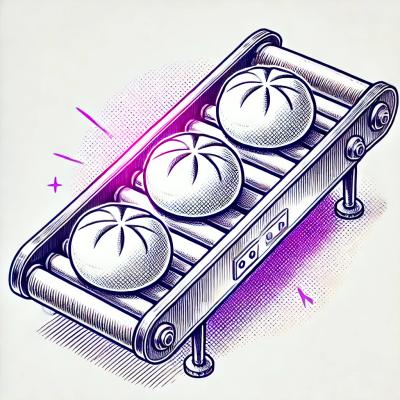
Security News
Bun 1.2 Released with 90% Node.js Compatibility and Built-in S3 Object Support
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Helper to quickly build up dom in javascript object format
Basic feature list:
And here's some code! :+1:
var root = CreateElement('div', {
className: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
items: [
{ tag: 'div', width: 50, height: 50, text: 'Intro', display: 'inline-block', background: 'yellowgreen' },
{ tag: 'div', width: 200, background: 'lightgreen',
items: [
{ tag: 'div', width: 20, height: 20, background: 'red' },
{ tag: 'div', width: 20, height: 20, background: 'orange' },
]
}
],
created: function () {
// called when finished every setup
},
appended: function () {
// called when
// 1. element is root (created by CreateElement)
// 2. parent is Node and appended element successfully
}
})
var outerScopeDataSource = [
{ name: 'yellow' },
{ name: 'green' },
{ name: 'pink' }
]
var root = CreateElement('div', {
className: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
items: [
{ tag: 'div', width: 50, height: 50, text: 'Intro', display: 'inline-block', background: 'yellowgreen',
items: [
function () {
return outerScopeDataSource.map(function (data) {
return { tag: 'custom-el', text: data.name }
})
}
]
},
{ tag: 'div', width: 200, background: 'lightgreen',
items: ['color', 'material'].map(function (val) {
return { tag: 'span', text: val }
})
}
]
})
var root = CreateElement('div', {
className: 'root',
id: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
padding: 5,
attrs: {
class: 'original',
dataTooltip: 'helo this is tip',
'data-id': 5
}
})
var root = CreateElement('div', {
className: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
items: [
{ tag: 'div', width: 50, height: 50, text: 'Intro', display: 'inline-block', background: 'yellowgreen' },
{ tag: 'div', width: 200, background: 'lightgreen',
items: [
{ tag: 'div', width: 20, height: 20, background: 'red',
ref: 'lightgreen' // access by root.refs.lightgreen
},
{ tag: 'div', width: 20, height: 20, background: 'orange', ref: 'orange',
ref: 'orange', refScope: 'parent' // access by root.refs.orange.refs.orange
},
]
}
]
})
var root = CreateElement('div', {
className: 'root',
id: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
items: [
{ tag: 'div', width: 50, height: 50, text: 'Intro', display: 'inline-block', background: 'yellowgreen' },
{ tag: 'div', width: 200, background: 'lightgreen',
items: [
{ tag: 'div', className: 'red', width: 20, height: 20, background: 'red',
click: {
handler: function (e) {
console.log('This is:', this.localName + '.' + this.className) // div.red
}
}
},
{ tag: 'div', className: 'orange', width: 20, height: 20, background: 'orange',
click: {
scope: 'root',
handler: function (e) {
console.log('This is:', this.localName + '.' + this.className) // div.root
}
},
events: [
{ type: 'custom:event', handler: function () {
console.log('This is div.orange')
}}
]
},
{ tag: 'div', className: 'yellow', width: 20, height: 20, background: 'yellow',
click: {
scope: 'root',
handler: 'onClickYellow',
capture: true
}
}
]
}
],
onClickYellow: function (e) {
var t = e.target
// From div.yellow to div.root
console.log('From ', t.localName + '.' + t.className + ' to ' + this.localName + '.' + this.className)
},
events: [
{ type: 'mouseout', handler: function (e) {
console.log('Out of:', e.target)
}}
]
})
var root = CreateElement('div', {
className: 'root',
id: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
padding: 5,
items: {
// data source
for: [
{ name: 'apple', cost: 0.5 },
{ name: 'mango', cost: 0.5 },
{ name: 'grape', cost: 0.6, suppliers: {
data: [
{ name: 'US', time: 5 },
{ name: 'UK', time: 4 }
]}
}
],
tplFn: function (item, itemIdx) {
return { tag: 'div', text: item.name, padding: 5, margin: '5px 0 0 5px', background: 'tomato',
items: {
for: item.suppliers,
root: 'data', // specify which property to look for data
tplFn: function (sup, supIdx) {
return { tag: 'div', padding: 5, background: 'lightblue', text: sup.name + '. Time: ' + sup.time + ' days' }
}
}
}
}
}
})
var src = [
{ name: 'apple', cost: 0.5 },
{ name: 'mango', cost: 0.5 },
{ name: 'grape', cost: 0.6, suppliers: {
data: [
{ name: 'US', time: 5 },
{ name: 'UK', time: 4 }
]}
}
]
var root = CreateElement('div', {
className: 'root',
id: 'root',
parent: document.body,
width: 300,
height: 300,
background: 'darkgreen',
padding: 5,
items: {
// data source
for: null,
//
update: {
// update this when root.observe.data = src
observeProp: 'data'
},
tplFn: function (item, itemIdx) {
return { tag: 'div', text: item.name, padding: 5, margin: '5px 0 0 5px', background: 'tomato',
items: {
for: item.suppliers,
root: 'data', // specify which property to look for data
tplFn: function (sup, supIdx) {
return {
tag: 'div',
padding: 5,
background: 'lightblue',
text: sup.name + '. Time: ' + sup.time + ' days',
click: { scope: 'root', handler: 'onClickSupplier' }
}
}
}
}
}
},
onClickSupplier: function (e) {
// Do something
},
events: [
{ type: 'mouseout', handler: function (e) {
console.log('Out of:', e.target)
}}
]
})
Prototyping some design and testing event/ interaction made a bit more convinient when
<script src="dominic.min.js"></script>
npm i dominic
CreateElement(name, opts)
name: String
opts: Object
.MIT
FAQs
Helper to quickly build up DOM in simple javascript object format.
The npm package dominic receives a total of 74 weekly downloads. As such, dominic popularity was classified as not popular.
We found that dominic demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.