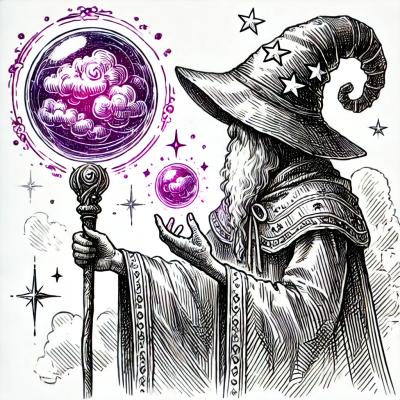
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
discord.ts (@typeit/discord)
Create your discord bot by using TypeScript and decorators!
This module is an extension of discord.js, so the internal behavior (methods, properties, ...) is the same.
This library allows you to use TypeScript decorators on discord.js, it simplify your code and improve the readability !
https://owencalvin.github.io/discord.ts/
Discord has it's own command system now, you can simply declare commands and use Slash commands this way
import { Discord, Slash } from "@typeit/discord";
import { CommandInteraction } from "discord.js";
@Discord()
abstract class AppDiscord {
@Slash("hello")
private hello(
@Option("text")
text: string,
interaction: CommandInteraction
) {
// ...
}
}
There is a whole system that allows you to implement complex Slash commands
@Choice
@Choices
@Option
@Permission
@Guild
@Group
@Description
@Guard
We can declare methods that will be executed whenever a Discord event is triggered.
Our methods must be decorated with the @On(event: string)
or @Once(event: string)
decorator.
That's simple, when the event is triggered, the method is called:
import { Discord, On, Once } from "@typeit/discord";
@Discord()
abstract class AppDiscord {
@On("messageCreate")
private onMessage() {
// ...
}
@Once("messageDelete")
private onMessageDelete() {
// ...
}
}
We implemented a guard system thats work pretty like the Koa middleware system
You can use functions that are executed before your event to determine if it's executed. For example, if you want to apply a prefix to the messages, you can simply use the @Guard
decorator.
The order of execution of the guards is done according to their position in the list, so they will be executed in order (from top to bottom).
Guards can be set for @Slash
, @On
, @Once
, @Discord
and globaly.
import { Discord, On, Client, Guard } from "@typeit/discord";
import { NotBot } from "./NotBot";
import { Prefix } from "./Prefix";
@Discord()
abstract class AppDiscord {
@On("messageCreate")
@Guard(
NotBot, // You can use multiple guard functions, they are excuted in the same order!
Prefix("!")
)
async onMessage([message]: ArgsOf<"messageCreate">) {
switch (message.content.toLowerCase()) {
case "hello":
message.reply("Hello!");
break;
default:
message.reply("Command not found");
break;
}
}
}
Use npm or yarn to install @typeit/discord@slash with discord.js
Please refer to the documentation
Simply join the Discord server
You can also find help with the different projects that use discord.ts and in the examples folder
FAQs
Create a discord bot with TypeScript and Decorators!
The npm package discordx receives a total of 773 weekly downloads. As such, discordx popularity was classified as not popular.
We found that discordx demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.