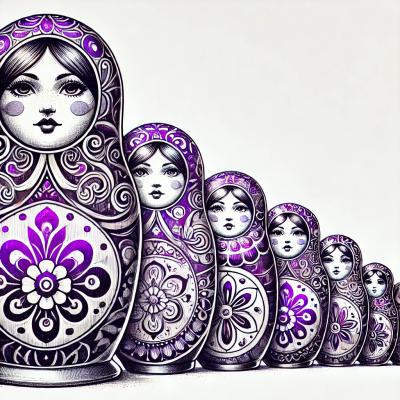
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
dmd (document with markdown) is a collection of handlebars templates for generating markdown documentation from jsdoc-parse input data. It is the default template set used by jsdoc-to-markdown.
The dmd (documentation markdown) npm package is a tool for generating markdown documentation from JSDoc comments in your JavaScript code. It allows you to create well-structured and readable documentation for your projects.
Generate Markdown Documentation
This feature allows you to generate markdown documentation from JSDoc comments in your JavaScript files. The code sample demonstrates how to use dmd in conjunction with jsdoc-to-markdown to read JSDoc data from a file and convert it into markdown format.
const dmd = require('dmd');
const jsdoc2md = require('jsdoc-to-markdown');
const jsdocData = jsdoc2md.getTemplateDataSync({ files: 'your-file.js' });
const output = dmd(jsdocData);
console.log(output);
Custom Templates
This feature allows you to use custom templates to format the generated markdown documentation. The code sample shows how to define a custom template and use it with dmd to generate documentation.
const dmd = require('dmd');
const jsdoc2md = require('jsdoc-to-markdown');
const jsdocData = jsdoc2md.getTemplateDataSync({ files: 'your-file.js' });
const template = '{{#module}}{{name}}{{/module}}';
const output = dmd(jsdocData, { template: template });
console.log(output);
Helper Functions
This feature allows you to define and use custom helper functions in your templates. The code sample demonstrates how to create a helper function that converts text to uppercase and use it in the generated documentation.
const dmd = require('dmd');
const jsdoc2md = require('jsdoc-to-markdown');
const jsdocData = jsdoc2md.getTemplateDataSync({ files: 'your-file.js' });
const helpers = {
uppercase: function (str) { return str.toUpperCase(); }
};
const output = dmd(jsdocData, { helper: helpers });
console.log(output);
jsdoc-to-markdown is a tool that generates markdown documentation from JSDoc comments. It is often used in conjunction with dmd to provide the data that dmd formats into markdown. Unlike dmd, jsdoc-to-markdown focuses on extracting and converting JSDoc comments into a format that can be further processed.
documentation is a documentation generation tool that supports multiple output formats, including HTML and markdown. It provides a more comprehensive solution compared to dmd, with built-in support for various output formats and more advanced features like linting and validation of JSDoc comments.
docco is a quick-and-dirty documentation generator that produces HTML documentation with source code and comments side-by-side. While it does not generate markdown like dmd, it is useful for creating readable documentation that closely follows the source code.
dmd (document with markdown) is a module containing handlebars partials and helpers intended to transform jsdoc-parse output into markdown API documentation. It exposes dmd
, a function which requires data and a template. See jsdoc-to-markdown for example output.
With this input file containing jsdoc-parse output:
[
{
"id": "fatUse",
"name": "fatUse",
"kind": "member",
"description": "I am a global variable",
"scope": "global"
}
]
this command:
$ cat examples/input/doclet.json | dmd
produces this markdown output:
<a name="fatUse"></a>
## fatUse
I am a global variable
**Kind**: global variable
Install:
$ npm install dmd --save
Example:
var dmd = require("dmd");
var options = {
template: "my-template.hbs"
};
process.stdin.pipe(dmd(options)).pipe(process.stdout);
Install the dmd
tool globally:
$ npm install -g dmd
Example:
$ cat examples/doclet.json | dmd
$ dmd --help
The default template contains a single call to the main partial:
{{>main}}
This partial outputs all documentation and an index (if there are enough items). You can customise the output by supplying your own template. For example, you could write a template like this:
# A Module
This is the readme for a module.
## Install
Install it using the power of thought. While body-popping.
# API Documentation
{{>main}}
and employ it like this:
$ cat your-docs.json | dmd --template readme-template.hbs
You can customise the generated documentation to taste by overriding or adding partials and/or helpers.
