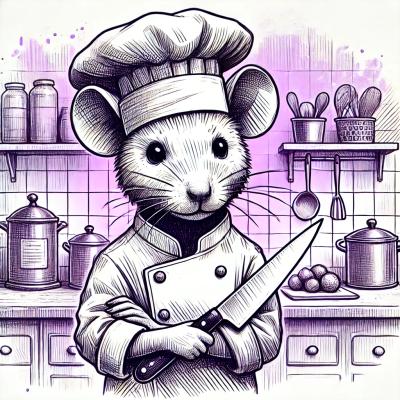
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
domain-browser
Advanced tools
Node's domain module for the web browser. This is merely an evented try...catch with the same API as node, nothing more.
The domain-browser package is a shim for Node.js's domain module, which allows you to handle multiple different IO operations as a single group. This is particularly useful in the context of handling errors across asynchronous operations. The package provides a way to group and handle errors and cleanup resources in client-side JavaScript that mimics Node.js's domain module functionality.
Error Handling
This feature allows grouping of IO operations and handling errors that occur within that group. The code sample demonstrates creating a domain, setting up an error handler, and running a function that throws an error asynchronously.
var domain = require('domain-browser');
var d = domain.create();
d.on('error', function(err) {
console.error('Caught error!', err);
});
d.run(function() {
setTimeout(function() {
throw new Error('Failed!');
}, 1000);
});
This package provides similar functionality to domain-browser by using async hooks to track asynchronous operations and errors. It differs in that it uses a more modern API provided by Node.js, which can offer better performance and more detailed control over asynchronous contexts.
Zone.js is another library that captures asynchronous operations into 'zones', which is similar to domains but with a broader scope that includes patching asynchronous APIs in the browser to provide context across async operations. It's more comprehensive than domain-browser, often used in frameworks like Angular for managing application state and change detection.
Node's domain module for the web browser. This is merely an evented try...catch with the same API as node, nothing more.
npm install --save domain-browser
import * as pkg from ('domain-browser')
const pkg = require('domain-browser')
<script type="module">
import * as pkg from '//dev.jspm.io/domain-browser@4.17.0'
</script>
This package is published with the following editions:
domain-browser
aliases domain-browser/source/index.js
domain-browser/source/index.js
is ES5 source code for web browsers and Node.js with Require for modulesDiscover the release history by heading on over to the HISTORY.md
file.
These amazing people are maintaining this project:
No sponsors yet! Will you be the first?
These amazing people have contributed code to this project:
Discover how you can contribute by heading on over to the CONTRIBUTING.md
file.
Unless stated otherwise all works are:
and licensed under:
FAQs
Node's domain module for the web browser. This is merely an evented try...catch with the same API as node, nothing more.
The npm package domain-browser receives a total of 4,565,935 weekly downloads. As such, domain-browser popularity was classified as popular.
We found that domain-browser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.