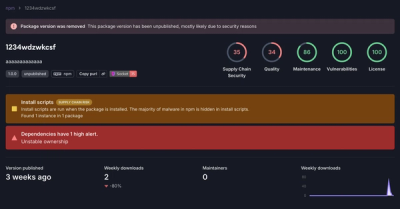
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
download-stats
Advanced tools
Get and calculate npm download stats for npm modules.
(TOC generated by verb using markdown-toc)
Install with npm:
$ npm install --save download-stats
var stats = require('download-stats');
Get a range of download counts for the specified repository. This method returns a stream of raw data in the form of { day: '2016-01-10', downloads: 123456 }
.
Example
var start = new Date('2016-01-09');
var end = new Date('2016-01-10');
stats.get(start, end, 'micromatch')
.on('error', console.error)
.on('data', function(data) {
console.log(data);
})
.on('end', function() {
console.log('done.');
});
// { day: '2016-01-09', downloads: 53331 }
// { day: '2016-01-10', downloads: 47341 }
Params
start
{Date}: Start date of stream.end
{Date}: End date of stream.repo
{String}: Repository to get downloads for. If repo
is not passed, then all npm downloads for the day will be returned.returns
{Stream}: Stream of download data.Calculate object containing methods to calculate stats on arrays of download counts. See [calculate][#calculate] api docs for more information.
Get a range of download counts for the specified repository. This method returns a stream of raw data in the form of { day: '2016-01-10', downloads: 123456 }
.
Example
var start = new Date('2016-01-09');
var end = new Date('2016-01-10');
stats.get(start, end, 'micromatch')
.on('error', console.error)
.on('data', function(data) {
console.log(data);
})
.on('end', function() {
console.log('done.');
});
// { day: '2016-01-09', downloads: 53331 }
// { day: '2016-01-10', downloads: 47341 }
Params
start
{Date}: Start date of stream.end
{Date}: End date of stream.repo
{String}: Repository to get downloads for. If repo
is not passed, then all npm downloads for the day will be returned.returns
{Stream}: Stream of download data.Get a specific point (all-time, last-month, last-week, last-day)
Example
stats.get.period('last-day', 'micromatch', function(err, results) {
if (err) return console.error(err);
console.log(results);
});
// { day: '2016-01-10', downloads: 47341 }
Params
period
{String}: Period to retrieve downloads for.repo
{String}: Repository to retrieve downloads for.cb
{Function}: Callback function to get resultsGet the all time total downloads for a repository.
Example
stats.get.allTime('micromatch', function(err, results) {
if (err) return console.error(err);
console.log(results);
});
// { day: '2016-01-10', downloads: 47341 }
Params
repo
{String}: Repository to retrieve downloads for.cb
{Function}: Callback function to get resultsGet the last month's total downloads for a repository.
Example
stats.get.lastMonth('micromatch', function(err, results) {
if (err) return console.error(err);
console.log(results);
});
// { downloads: 7750788, start: '2016-10-10', end: '2016-11-08', package: 'micromatch' }
Params
repo
{String}: Repository to retrieve downloads for.cb
{Function}: Callback function to get resultsGet the last week's total downloads for a repository.
Example
stats.get.lastWeek('micromatch', function(err, results) {
if (err) return console.error(err);
console.log(results);
});
// { downloads: 1777065, start: '2016-11-02', end: '2016-11-08', package: 'micromatch' }
Params
repo
{String}: Repository to retrieve downloads for.cb
{Function}: Callback function to get resultsGet the last day's total downloads for a repository.
Example
stats.get.lastDay('micromatch', function(err, results) {
if (err) return console.error(err);
console.log(results);
});
// { downloads: 316004, start: '2016-11-08', end: '2016-11-08', package: 'micromatch' }
Params
repo
{String}: Repository to retrieve downloads for.cb
{Function}: Callback function to get resultsGroup array into object where keys are groups and values are arrays. Groups determined by provided fn
.
Example
var groups = calculate.group(downloads, function(download) {
// day is formatted as '2010-12-25'
// add this download to the '2010-12' group
return download.day.substr(0, 7);
});
Params
arr
{Array}: Array of download objectsfn
{Function}: Function to determine group the download belongs in.returns
{String}: Key to use for the groupCalculate the total for each group (key) in the object.
Params
groups
{Object}: Object created by a group
function.returns
{Object}: Object with calculated totalsCalculate the total downloads for an array of download objects.
Params
arr
{Array}: Array of download objects (must have a .downloads
property)returns
{Number}: Total of all downloads in the array.Calculate the average for each group (key) in the object.
Params
groups
{Object}: Object created by a group
function.returns
{Object}: Object with calculated averageCalculate the average downloads for an array of download objects.
Params
arr
{Array}: Array of download objects (must have a .downloads
property)returns
{Number}: Average of all downloads in the array.Create an array of downloads before specified day.
Params
day
{String}: Day specifying last day to use in group.arr
{Array}: Array of downloads to check.returns
{Array}: Array of downloads happened before or on specified day.Calculate the total downloads happening before the specified day.
Params
day
{String}: Day specifying last day to use in group.arr
{Array}: Array of downloads to check.returns
{Number}: Total downloads happening before or on specified day.Create an array of downloads for the last X
days.
Params
days
{Number}: Number of days to go back.arr
{Array}: Array of downloads to check.init
{String}: Optional day to use as the last day to include. (Days from init || today
- days
to init || today
)returns
{Array}: Array of downloads for last X
days.Calculate total downloads for the last X
days.
Params
days
{Number}: Number of days to go back.arr
{Array}: Array of downloads to check.init
{String}: Optional day to use as the last day to include. (Days from init || today
- days
to init || today
)returns
{Array}: Array of downloads for last X
days.Create an array of downloads for the previous X
days.
Params
days
{Number}: Number of days to go back.arr
{Array}: Array of downloads to check.init
{String}: Optional day to use as the prev day to include. (Days from init || today
- days
- days
to init || today
- days
)returns
{Array}: Array of downloads for prev X
days.Calculate total downloads for the previous X
days.
Params
days
{Number}: Number of days to go back.arr
{Array}: Array of downloads to check.init
{String}: Optional day to use as the prev day to include. (Days from init || today
- days
- days
to init || today
- days
)returns
{Array}: Array of downloads for prev X
days.Create an object of download groups by month.
Params
arr
{Array}: Array of downloads to group and total.returns
{Object}: Groups with arrays of download objectsCalculate total downloads grouped by month.
Params
arr
{Array}: Array of downloads to group and total.returns
{Object}: Groups with total downloads calculatedCreate an object of download groups by month.
Params
arr
{Array}: Array of downloads to group and total.returns
{Object}: Groups with arrays of download objectsCalculate total downloads grouped by year.
Params
arr
{Array}: Array of downloads to group and total.returns
{Object}: Groups with total downloads calculatedPull requests and stars are always welcome. For bugs and feature requests, please create an issue.
(This document was generated by verb-generate-readme (a verb generator), please don't edit the readme directly. Any changes to the readme must be made in .verb.md.)
To generate the readme and API documentation with verb:
$ npm install -g verb verb-generate-readme && verb
Install dev dependencies:
$ npm install -d && npm test
Brian Woodward
Copyright © 2016, Brian Woodward. Released under the MIT license.
This file was generated by verb-generate-readme, v0.2.0, on November 09, 2016.
FAQs
Get and calculate npm download stats for npm modules.
The npm package download-stats receives a total of 285,933 weekly downloads. As such, download-stats popularity was classified as popular.
We found that download-stats demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.