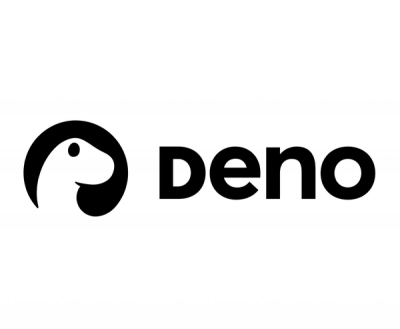
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
This library allows you to interact with DotCMS API's easily from the browser, nodejs and React Native. [Full Documentation](https://dotcms.github.io/core-web/dotcms/)
This library allows you to interact with DotCMS API's easily from the browser, nodejs and React Native. Full Documentation
npm install dotcms --save
or
yarn install dotcms
import { initDotCMS } from 'dotcms';
const dotcms = initDotCMS({
hostId: 'DOTCMS_SITE_IDENTIFIER',
host: 'YOUR_DOTCMS_INSTANCE',
token: 'YOUR AUTH TOKEN'
});
// Example
dotcms.page
.get({
url: '/about-us'
})
.then((data) => {
console.log(data);
})
.catch((err) => {
console.error(err.status, err.message);
});
Next.js gives you the best developer experience with all the features you need for production. Read more
import { useEffect, useState } from 'react';
import { initDotCMS } from 'dotcms';
const dotcms = initDotCMS({
host: 'YOUR_DOTCMS_INSTANCE',
token: 'YOUR AUTH TOKEN'
});
export default function Home() {
const [state, setState] = useState(null);
useEffect(async () => {
const page = await dotcms.page.get({
url: '/index'
});
setState(page);
}, []);
return state && <h1>{state.page.title}</h1>;
}
import { useEffect, useState } from 'react';
import { initDotCMS } from 'dotcms';
export default function Home(props) {
return <h1>{props.page.title}</h1>;
}
export async function getServerSideProps(context) {
const page = await dotcms.page.get({
url: context.req.url // you can map the urls with dotcms
});
return {
props: page // will be passed to the page component as props
};
}
More about data fetching in Nextjs.
Run nx test dotcms
to execute the unit tests.
This library was generated with Nx.
FAQs
This library allows you to interact with DotCMS API's easily from the browser, nodejs and React Native. [Full Documentation](https://dotcms.github.io/core-web/dotcms/)
The npm package dotcms receives a total of 113 weekly downloads. As such, dotcms popularity was classified as not popular.
We found that dotcms demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.