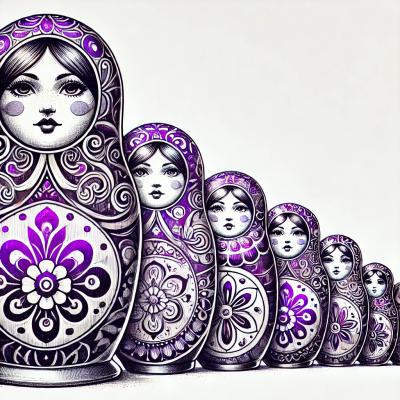
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
👬 A Friendly wrapper for your favourite DOM Apis ✨ in **800 bytes** ( minified + gzipped )
👬 A Friendly wrapper for your favourite DOM Apis ✨ in 800 bytes ( minified + gzipped ) 🙌
DollarDOM is not a polyfill or a new dom library. It's a simple wrapper for these mostly used DOM APIs:
DollarDOM abstracts the above methods and provides a $
object ( jQuery style ). It also has an on
method which can be chained with the $
selector function. Here is a simple example:
var collection = document.querySelectorAll('.some-class');
collection = [].slice.call(collection) // required for older browsers.
collection.forEach( elem => {
elem.addEventListener('click', function(){
console.log(this.innerHTML);
});
});
can be written as:
$('.some-class').on('click', function(){
console.log(this.innerHTML);
});
and in a better way, with event delegation:
$(document).on('click', '.some-class', function(){
console.log(this.innerHTML);
});
In bullet points, you can use DollarDOM, if:
using npm
npm install --save dollar-dom
using yarn
yarn add dollar-dom
If you're using module bundlers like webpack, Browserify or rollup, you can import $
from DollarDom module:
import {$} from 'dollar-dom';
// or
const {$} = require('dollar-dom');
If you wish to include as a script, DollarDOM can be included like this:
<script src="https://unpkg.com/dollar-dom/build/dollar-dom.min.js"></script>
and will be available as a global object named dollarDom
in the browser.
$
Create DOM from string:
Generating DOM from string is simple.
let newEl = $(`
<div class="parent">
<ul class="list">
<li class="child">1</li>
<li class="child">2</li>
<li class="child">3</li>
<li class="child">4</li>
<li class="child">5</li>
</ul>
<div class="section">
<span class="child">100</div>
</div>
</div>
`)
document.body.appendChild(newEl);
Single element selector ( same as querySelector ):
Let's try to find the element from the DOM we just created.
let parent = $('.parent');
console.log( parent.tagName ) // logs 'DIV'
You can limit the selector to any parent element
let child = $('.child', '.section');
console.log( child.tagName ) // logs 'SPAN'
// works with a parent dom element too
let listElement = $('.list').get(0);
let child = $('.child', listElement);
console.log( child.tagName ) // logs 'LI'
Multiple elements selector ( same as querySelectorAll ):
let children = $('.child');
children.forEach( child => {
console.log(child); // Logs LI, LI, LI, LI, LI, SPAN
});
// with a parent
let children = $('.child', '.section');
children.forEach( child => {
console.log(child); // Logs SPAN
});
Difference between a collection and a single element selector:
By default, $
returns a collection. But you can call any DOM element method on it, and it will be applied on the
first element of the collection. However, if you call the on
method ( which is dollarDOM specific ), it will be applied on all elements in the collection -- You can see that more in the on
section.
Example:
let out = $('.child', '.list');
out.forEach( child => {
console.log(child.innerHTML); // Logs 1, 2, 3, 4, 5
});
out.innerHTML = 'Hello';
out.forEach( child => {
console.log(child.innerHTML); // Logs Hello, 2, 3, 4, 5
});
on
Attach event handler:
$
makes attaching the event handler a lot easy. If you're coming from the jQuery world, there won't be any surprices.
// Events will be attached to each .child element
$('.child').on('click', function(e){
console.log( this.textContent ) // NOTE: "this" points to the element clicked. Make sure not to use arrow function as a handler
console.log( e ) // mouseClick event
});
Remove event handler:
The output of the on
method is a function which can be used to remove the attached event handlers.
let removeListeners = $('.child').on('click', function(e){
console.log( this.textContent );
});
// remove attached event handlers
removeListeners();
Event Delegation example:
In the above examples, the 'click' event will be attached in each .child
element. This is not performance friendly. DollarDOM has built-in event delegation support ( The syntax is similar to jQuery event delegation ).
// Only one event will be attached to the .parent element
$('.parent').on('click', '.child', function(e){
console.log( this.textContent ); // on click of the .child, it's textContent will be logged.
});
get
Get the element from collection:
get
is a utility method to get a single element from the collection. It accept an index
argument and the element in that position will be returned.
let collection = $('.child');
// NOTE: index starts from 0
let spanElement = collection.get(5);
console.log( spanElement.textContent ) // Logs 100
We love contributions from everyone.
yarn install
or npm install
AVA
for unit tests.
yarn test
or npm test
yarn test-w
or npm run test-w
yarn build
or npm build
.MIT @ Namshi.com
FAQs
👬 A Friendly wrapper for your favourite DOM Apis ✨ in **800 bytes** ( minified + gzipped )
The npm package dollar-dom receives a total of 1 weekly downloads. As such, dollar-dom popularity was classified as not popular.
We found that dollar-dom demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.