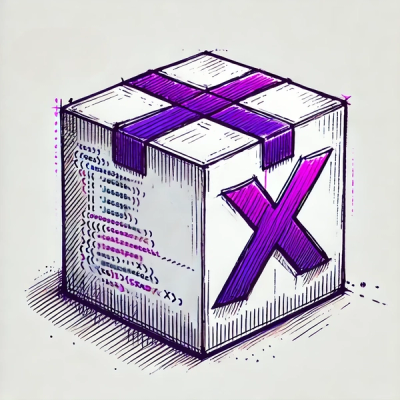
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
The dlv npm package is a safe deep property access utility. It allows you to access deeply nested properties within an object without the risk of throwing an error if a part of the path does not exist. It's particularly useful when dealing with complex data structures where some properties may be undefined or null.
Safe property access
Access a deeply nested property without throwing if the path does not exist.
const dlv = require('dlv');
const obj = { a: { b: { c: 1 } } };
const value = dlv(obj, 'a.b.c'); // 1
Default value
Provide a default value if the deep property is undefined.
const dlv = require('dlv');
const obj = { a: { b: { } } };
const value = dlv(obj, 'a.b.c', 'default'); // 'default'
Access array indexes
Access an array index within a deeply nested structure.
const dlv = require('dlv');
const obj = { a: { b: [1, 2, 3] } };
const value = dlv(obj, ['a', 'b', 0]); // 1
Lodash's get function is similar to dlv in that it allows for safe access to nested object properties. It is part of the larger Lodash library which offers a wide range of utilities for working with arrays, numbers, objects, strings, etc. Compared to dlv, lodash.get is part of a larger utility library, which might be preferable for some projects that can make use of other Lodash functions.
The idx library provides a utility to safely access deeply nested properties in JavaScript. It uses a callback pattern and is built with TypeScript support. idx is similar to dlv but uses a different syntax and can be more verbose. It also supports TypeScript out of the box, which can be a deciding factor for projects using TypeScript.
dlv(obj, keypath)
Safely get a dot-notated path within a nested object, with ability to return a default if the full key path does not exist or the value is undefined
Smallest possible implementation: only 130 bytes.
You could write this yourself, but then you'd have to write tests.
Supports ES Modules, CommonJS and globals.
npm install --save dlv
delve(object, keypath, [default])
import delve from 'dlv';
let obj = {
a: {
b: {
c: 1,
d: undefined,
e: null
}
}
};
//use string dot notation for keys
delve(obj, 'a.b.c') === 1;
//or use an array key
delve(obj, ['a', 'b', 'c']) === 1;
delve(obj, 'a.b') === obj.a.b;
//returns undefined if the full key path does not exist and no default is specified
delve(obj, 'a.b.f') === undefined;
//optional third parameter for default if the full key in path is missing
delve(obj, 'a.b.f', 'foo') === 'foo';
//or if the key exists but the value is undefined
delve(obj, 'a.b.d', 'foo') === 'foo';
//Non-truthy defined values are still returned if they exist at the full keypath
delve(obj, 'a.b.e', 'foo') === null;
//undefined obj or key returns undefined, unless a default is supplied
delve(undefined, 'a.b.c') === undefined;
delve(undefined, 'a.b.c', 'foo') === 'foo';
delve(obj, undefined, 'foo') === 'foo';
dlv
and very fast.dlv
and is implemented in a very similar manner.FAQs
Safely get a dot-notated property within an object.
The npm package dlv receives a total of 8,065,130 weekly downloads. As such, dlv popularity was classified as popular.
We found that dlv demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.