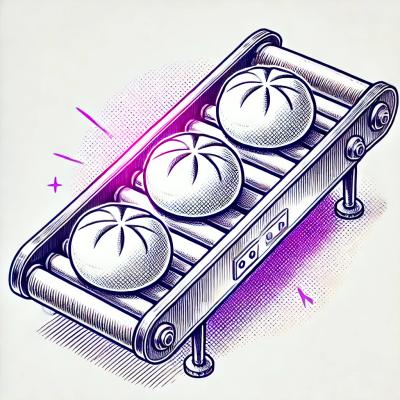
Security News
Bun 1.2 Released with 90% Node.js Compatibility and Built-in S3 Object Support
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Helper to quickly build up dom in javascript object format
Basic feature list:
And here's some code! :+1:
var root = Dominic.create({
cls: 'root', // or className: 'root' | cls as alias for className
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
items: [ // or: children
// also accept string, number as children, will be converted to text node
{ tag: 'div', width: 50, height: 50, text: 'Intro', display: 'inline-block', background: 'yellowgreen' },
{ tag: 'div', width: 200, background: 'lightgreen',
items: [
{ tag: 'div', width: 20, height: 20, background: 'red' },
{ tag: 'div', width: 20, height: 20, background: 'orange' },
],
created: function(el, root) {
// el: the div element with background: 'lightgreen' and 2 children 'red' & 'orange'
// root: the root elemnt directly created by Dominic.create
// this: = root element
}
}
],
created: function (el, root) {
// called when finished every setup
},
appended: function () {
// called when
// 1. element is root (created by Dominic.create)
// 2. parent is Node and appended element successfully
}
})
width
, height
, minHeight
, maxHeight
, minWidth
, maxWidth
margin
, padding
, margin
and padding
+ (Top - Left - Right - Bottom)
will be converted to proper CSS value if value is numbervar outerScopeDataSource = [
{ name: 'yellow' },
{ name: 'green' },
{ name: 'pink' }
]
var root = Dominic.create({
cls: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
items: [
{ width: 50, height: 50, text: 'Intro', display: 'inline-block', background: 'yellowgreen',
items: [
function () {
return outerScopeDataSource.map(function (data) {
return { tag: 'custom-el', text: data.name }
})
}
]
},
{ width: 200, background: 'lightgreen',
items: ['color', 'material'].map(function (val) {
return { text: val }
})
}
]
})
var root = Dominic.create({
cls: 'root',
parent: document.body,
width: '100%',
height: '100%',
defaults: {
display: 'inline-block',
height: '100%',
cls: 'default-class',
style: {
verticalAlign: 'top'
}
},
items: [
{ cls: 'sidebar', width: 200, ref: 'sidebar', background: 'lightgreen' },
{ cls: 'main', width: 'calc(100% - 200px)', ref: 'main', background: 'lightblue',
defaults: {
background: 'tomato',
margin: 5,
height: 50,
width: 50,
display: 'inline-block'
},
items: [
{ text: 1 },
{ text: 2 },
[3,4,5,6].map(function (v) { return { text: v } }),
function () {
return [5,6,7,8].map(function (v) { return { text: v } })
}
]
},
{ tag: 'test' }
]
})
display = 'inline-block', height = '100%'
class = 'default-class'
xtraCls
, xCls
: string
var root = Dominic.create({
cls: 'root',
parent: document.body,
width: '100%',
height: '100%',
defaults: {
cls: 'default-class',
},
items: [
{ xCls: 'sidebar' },
{ xtraCls: 'main' },
{ tag: 'test' }
]
})
if
/ hide
var root = Dominic.create({
className: 'root',
parent: document.body,
width: '100%',
height: '100%',
defaults: {
display: 'inline-block',
height: '100%',
className: 'default-class',
style: {
verticalAlign: 'top'
}
},
items: [
{ cls: 'sidebar', width: 200, ref: 'sidebar', background: 'lightgreen' },
{ cls: 'main',
width: 'calc(100% - 200px)',
ref: 'main',
background: 'lightblue',
defaults: {
background: 'tomato',
margin: 5,
height: 50,
width: 50,
display: 'inline-block'
},
items: [
{ text: 'First' },
{ text: 'Second' },
[3,4,5,6].map(function (v, i) {
return { text: 'Value is: ' + v, if: v < 4 }
}),
function () {
return [5,6,7,8].map(function (v) { return { text: v, hide: v > 6 } })
}
]
}
]
})
var root = Dominic.create({
className: 'root',
id: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
padding: 5,
attrs: {
class: 'original',
dataTooltip: 'halo this is tip',
'data-id': 5
}
})
var root = Dominic.create({
className: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
items: [
{ width: 50, height: 50, text: 'Intro', display: 'inline-block' },
{ width: 200, ref: 'orange', // access by root.refs.orange
items: [
{ width: 20, height: 20, background: 'red',
ref: 'lightgreen' // access by root.refs.lightgreen
},
