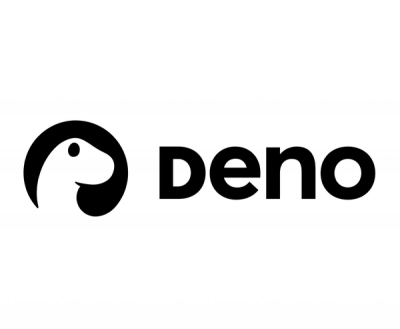
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
divshot-api
Advanced tools
Wrapper for the Divshot API.
NPM
npm install divshot-api --save
Bower
bower install divshot --save
Refer to the Narrator api for a more in depth understanding of all available methods.
CommonJS (Node/Browserify)
var Divshot = require('divshot');
Standalone
<script src="/path/to/divshot.standalone.min.js"></div>
###Instantiate
var api = Divshot.createClient({
client_id: '123abc', // MUST be specified
email: 'someone@divshot.com',
password: 'somepassword123!',
// OR
token: 'your divshot access token'
});
###Browser Authentication
The Divshot API wrapper provides a simple, popup-based authentication mechanism for
easily authenticating a user without requiring the handling of usernames or passwords.
To use browser authentication, simply instantiate a client and call the auth
method:
divshot.auth(type, options, callback)
This method returns a promise but takes an optional callback. type
should be either
password
or github
(defaults to password
).
var api = Divshot.createClient({client_id: 'abc123'});
divshot.auth().then(function(response) {
response.user; // user details
response.access_token; //access token
}, function (error) {
// do something with an error
});
If you need to accept an email and password directly, you can do so as in the following example. Passwords MUST NOT be stored and should only come from direct user input (e.g. a form field).
divshot.auth('password', {email: 'abby@example.com', password: 'test123'}).then(function(response) {
// works just as above
}, function (error) {
// ditto
});
For convenience, the auth
method allows you to store a cookie with an encoded access token
to keep the user logged into Divshot. Simply pass the store: true
option to auth
:
api.auth({store: true}, function(error, token, user){
// ...
});
This will automatically create a cookie on the current domain to store the access token for one
week. On subsequent page loads you can use the authWithCookie()
method to authenticate a client
based on the cookie. This method will return true
if a cookie was found and false
otherwise.
if (api.authWithCookie()) {
// cookie found, api is now authenticated and can
// make calls to retrieve protected resources
} else {
// no cookie found, display an auth button etc
}
###Angular Module
Located at /dist/divshot.angular.js
angular.module('myApp', ['divshot'])
.config(function (divshotProvider) {
divshotProvider.configure({
token: 'divshot_api_access_token'
});
}).
controller('SomeCtrl', function ($scope, divshot) {
$scope.apps = divshot.apps.list();
});
Custom XHR arguments can be set to be sent with each request. Refer to Angular's $http documentation for which arguments are supported.
angular.module('myApp', ['divshot'])
.config(function (divshotProvider) {
divshotProvider.configure({
token: 'divshot_api_access_token'
});
}).
controller('SomeCtrl', function ($scope, divshot) {
divshot.withCredentials(true);
// or
divshot.xhr('withCredentials', true);
});
###User
By default, the authenticate
method will be called on each request as a pre hook. If a token is provided, this does not create another http request.
api.user.authenticate(function (err, token) {
});
api.user.setCredentials({
email: 'someone@divshot.com',
password: 'somepassword123!',
// OR
token: 'some_really_long_access_token_from_divshot'
});
// User data
api.user.self().then(function (user) {
});
// OR
api.user.id(userId).get().then(function (user) {
});
// Update user
var user = api.user.id(userId);
user.update({
name: 'First Last'
}).then(function (user) {
});
// Set welcomed for new users
api.user.setWelcomed().then(function () {
});
// Delete account
api.user.deleteAcccount(user@email.com).then(function () {
});
// Change password
divshot.user.password.update({
password: 'Password123',
password_confirm: 'Password123'
}).then(function (res) {
});
// Reset password if forgotten
divshot.password.reset(userId).then(function (res) {
});
// Add email
divshot.user.emails.add('something@aol.com').then(function (res) {
});
// Set primary email
divshot.user.emails.primary('something@aol.com').then(function (res) {
});
// Remove email
divshot.user.emails.remove('something@aol.com').then(function (res) {
});
// Resend email
divshot.user.emails.resend('something@aol.com').then(function (res) {
});
// Users orgs
divshot.organizations.list().then(function (orgs) {
});
// A single organization
divshot.organizations.id(someOrgId).get().then(function (org) {
});
// Apps from an organization
divshot.organizations.id(someOrgId).apps.list().then(function (apps) {
});
// Create organization
divshot.organizations.create({
name: 'name',
nick: 'nick',
billing_email: 'someone@aol.com',
gravatar_email: 'someone@aol.com'
}).then(function (res) {
});
// Update organization
divshot.organizations.id(someOrgId).update({
name: 'name',
billing_email: 'someone@aol.com',
gravatar_email: 'someone@aol.com',
etc: 'other stuff'
}).then(function (res) {
});
// Get org members
divshot.organizations.id(someOrg).members.list().then(function (members) {
});
// Invite members to organization
divshot.organizations.id(someOrg).members.create({
name: email,
email: email
}).then(function (res) {
});
// Update a member in organiztion
divshot.organizations.id(someOrg).members.id(memberid).update({
admin: false // or true
}).then(function (res) {
});
// Remove a member from an organization
divshot.organizations.id(someOrg).members.id(memberId).remove().then(function () {
});
// List apps
api.apps.list().then(function (apps) {
});
// Create an app
api.apps.create('app-name').then(function (app) {
});
// Create an app from object
api.apps.createFromObject(params).then(function (app) {
});
// A specific app
var app = api.apps.id('app name');
app.get().then(function (app) {
});
// Delete an app
app.remove().then(function (res) {
});
app.builds.list(function (err, builds) {
});
app.builds.id('build id').get(function (err, build) {
// Specific build by id
});
app.builds.id('build id').finalize(function (err, response) {
});
app.builds.id('build id').release('production', function (err, response) {
});
###Releases
app.releases.list(function (err, releases) {
});
app.releases.env('production').get(function (err, release) {
// Release by environment
});
app.releases.env('production').rollback(function (err, response) {
});
// Rollback to specific version
app.releases.env('production').rollbackTo('v12').then(function () {
});
app.releases.env('production').promote('staging', function (err, callback) {
// Here, "staging" is the from environment
// and "production" is the to-environment
});
###Domains
// List domains for an app
app.domains.list().then(function(domains) {
});
// Add a domain
app.domains.add('www.domain.com').then(function (response) {
});
// Remove a domain
app.domains.remove('www.domain.com').then(function (response) {
});
Update data associated with an App's subscription
app.subscription.update('card number').then(function (res) {
});
Manipulate webhooks associated with your app
app.webhooks.list().then(function (response) {
});
app.webhooks.create({url: 'some-url.com', active: true}).then(function () {
});
app.webhooks.resume(hook).then(function () {
});
app.webhooks.pause(hook).then(function () {
});
app.webhooks.remove(hookId).then(function () {
});
###App Environment Configuration
app.env('development').config({
auth: 'username:password'
}, function (err, response) {
});
grunt build
FAQs
Wrapper for the Divshot api
We found that divshot-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.