discord()
class Example {
@ButtonComponent({ id: "hello" })
handler(interaction: ButtonInteraction): void {
interaction.reply(":wave:");
}
@ButtonComponent({ id: "hello" })
handler2(interaction: ButtonInteraction): void {
console.log(`${interaction.user} says hello`);
}
@Slash()
test(interaction: CommandInteraction): void {
const btn = new ButtonBuilder()
.setLabel("Hello")
.setStyle(ButtonStyle.Primary)
.setCustomId("hello");
const buttonRow =
new ActionRowBuilder<MessageActionRowComponentBuilder>().addComponents(
btn
);
interaction.reply({
components: [buttonRow],
});
}
}
Create discord select menu handler with ease!
const roles = [
{ label: "Principal", value: "principal" },
{ label: "Teacher", value: "teacher" },
{ label: "Student", value: "student" },
];
@Discord()
class Example {
@SelectMenuComponent({ id: "role-menu" })
async handle(interaction: SelectMenuInteraction): Promise<unknown> {
await interaction.deferReply();
// extract selected value by member
const roleValue = interaction.values?.[0];
// if value not found
if (!roleValue) {
return interaction.followUp("invalid role id, select again");
}
interaction.followUp(
`you have selected role: ${
roles.find((r) => r.value === roleValue)?.label ?? "unknown"
}`
);
return;
}
@Slash({ description: "roles menu", name: "my-roles" })
async myRoles(interaction: CommandInteraction): Promise<unknown> {
await interaction.deferReply();
// create menu for roles
const menu = new SelectMenuBuilder()
.addOptions(roles)
.setCustomId("role-menu");
// create a row for message actions
const buttonRow =
new ActionRowBuilder<MessageActionRowComponentBuilder>().addComponents(
menu
);
// send it
interaction.editReply({
components: [buttonRow],
content: "select your role!",
});
return;
}
}
Create discord context menu options with ease!
@Discord()
class Example {
@ContextMenu({
name: "Hello from discordx",
type: ApplicationCommandType.Message,
})
messageHandler(interaction: MessageContextMenuCommandInteraction): void {
console.log("I am message");
interaction.reply("message interaction works");
}
@ContextMenu({
name: "Hello from discordx",
type: ApplicationCommandType.User,
})
userHandler(interaction: UserContextMenuCommandInteraction): void {
console.log(`Selected user: ${interaction.targetId}`);
interaction.reply("user interaction works");
}
}
Create discord modal with ease!
@Discord()
class Example {
@Slash()
modal(interaction: CommandInteraction): void {
// Create the modal
const modal = new ModalBuilder()
.setTitle("My Awesome Form")
.setCustomId("AwesomeForm");
// Create text input fields
const tvShowInputComponent = new TextInputBuilder()
.setCustomId("tvField")
.setLabel("Favorite TV show")
.setStyle(TextInputStyle.Short);
const haikuInputComponent = new TextInputBuilder()
.setCustomId("haikuField")
.setLabel("Write down your favorite haiku")
.setStyle(TextInputStyle.Paragraph);
const row1 = new ActionRowBuilder<TextInputBuilder>().addComponents(
tvShowInputComponent
);
const row2 = new ActionRowBuilder<TextInputBuilder>().addComponents(
haikuInputComponent
);
// Add action rows to form
modal.addComponents(row1, row2);
// --- snip ---
// Present the modal to the user
interaction.showModal(modal);
}
@ModalComponent()
async AwesomeForm(interaction: ModalSubmitInteraction): Promise<void> {
const [favTVShow, favHaiku] = ["tvField", "haikuField"].map((id) =>
interaction.fields.getTextInputValue(id)
);
await interaction.reply(
`Favorite TV Show: ${favTVShow}, Favorite haiku: ${favHaiku}`
);
return;
}
}
Create a simple command handler for messages using @SimpleCommand
. Example !hello world
@Discord()
class Example {
@SimpleCommand({ aliases: ["hey", "hi"], name: "hello" })
hello(command: SimpleCommandMessage): void {
command.message.reply(":wave:");
}
}
We can declare methods that will be executed whenever a Discord event is triggered.
Our methods must be decorated with the @On(event: string)
or @Once(event: string)
decorator.
That's simple, when the event is triggered, the method is called:
@Discord()
class Example {
@On({ name: "messageCreate" })
messageCreate() {
// ...
}
@Once({ name: "messageDelete" })
messageDelete() {
// ...
}
}
Create a reaction handler for messages using @Reaction
.
@Discord()
class Example {
@Reaction({ emoji: "โญ", remove: true })
async starReaction(reaction: MessageReaction, user: User): Promise<void> {
await reaction.message.reply(`Received a ${reaction.emoji} from ${user}`);
}
@Reaction({ aliases: ["๐", "custom_emoji"], emoji: "๐" })
async pin(reaction: MessageReaction): Promise<void> {
await reaction.message.pin();
}
}
We implemented a guard system that functions like the Koa middleware system
You can use functions that are executed before your event to determine if it's executed. For example, if you want to apply a prefix to the messages, you can simply use the @Guard
decorator.
The order of execution of the guards is done according to their position in the list, so they will be executed in order (from top to bottom).
Guards can be set for @Slash
, @On
, @Once
, @Discord
and globally.
import { Discord, On, Client, Guard } from "discordx";
import { NotBot } from "./NotBot";
import { Prefix } from "./Prefix";
@Discord()
class Example {
@On()
@Guard(
NotBot // You can use multiple guard functions, they are executed in the same order!
)
messageCreate([message]: ArgsOf<"messageCreate">) {
switch (message.content.toLowerCase()) {
case "hello":
message.reply("Hello!");
break;
default:
message.reply("Command not found");
break;
}
}
}
FAQs
Create a discord bot with TypeScript and Decorators!
The npm package discordx receives a total of 773 weekly downloads. As such, discordx popularity was classified as not popular.
We found that discordx demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISAโs 2024 report highlights the EUโs top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.