{ width: 20, height: 20, background: 'orange',
// access by root.refs.orange.refs.orange
ref: 'orange', refScope: 'parent'
},
]
}
]
})
Reserved keyword for events:
click
mousedown
mouseup
mouseover
mouseout
mouseenter
mouseleave
mousemove
dragstart
dragend
drag
dragover
dragenter
dragleave
drop
blur
focus
keydown
keypress
keyup
change
input
submit
touchstart
touchmove
touchend
wheel
scroll
var root = Dominic.create({
className: 'root',
id: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
items: [
{ tag: 'div', width: 50, height: 50, text: 'Intro', display: 'inline-block', background: 'yellowgreen' },
{ tag: 'div', width: 200, background: 'lightgreen',
items: [
{ tag: 'div', className: 'red', width: 20, height: 20, background: 'red',
// normal click handler
click: {
handler: function (e) {
// div.red
console.log('This is:', this.localName + '.' + this.className)
}
}
},
{ tag: 'div', className: 'orange', width: 20, height: 20, background: 'orange',
click: {
// change scope to root element
scope: 'root',
handler: function (e) {
// div.root
console.log('This is:', this.localName + '.' + this.className)
}
},
events: [
{ type: 'custom:event', handler: function () {
console.log('This is div.orange')
}}
]
},
{ tag: 'div', className: 'yellow', width: 20, height: 20, background: 'yellow',
click: {
scope: 'root',
// Will look up for `onClickYellow` on root element
// Throw error if not found
handler: 'onClickYellow',
capture: true
}
}
]
}
],
onClickYellow: function (e) {
var t = e.target
// From div.yellow to div.root
console.log('From ', t.localName + '.' + t.className + ' to ' + this.localName + '.' + this.className)
},
events: [
{ type: 'mouseout', handler: function (e) {
console.log('Out of:', e.target)
}}
]
})
var root = Dominic.create({
cls: 'root',
id: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
items: [
{ width: 50, height: 50, text: 'Intro', display: 'inline-block', background: 'yellowgreen' },
{ width: 200, background: 'lightgreen',
items: [
{ cls: 'child red', width: 20, height: 20, background: 'red' },
{ cls: 'child orange', width: 20, height: 20, background: 'orange' },
{ cls: 'child yellow', width: 20, height: 20, background: 'yellow' }
],
click: { scope: 'root', handler: 'onClickLightgreen', delegate: '.child' }
}
],
onClickLightgreen: function (e, match, delegate) {
// this: scope when register event listener. Default: element has listener
// e: event object
// match: element matching delegate
// delegate: delegate css selector passed when register event listener
console.log('This is: ' + match.localName + '.' + match.className.replace(' ', '.'))
}
})
var root = Dominic.create({
cls: 'root',
parent: document.body,
width: '100%',
height: '100%',
defaults: {
cls: 'default-class',
},
items: [
{ xCls: 'sidebar' },
{ xtraCls: 'main' },
{ tag: 'input',
keydown: { scope: 'root', handler: 'sayHelo', key: 13 }
},
{ tag: 'input',
keydown: { scope: 'root', handler: 'onArrowKey', key: [37,38,39,40] }
}
],
sayHelo: function (e) {
console.log('helo', e.target.value)
},
onArrowKey: function (e) {
console.log('Navigating:', e.keyCode)
}
})
for
: data sourceTplFn
: function (item, itemIndex)var root = Dominic.create({
className: 'root',
id: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
padding: 5,
items: {
// data source
for: [
{ name: 'apple', cost: 0.5 },
{ name: 'mango', cost: 0.5 },
{ name: 'grape', cost: 0.6,
suppliers: { data: [
{ name: 'US', time: 5 },
{ name: 'UK', time: 4 }
]}
}
],
tplFn: function (item, itemIdx) {
return { tag: 'div', text: item.name, padding: 5, margin: '5px 0 0 5px', background: 'tomato',
items: {
for: item.suppliers,
root: 'data', // specify which property to look for data
tplFn: function (sup, supIdx) {
return { tag: 'div',
padding: 5,
background: 'lightblue',
text: sup.name + '. Time: ' + sup.time + ' days'
}
}
}
}
}
}
})
alwaysIterate
.var root = Dominic.create({
cls: 'root',
parent: document.body,
defaults: {
cls: 'default-class'
},
items: [
{ for: { a: 5, b: 6, c: 7},
observeProp: 'data1',
alwaysIterate: true,
// value & key instead value & index
tplFn: function (v, key) {
return { text: 'Value is: [' + v + ']. Key is: [' + key + ']' }