For example, let's say you wanted this datestamp at the bottom of your generated docs:
**documentation generated on Sun, 01 Mar 2015 09:30:17 GMT**
You need to do two things:
A helper file is just a plain commonJS module. Each method exposed on the module will be available as a helper in your templates. So, our new helper module:
exports.generatedDate = function(){
return new Date().toUTCString();
}
Read more about helpers in the handlebars documentation.
Create a duplicate of the main partial (typically in the project you are documenting) containing your new footer:
{{>main-index~}}
{{>all-docs~}}
**documentation generated on {{generatedDate}}**
the file basename of a partial is significant - if you wish to override main
(invoked by {{>main}}
) then the filename of your partial must be main.hbs
.
To use the overrides, pass their file names as options to dmd (or jsdoc-to-markdown if you're using that):
$ cat your-parsed-docs.json | dmd --partial custom/main.hbs --helper custom/generatedDate.js
If you have multiple overrides, the syntax is
$ cat your-parsed-docs.json | dmd --partial override1.hbs override2.hbs
Globbing also works:
$ cat your-parsed-docs.json | dmd --partial overrides/*.hbs
If you wish to version-control and/or share your customisations you can create a plugin for distribution via npm. See dmd-plugin-example as an example and boilerplate to get you started.
Once you have your plugin, install it where required as a dev-dependency. Then supply the plugin package name(s) to the --plugin
option, for example:
$ cd my-project
$ npm install dmd-plugin-example --save-dev
$ jsdoc2md lib/my-module.js --plugin dmd-plugin-example
Transform
⏏Transforms doclet data into markdown documentation. Returns a transform stream - pipe doclet data in to receive rendered markdown out.
Kind: Exported function
Params
DmdOptions
- The render optionsAll dmd options and their defaults
Kind: inner class of dmd
string
number
string
array
array
array
string
boolean
boolean
string
string
string
string
string
array
string
The template the supplied documentation will be rendered into. Use the default or supply your own template for full control over the output.
Kind: instance property of DmdOptions
Default: "{{>main}}"
Example
var fs = require("fs")
var dmd = require("../")
var template = "The description from my class: {{#class name='MyClass'}}{{description}}{{/class}}"
fs.createReadStream(__dirname + "/my-class.json")
.pipe(dmd({ template: template }))
.pipe(process.stdout)
outputs:
The description from my class: MyClass is full of wonder
the equivation operation using the command-line tool:
$ dmd --template template.hbs --src my-class.json
number
The initial heading depth. For example, with a value of 2
the top-level markdown headings look like "## The heading"
.
Kind: instance property of DmdOptions
Default: 2
string
Specifies the default language used in @example blocks (for syntax-highlighting purposes). In gfm mode, each @example is wrapped in a fenced-code block. Example usage: --example-lang js
. Use the special value none
for no specific language. While using this option, you can override the supplied language for any @example by specifying the @lang
subtag, e.g @example @lang hbs
. Specifying @example @lang off
will disable code blocks for that example.
Kind: instance property of DmdOptions
Default: "js"
array
Use an installed package containing helper and/or partial overrides
Kind: instance property of DmdOptions
array
handlebars helper files to override or extend the default set
Kind: instance property of DmdOptions
array
handlebars partial files to override or extend the default set
Kind: instance property of DmdOptions
string
Format identifier names in the code style, (i.e. format using backticks or <code></code>
)
Kind: instance property of DmdOptions
boolean
By default, dmd generates github-flavoured markdown. Not all markdown parsers render gfm correctly. If your generated docs look incorrect on sites other than Github (e.g. npmjs.org) try enabling this option to disable Github-specific syntax.
Kind: instance property of DmdOptions
boolean
Put <hr>
breaks between identifiers. Improves readability on bulky docs.
Kind: instance property of DmdOptions
Default: false
string
none, grouped, table, dl
Kind: instance property of DmdOptions
Default: "dl"
string
none, grouped, table, dl
Kind: instance property of DmdOptions
Default: "dl"
string
Two options to render parameter lists: 'list' or 'table' (default). Table format works well in most cases but switch to list if things begin to look crowded / squashed.
Kind: instance property of DmdOptions
Default: "table"
string
list, table
Kind: instance property of DmdOptions
Default: "table"
string
grouped, list
Kind: instance property of DmdOptions
Default: "grouped"
array
a list of fields to group member indexes by
Kind: instance property of DmdOptions
Default: ["scope","category"]
© 2014-2016 Lloyd Brookes <75pound@gmail.com>. Documented by jsdoc-to-markdown.
FAQs
The default output template for jsdoc-to-markdown
The npm package dmd receives a total of 172,059 weekly downloads. As such, dmd popularity was classified as popular.
We found that dmd demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.