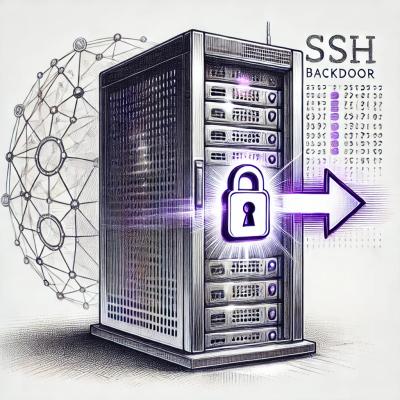
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
doofinder
Advanced tools
This library allows you to make requests to Doofinder Search Engines and show the results in your website. You'll be able to retrieve and shape your data easily with it.
npm install doofinder
bower install doofinder
https://raw.githubusercontent.com/doofinder/js-doofinder/master/dist/doofinder.min.js
We offer some simple CSS. You can download it in the link https://raw.githubusercontent.com/doofinder/js-doofinder/master/dist/doofinder.css
In this example we'll write a view that will just show results.
Let's begin by showing a simple HTML template (myview.html):
<html lang="en">
<head>
<script type="application/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/2.1.4/jquery.min.js"></script>
<script type="application/javascript" src="path/to/your/js/doofinder.min.js"></script>
<script>
(function(doofinder, document){
$(document).ready(function(){
// Use here your Search Engine hashid and doofinder zone
var client = new doofinder.Client('97be6c1016163d7e9bceedb5d9bbc032', 'eu1');
// #query is the DOM selector that points to the search box
var queryInputWidget = new doofinder.widgets.QueryInput('#query');
// #scroll is where we'll display the results
var resultsWidget = new doofinder.widgets.ScrollResults('#scroll');
// The controller to rule them all
var controller = new doofinder.Controller(
client,
[queryInputWidget, resultsWidget]
);
});
})(doofinder, document);
</script>
<style>
#scroll{
position: relative;
height: 800px;
overflow: auto;
}
</style>
</head>
<body>
<input type="text" id="query"/>
<div id="scroll">
</div>
</body>
Note that we are importing two javascript files:
We need to create the inner scroll via css. We get it setting the sroll wrapper's height and overflow as you can see above. It is also necessary set the position to "relative" in order to calculate the scroll position to ask for the next page.
The options we have filled in for the Client:
For the QueryInput widget:
For the ScrollResults widget:
WARNING: Note that Doofinder Search API is protected with CORS, so you must enable the host you are requesting from. You can do this from your Doofinder Administration Panel > Configuration > TODO(@ecoslado) This feature in Admin Panel.
With all this in place you'll have a search box where you can write a query and the results will be shown. Scrolling into the layer you'll see more results.
Controller is the class that manages client and widgets. Allows you to make different queries to your index and interact with the different widgets you will instantiate.
Argument | Required | Type | Description |
---|---|---|---|
client | Yes | doofinder.Client | The Search API wrapper |
widgets | Yes | doofinder.Widget Array(doofinder.Widget) | Array of widgets for interacting and rendering the results |
searchParameters | No | Object | An object with params that will passed to the client for every search. You can use here all the parameters defined in Doofinder Search API. |
This method makes a query to the Search API and renders the results into the widgets.
Argument | Required | Type | Description |
---|---|---|---|
query | Yes | String | The query terms. |
params | No | Object | An object with search parameters. You can use here all the parameters defined in Doofinder Search API. |
Asks for the next page of the previous search done, and sends the response to all widgets to render it.
Asks for a concrete page. Then pass the response to all widgets to render it.
Argument | Required | Type | Description |
---|---|---|---|
page | Yes | Number | The page requested |
Adds a filter to the currently applied in the search.
Argument | Required | Type | Description |
---|---|---|---|
facet | Yes | String | The name of the facet. |
value | Yes | String Object | The value the facet should have. This can be a String , if the facet is a term facet or an Object if it's a range. |
Removes a filter from the currently applied.
Argument | Required | Type | Description |
---|---|---|---|
facet | Yes | String | The name of the facet. |
value | Yes | String Object | The value of the facet. This can be a String , if the facet is a term facet or an Object if it's a range. |
Adds new search parameter to the current status.