}
},
{ xCls: 'sidebar' },
{ xtraCls: 'main', items: {
for: [5,6,7,8],
observeProp: 'data2',
tplFn: function (v) { return v }
}},
]
})
// change:
root.observe.data1 = { name: 'Dominic', purpose: 'Helper', target: 'quick dom for test' }
root.observe.data2 = [ 'Helo ', 'This ', 'is ', 'a ', 'test ' ]
var src = [
{ name: 'apple', cost: 0.5 },
{ name: 'mango', cost: 0.5 },
{ name: 'grape', cost: 0.6,
suppliers: { data: [
{ name: 'US', time: 5 },
{ name: 'UK', time: 4 }
]}
}
]
var root = Dominic.create({
className: 'root',
id: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
padding: 5,
items: {
// data source
for: null,
// update this when root.observe.data = src
observeProp: 'data',
tplFn: function (item, itemIdx) {
return { tag: 'div', text: item.name, padding: 5, margin: '5px 0 0 5px', background: 'tomato',
items: {
for: item.suppliers,
root: 'data', // specify which property to look for data
tplFn: function (sup, supIdx) {
return {
tag: 'div',
padding: 5,
background: 'lightblue',
text: sup.name + '. Time: ' + sup.time + ' days',
click: { scope: 'root', handler: 'onClickSupplier' }
}
}
}
}
}
},
onClickSupplier: function (e) {
// Do something
},
events: [
{ type: 'mouseout', handler: function (e) {
console.log('Out of:', e.target)
}}
]
})
// first change
root.observe.data = src
// add more value
root.observe.push('data', {
name: 'mangox',
cost: 0.5,
suppliers: { data: [
{ name: 'Russia', time: 4 },
{ name: 'China', time: 5 }
]}
})
/**
* @param observeProperty {string}
* @param data {any}
*/
root.observe.push(observeProperty, data)
/**
* If index is absent, insert at the end, same behavior with push
* @param index? {int}
*/
root.observe.insert(observeProperty, index, data)
/**
* @param indexes {int[] | int}
*/
root.observe.remove(observeProperty, indexes)
/**
* These following methods have same behavior like in an array
*/
root.observe.pop(observeProperty)
root.observe.shift(observeProperty)
root.observe.unshift(observeProperty, data)
Dominic.register('input', function Input(defs) {
return {
tag: 'label',
parent: defs.parent,
display: 'block',
items: [
{ tag: 'span', items: { tag: 'b', text: 'Label name:' } },
{ tag: 'input', placeholder: 'Choose label name' }
]
}
})
Dominic.create({
ctype: 'input',
parent: document.body
})
// Define
Dominic.register('input', function Input(defs) {
return {
tag: 'label',
display: 'block',
items: [
{ tag: 'span', items: { tag: 'b', text: defs.label } },
{ tag: 'input', placeholder: 'Choose label name' }
]
}
})
// Define
Dominic.register('tab', function Tab(defs) {
var configs = {
cls: 'd-tab-ct',
parent: defs.parent,
items: [
{ cls: 'd-tab-btns',
defaults: {
tag: 'span',
display: 'inline-block',
height: 22,
padding: 4
},
items: {
for: defs.tabs,
observeProp: 'tabs',
tplFn: function(tab, i) {
return { text: tab.name }
}
}
},
{ cls: 'd-tab-tabs', items: {
for: defs.tabs,
observeProp: 'tabs',
tplFn: function(tab, i) {
console.log('tab data is', tab)
// Usage
return { ctype: 'input', label: 'Tab number ' + i }
}
}},
]
}
if (defs.created)
configs.created = defs.created
if (defs.appended)
configs.appended = defs.appended
return configs
})
Dominic.create({
ctype: 'tab',
parent: document.body,
tabs: [
{ name: 'Tab 1' },
{ name: 'Tab 2' },
{ name: 'Tab 3' }
],
created: function() {
console.log('finished initiating tab')
},
appended: function() {
console.log('Now in document')
}
})
Prototyping some design and testing event/ interaction made a bit more convinient when
<script src="dominic.min.js"></script>
npm i dominic
/**
* @param defs {Object} opts options for root element, className, id, children etc...
* @return {DOM}
*/
Dominic.create(defs)
/**
* @param name {string} component name
* @param fn {Function} function used by dominic to create component. should return an definition object
*/
Dominic.register(name, fn)
facebook
, twitter
, pinterest
tab
, combobox
, table
.MIT
FAQs
Helper to quickly build up DOM in simple javascript object format.
The npm package dominic receives a total of 74 weekly downloads. As such, dominic popularity was classified as not popular.
We found that dominic demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.