Filter Draft.js content to preserve only the formatting you allow. Built for Draftail and Wagtail.
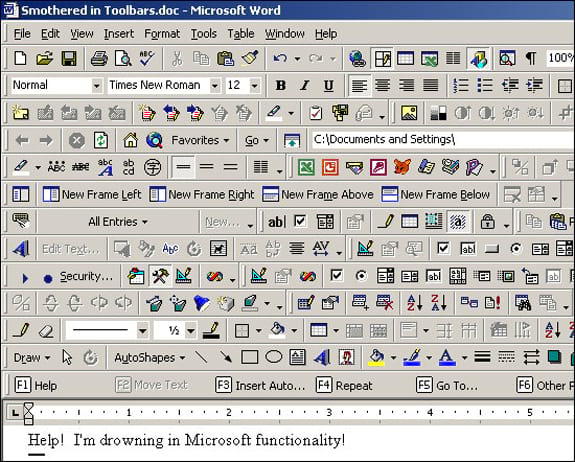
The main use case is to filter out disallowed formattings when copy-pasting rich text into an editor, for example from Word or Google Docs. Check out the online demo!
Using the filters
First, grab the package from npm:
npm install --save draftjs-filters
Then, in your editor import filterEditorState
and call it in the Draft.js onChange
handler. This function takes two parameters: the filtering configuration, and the editorState
.
import { filterEditorState } from "draftjs-filters"
function onChange(nextEditorState) {
const { editorState } = this.state
const content = editorState.getCurrentContent()
const nextContent = nextEditorState.getCurrentContent()
const shouldFilterPaste =
nextContent !== content &&
nextEditorState.getLastChangeType() === "insert-fragment"
let filteredEditorState = nextEditorState
if (shouldFilterPaste) {
filteredEditorState = filterEditorState(
{
blocks: ["header-two", "header-three", "unordered-list-item"],
styles: ["BOLD"],
entities: [
{
type: "IMAGE",
attributes: ["src"],
whitelist: {
src: "^http",
},
},
{
type: "LINK",
attributes: ["url"],
},
],
maxNesting: 1,
whitespacedCharacters: ["\n", "\t", "📷"],
},
filteredEditorState,
)
}
this.setState({ editorState: filteredEditorState })
}
Here are the available options:
blocks: Array<DraftBlockType>,
styles: Array<string>,
entities: Array<{
type: string,
attributes: Array<string>,
whitelist: Object,
}>,
maxNesting: number,
whitespacedCharacters: Array<string>,
Advanced usage
filterEditorState
isn't very flexible. If you want more control over the filtering, simply compose your own filter function with the other single-purpose utilities. The Draft.js filters are published as ES6 modules using Rollup – module bundlers like Rollup and Webpack will tree shake (remove) the unused functions so you only bundle the code you use.
preserveAtomicBlocks((content: ContentState))
resetAtomicBlocks((content: ContentState))
removeInvalidAtomicBlocks((whitelist: Array<Object>), (content: ContentState))
removeInvalidDepthBlocks((content: ContentState))
limitBlockDepth((max: number), (content: ContentState))
filterBlockTypes((whitelist: Array<DraftBlockType>), (content: ContentState))
filterInlineStyles((whitelist: Array<string>), (content: ContentState))
filterEntityRanges(
(filterFn: (
content: ContentState,
entityKey: string,
block: ContentBlock,
) => boolean),
(content: ContentState),
)
shouldKeepEntityType((whitelist: Array<Object>), (type: string))
shouldRemoveImageEntity((entityType: string), (blockType: DraftBlockType))
shouldKeepEntityByAttribute(
(entityTypes: Array<Object>),
(entityType: string),
(data: Object),
)
filterEntityData((entityTypes: Array<Object>), (content: ContentState))
replaceTextBySpaces((characters: Array<string>), (content: ContentState))
Browser support and polyfills
The Draft.js filters follow the browser support targets of Draft.js. Be sure to have a look at the required Draft.js polyfills.
Word processor support
Have a look at our test data in pasting/
.
Editor - Browser | Chrome Windows | Chrome macOS | Firefox Windows | Firefox macOS | Edge Windows | IE11 Windows | Safari macOS | Safari iOS | Chrome Android |
---|
Word 2016 | | | | | | | | N/A | N/A |
Word 2010 | | N/A | | N/A | | | N/A | N/A | N/A |
Apple Pages | N/A | | N/A | | N/A | N/A | | | N/A |
Google Docs | | | | | | | | | |
Word Online | | | | | | Unsupported | | ? | ? |
Dropbox Paper | | | | | | Unsupported | | ? | ? |
IE11
There are known Draft.js issues with pasting in IE11. For now, we advise users to turn on stripPastedStyles
in IE11 only so that Draft.js removes all formatting but preserves whitespace:
const IS_IE11 = !window.ActiveXObject && "ActiveXObject" in window
const editor = <Editor stripPastedStyles={IS_IE11} />
Contributing
See anything you like in here? Anything missing? We welcome all support, whether on bug reports, feature requests, code, design, reviews, tests, documentation, and more. Please have a look at our contribution guidelines.
Development
Install
Clone the project on your computer, and install Node. This project also uses nvm.
nvm install
npm install
./.githooks/deploy
Working on the project
Everything mentioned in the installation process should already be done.
nvm use
npm run start
npm run lint
npm run flow
npm run format
npm run test:watch
npm run test:coverage
npm run report:coverage
npm run report:build
npm run
Releases
Use npm run release
, which uses standard-version to generate the CHANGELOG and decide on the version bump based on the commits since the last release.
Credits
View the full list of contributors. MIT licensed. Website content available as CC0.
Microsoft Word toolbars screenshot from PCWorld – Microsoft Word Turns 25 article.