Argument | Required | Type | Description |
---|---|---|---|
param | Yes | String | The name of the param. |
value | Yes | Mixed | The value of the param. |
Removes a parameter from the current status.
Argument | Required | Type | Description |
---|---|---|---|
param | Yes | String | The name of the param. |
Gets the params to the initial state.
Sets a param for every query.
Argument | Required | Type | Description |
---|---|---|---|
param | Yes | String | The name of the param. |
value | Yes | Mixed | The value of the param. |
Makes a search with the current filter status. Then, it calls to every widget to render the results.
Adds a widget to the controller after the instantiation.
Argument | Required | Type | Description |
---|---|---|---|
widget | Yes | doofinder.Widget | The widget to add. |
This method adds a callback to an event triggered from the controller. Events are triggered from the controller when a query is going to be done or when results are received.
Argument | Required | Type | Description |
---|---|---|---|
event | Yes | String | The event name. |
callback | Yes | Function | The function which receives the API Search response. |
The events you can bind in widget are the described above. Note that each event sends different arguments to the callback in order to implement it properly.
Event Name | Callback Arguments | Description |
---|---|---|
df:search |
| This event is triggered when controller.search is called. |
df:next_page |
| This event is triggered when controller.nextPage is called. |
df:get_page |
| This event is triggered when controller.getPage is called. |
df:results_received |
| This event is triggered when new results are received from Search API. |
As an example, we'll print in the console the total results in the df:results_received
event.
controller = new doofinder.Controller(client, widgets, options);
controller.bind('df:results_received', function(event, res){
console.log(res.total);
});
Sends a request to the Search API in order to account the hits for a product.
Argument | Required | Type | Description |
---|---|---|---|
dfid | Yes | String | Unique product identifier. |
Widgets are visual elements that take part into the search. They can be search inputs, places where display the results, places to put the facets, etc. The events you can bind in widget depend on the widget you are instantiating. Above we'll describe all the available widget and theirs correponding events.
This widget triggers searches when a user types on it.
Argument | Required | Type | Description |
---|---|---|---|
selector | Yes | String | Input CSS selector. |
options | No | Object | Options to configure the input. |
The options to configure the input are:
Option | Type | Description |
---|---|---|
wait | Number | milliseconds that the widget waits to check the input content length. |
captureLength | Number | number of Requireds typed when first search is performed |
This method adds a callback to an event triggered from the widget. Events are triggered from every widget when a query is going to be done or when results are received or when they are rendered in a widget.
Argument | Required | Type | Description |
---|---|---|---|
event | Yes | String | The event name |
callback | Yes | Function | The function which receives the API Search response. |
This widget shows the results in a DOM node. When a new search or filter is done or a new page is requested the new content will replace the older.
Argument | Required | Type | Description |
---|---|---|---|
container | Yes | String | Results container CSS selector. |
options | No | Object | Options to configure the input. |
The options to configure the widget are:
Option | Type | Description |
---|---|---|
template | String | Handlebars template to shape the results. |
templateVars | Object | Extra info you want to render in the template. Look at Example 2. |
templateFunctions | Object | Custom helpers to use in your template. Look at Example 3. |
This method adds a callback to an event triggered from the widget. Events are triggered from every widget when a query is going to be done or when results are received or when they are rendered in a widget.
Argument | Required | Type | Description |
---|---|---|---|
event | Yes | String | The query terms. |
callback | Yes | Function | The function which receives the API Search response. |
The events you can bind in widget are the described above. Note that each event sends different arguments to the callback in order to implement it properly.
Event Name | Callback Arguments | Description |
---|---|---|
df:rendered |
| This event is triggered when the results are rendered. |
As an example, we'll print in the console the total results in the df:rendered
event.
results = new doofinder.widgets.Results(container, options);
results.bind('df:rendered', function(event, res){
console.log(res.total);
});
This widget render the results in an DOM node with an inner scroll. So the next page will be requested when scroll reaches the bottom.
