Disgame
Disgame is an extremely lightweight Discord game engine. (Beta)
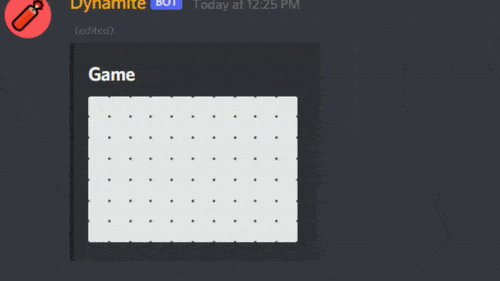
const wait = (ms: number) => new Promise((resolve, reject) => setTimeout(resolve, ms));
client.on("message", async (msg) => {
const gameMessage = await msg.channel.send(
new Discord.MessageEmbed()
.setTitle("Game")
.setDescription("...")
.setFooter("")
)
const game = new Disgame({
message: gameMessage,
size: {
width: 10,
height: 7
},
backgroundEmoji: "⬜"
});
const tile1 = game.addTile(new Tile({ emoji: "🍎", position: { x: 0, y: 0 } }));
const tile2 = game.addTile(new Tile({ emoji: "🍌", position: { x: 3, y: 3 } }));
const tile3 = game.addTile(new Tile({ emoji: "🍐", position: { x: 5, y: 5 } }));
for (var i = 0; i < 4; i++) {
tile1.position.x++;
tile1.position.y++;
game.render();
await wait(1000);
}
game.removeTile(tile1);
game.render();
});
Table of Contents
- Overview
- Usage
- Base
- Tiles
- Examples
- Credits
Usage
Base
The base (Disgame) is the start to any game. It is used to render the view to a chosen message
const game = new Disgame({
message: gameMessage,
size: {
width: 10,
height: 7
},
backgroundEmoji: "⬜"
});
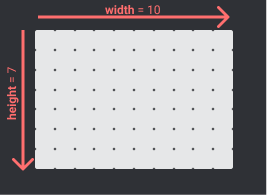
Options/Properties
Optional | Name | Description | Default |
---|
❌ | message | Discord.Message The message in which you want to render the game when render() is called. | |
✅ | size | {width: number; height: number} The size of the canvas. | {width: 10, height: 10} |
✅ | backgroundEmoji | `string The emoji used to fill the background. | ⬛ |
Methods
Name | Description | Returns |
---|
render() | "Renders" the game view as text to the Discord message. (Should be called when you want to update a change on the screen) | void |
addTile(tile: Tile) | Adds a new tile object to the game. | tile |
removeTile(tile: Tile) | Removes a tile from the game. | void |
Tiles
Tiles are emojis at certain positions on the game canvas. The can be added and remove from games using the respective game functions addTile(tile: Tile)
/removeTile(tile: Tile)
.
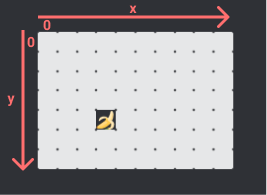
const game = new Disgame({
message: gameMessage,
size: {
width: 10,
height: 7
},
backgroundEmoji: "⬜"
});
let bananaTile = game.addTile(new Tile({
emoji: "🍌",
position: {
x: 3,
y: 4
}
}));
game.render();
Options/Properties
Optional | Name | Description | Default |
---|
❌ | emoji | string The emoji used to display the tile | |
✅ | position | (IPosition) {x: number, y: number} The tile's position | {x: 0, y: 0} |
Examples
Moving Tile
Coming soon
Credits
Made by Badusername420