Argument | Required | Type | Description |
---|---|---|---|
container | Yes | String | Results container CSS selector. |
options | No | Object | Options to configure the input. |
The options to configure the widget are:
Option | Type | Description |
---|---|---|
template | String | Handlebars template to shape the results. |
templateVars | Object | Extra info you want to render in the template. Look at Example 2. |
templateFunctions | Object | Custom helpers to use in your template. Look at Example 3. |
scrollOffset | Number | Distance in pixels to the bottom in scroll when next page is requested. Default 50. |
There is some CSS you have to add to your container in order to make the inner scroll to work properly:
#container {
height: 800px;
position: relative;
overflow: auto;
}
In the example above #container
could be your scroll container selector and the height can be set to the value you prefer.
This method adds a callback to an event triggered from the widget. Events are triggered from every widget when a query is going to be done or when results are received or when they are rendered in a widget.
Argument | Required | Type | Description |
---|---|---|---|
event | Yes | String | The event name. |
callback | Yes | Function | The function which receives the API Search response. |
The events you can bind in widget are the described above. Note that each event sends different arguments to the callback in order to implement it properly.
Event Name | Callback Arguments | Description |
---|---|---|
df:rendered |
| This event is triggered when the results are rendered. |
df:hit |
| This event is triggered when a result link is clicked. |
As an example, we'll print in the console the total results in the df:rendered
event.
results = new doofinder.widgets.ScrollResults(container, options);
results.bind('df:rendered', function(event, res){
console.log(res.total);
});
This widget render a term facet in a list of terms.
Argument | Required | Type | Description |
---|---|---|---|
container | Yes | String | Results container CSS selector. |
name | Yes | String | The facet key. |
options | No | Object | Options to configure the input. |
The options to configure the widget are:
Option | Type | Description |
---|---|---|
template | String | Handlebars template to shape the results. |
templateVars | Object | Extra info you want to render in the template. Look at Example 2. |
templateFunctions | Object | Custom helpers to use in your template. Look at Example 3. |
This method adds a callback to an event triggered from the widget. Events are triggered from every widget when a query is going to be done or when results are received or when they are rendered in a widget.
Argument | Required | Type | Description |
---|---|---|---|
event | Yes | String | The event name. |
callback | Yes | Function | The function which receives the API Search response. |
The events you can bind in widget are the described above. Note that each event sends different arguments to the callback in order to implement it properly.
Event Name | Callback Arguments | Description |
---|---|---|
df:rendered |
| This event is triggered when the facet is rendered. |
As an example, we'll print in the console the total results in the df:rendered
event.
facet = new doofinder.widgets.TermFacet(container, options);
facet.bind('df:rendered', function(event, res){
console.log(res.total);
});
This widget render a range facet in a slider. To show it properly is necessary some CSS. You can add this stylesheet:
https://raw.githubusercontent.com/doofinder/js-doofinder/master/dist/doofinder.css
Argument | Required | Type | Description |
---|---|---|---|
container | Yes | String | Results container CSS selector. |
name | Yes | String | The facet key. |
options | No | Object | Options to configure the input. |
The options to configure the widget are:
Option | Type | Description |
---|---|---|
template | String | Handlebars template to shape the results. |
templateVars | Object | Extra info you want to render in the template. Look at Example 2. |
templateFunctions | Object | Custom helpers to use in your template. Look at Example 3. |
This method adds a callback to an event triggered from the widget. Events are triggered from every widget when a query is going to be done or when results are received or when they are rendered in a widget.
Argument | Required | Type | Description |
---|---|---|---|
event | Yes | String | The event name. |
callback | Yes | Function | The function which receives the API Search response. |
The events you can bind in widget are the described above. Note that each event sends different arguments to the callback in order to implement it properly.
Event Name | Callback Arguments | Description |
---|---|---|
df:rendered |
| This event is triggered when the facet is rendered. |
As an example, we'll print in the console the total results in the df:rendered
event.
facet = new doofinder.widgets.RangeFacet(container, options);
facet.bind('df:rendered', function(event, res){
console.log(res.total);
});
This is the class which makes the queries to the Doofinder Search API.
Argument | Required | Type | Description |
---|---|---|---|
hashid | Yes | String | The unique search engine identifier. |
API Key/Zone | Yes | String | The secret key to authenticate the request or the doofinder zone. |
types | No | Array | An array of datatypes to restrict the queries to them. |
WARNING: Do not use the API key unless you're coding on the server side, if you don't put the API key just put the doofinder zone instead.
This method performs a Search API call and retrieves the data. The data will be received by a callback function.
Argument | Required | Type | Description |
---|---|---|---|
query | Yes | String | The query terms. |
params | No | Object | The query terms. |
callback | Yes | Function | The function which receives the API Search response. |
In the Quick Start example we composed a simple view using the Doofinder Library. Let's see how to shape the results by a Handlebars template.
var resultsTemplate = '{{#each results}}' +
' <div>'+
' <div>' +
' <a href="{{href}}">'+
' <img src="{{image}}" alt="{{header}}">'+
' </a>'+
' <div>'+
' <a target="_blank" data-df-hitcounter="{{dfid}}" href="{{href}}">'+
' <div>{{header}}</div>' +
' <div>{{body}}</div>' +
' <div>' +
' <span>{{#format-currency}}{{price}}{{/format-currency}}</span>' +
' </div>'+
' </a>' +
' </div>' +
' </div>' +
'</div>' +
'{{/each}}';
var resultsWidget = new doofinder.widgets.ScrollResults('#scroll', {template: resultsTemplate});
Let's have a look to the template:
{{#each results}}
to iterate through the items.{{field_name}}
tags to print the content of a field.{{#if filed_name}}
to check the presence of a field.{{#format-currency}}
helper to print the price with the coin symbol and formatted.Maybe you want to add more information to your template dynamically. Imagine you want to show prices lower that a given number, i.e. 100. You can add this variable to the template scope by the option templateVars.
var resultsWidget = new doofinder.widgets.ScrollResults('#scroll', {
template: resultsTemplate,
templateVars: {
maxPrice: 100
}
});
So you can modify your template in order to show just the prices lower than maxPrice
.
var resultsTemplate = '{{#each results}}' +
' <div>'+
' <div>' +
' <a href="{{href}}">'+
' <img src="{{image}}" alt="{{header}}">'+
' </a>'+
' <div>'+
' <a target="_blank" data-df-hitcounter="{{dfid}}" href="{{href}}">'+
' <div>{{header}}</div>' +
' <div>{{body}}</div>' +
' {{#lt price ../maxPrice}}'
' <div>' +
' <span>{{#format-currency}}{{price}}{{/format-currency}}</span>' +
' </div>' +
' {{/lt}}' +
' </a>' +
' </div>' +
' </div>' +
'</div>' +
'{{/each}}';
var resultsWidget = new doofinder.widgets.ScrollResults('#scroll', {
template: resultsTemplate,
templateVars: {
maxPrice: 100
}
});
And we have used the {{#lt}}
helper to compare the price
with the maxPrice
. Note that we have written ../maxPrice
instead of maxPrice
. This is because we are in the loop and we must access to a variable out of results
array. For more information about Handlebars templates, click here.
Template functions are called helpers in Handlebars. You can create and add custom helpers to your template by using the templateFunctions
option. We'll create a helper that convert a text in bold.
{
templateFunctions: {
bold: function(options){
return "<b>" + options.fn(this) + "</b>"
}
}
This helper will take the text in the tag and will wrapper it with the <b>
tag. Note that options.fn(this)
returns the text wrapped by the helper.
We'll use the helper to show the header and instantiate the widget.
var resultsTemplate = '{{#each results}}' +
' <div>'+
' <div>' +
' <a href="{{href}}">'+
' <img src="{{image}}" alt="{{header}}">'+
' </a>'+
' <div>'+
' <a target="_blank" data-df-hitcounter="{{dfid}}" href="{{href}}">'+
' <div>{{#bold}}{{header}}{{/bold}}</div>' +
' <div>{{body}}</div>' +
' <div>' +
' <span>{{#format-currency}}{{price}}{{/format-currency}}</span>' +
' </div>'+
' </a>' +
' </div>' +
' </div>' +
'</div>' +
'{{/each}}';
var resultsWidget = new doofinder.widgets.ScrollResults('#scroll', {
template: resultsTemplate,
templateFunctions: {
bold: function(options){
return "<b>" + options.fn(this) + "</b>"
}
}
});
FAQs
Javascript Library for Doofinder Search API
The npm package doofinder receives a total of 79 weekly downloads. As such, doofinder popularity was classified as not popular.
We found that doofinder demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.