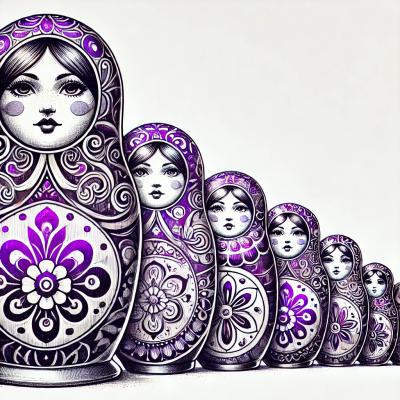
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
dsd-client-conn-lib
Advanced tools
Provides convenient way to call PDS server API of edzLabs.com's PDS service. Full API reference is located at [app.dase.io](https:*app.dase.io)
Provides convenient way to call PDS server API of edzLabs.com's PDS service. Full API reference is located at app.dase.io
var CONN = require("dsd-client-conn-lib");
var dsdConn = CONN.connClient2;
var dsdCst = CONN.Consts;
//default host for all is "partner.dase.io"
//but you may specify different hosts and ports like that:
//dsdConn.setCoreServer({ host: "partner.dase.io", port: 5090 });
//dsdConn.setDaseServer({ host: "partner.dase.io", port: 5100 });
//dsdConn.setPdsServer({ host: "partner.dase.io", port: 5200 });
//dsdConn.setKeychainServer({ host: "partner.dase.io", port: 5110 });
function log(title, msg) {
console.log(title, msg ? msg : "");
}
log("sendSilver should success");
dsdConn.getCoreClient().sendSilver({
senderId: "0x003e8c791cb39b4cad756b9c25431d6eb3fed85c",
senderPassword: "12345678",
silverAmount: 100,
toUserId: "0xb35ef3e31f2944d252eda42801caf5d890d1d7f9"
}).then(
ok => { log("sendSilver ok: ", ok); },
err => { log("sendSilver err: ", err); }
);
log("sendSilver2 should error");
dsdConn.getDaseClient().sendSilver({
senderId: "0x003e8c791cb39b4cad756b9c25431d6eb3fed85c",
senderPassword: "12345678",
toUserId: "0xb35ef3e31f2944d252eda42801caf5d890d1d7f9"
}).then(
ok => { log("sendSilver2 ok: ", ok); },
err => {
log("sendSilver2 err: ", err);
*specify error handlers if necessary
if(err.code === dsdCst.errorCodes.WrongParametersCount.id) {
log("I have wrong parameters count..."+dsdCst.errorCodes.WrongParametersCount.name);
*do something
}
else if(err.code === dsdCst.errorCodes.ServerSideError.id) {
log("I have server error..."+ dsdCst.errorCodes.ServerSideError.name);
*do something
}
}
);
console.log("addDab should success");
dsdConn.getPdsClient().addDab({
ownerId: "0x003e8c791cb39b4cad756b9c25431d6eb3fed85c",
assetUrl: "http:*localhost",
ownerPassword: "12345678",
publicDescription: "new client lib asset"
}).then(
ok => { log("addDab ok: ", ok); },
err => { log("addDab err: ", err); }
);
(Back)
Keys
Description: Method newUser
Keys
Description: Method newUser_Public
Keys
Description: Method getKeys
Keys
Description: Method getKeys_Public
Transaction
Description: Method sendWeis_Public
Integer
Description: Method getSilverBalance
Address
Description: Method getAddressByPubKey
Object
Description: Method getSystemAccounts
Integer
Description: Method getGoldBalance_Public
Transaction
Description: Method transferOut
Transaction
Description: Method transferOut_Public
Integer
Description: Method getBalanceWeis_Public
Integer
Description: Method buyGoldForWeis_Public
Integer
Description: Method weisAmountForGold_Public
Integer
Description: Method getLastBlock
Integer
Description: Method getLastBlock_Public
Integer
Description: Method silverCostInGold
Integer
Description: Method goldCostInSilver_Public
Transaction
Description: Method approveUserToSpendMySilver
Transaction
Description: Method approveTreasuryToSpendMySilver
Transaction
Description: Method sendSilver
Transaction
Description: Method sendGold_Public
Transaction
Description: Method spendAlienSilver
Boolean
Description: Method isValidUser
Boolean
Description: Method isValidUser_Public
Integer
Description: Method getEligibilityValue
Transaction
Description: Method wantVote
Integer
Description: Method getVoteRewardInSilver
Integer
Description: Method getVoteCostInSilver
Address
Description: Method getContractVoters
Address
Description: Method getQuorumVoters
Address
Description: Method getCandidatesPool
Boolean
Description: Method isInCandidatesPool
Boolean
Description: Method hasPledge
Boolean
Description: Method isLicensedForVoting
Address
Description: Method getMyCoinbase
Transaction
Description: Method withdrawPledge
Transaction
Description: Method offerBuyVoting
Boolean
Description: Method offerExchangeGoldForWeis
Boolean
Description: Method offerExchangeWeisForGold
Boolean
Description: Method recallExchangeOffer
Object
Description: Method getExchangeOffer
Object
Description: Method getExchangeOffers
Boolean
Description: Method acceptExchangeGoldForWeisOffer
Boolean
Description: Method acceptExchangeWeisForGoldOffer
Boolean
Description: Method declineExchangeOffer
Integer
Description: Method getExchangeOffersIdsToMe
Integer
Description: Method getExchangeOffersCountFor
Event
Description: Method getExchangePurchaseHistory
Event
Description: Method getExchangeSellHistory
Transaction
Description: Method sendMessageSecured
BytesArray
Description: Method getMessageSecured
Integer
Description: Method getMessagesSecuredCount
Integer
Description: Method getLastReadMessageIndex
Transaction
Description: Method setAttributeSecured
BytesArray
Description: Method getAttributeSecured
Keys
Description: Method newUser
Kind: global function
Returns: Keys
- Look at Type description in Docs.
Param | Type |
---|---|
password | String |
Keys
Description: Method newUser_Public
Kind: global function
Returns: Keys
- Look at Type description in Docs.
Param | Type |
---|---|
password | String |
Keys
Description: Method getKeys
Kind: global function
Returns: Keys
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
password | String |
Keys
Description: Method getKeys_Public
Kind: global function
Returns: Keys
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
password | String |
Transaction
Description: Method sendWeis_Public
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
sender | Address |
senderPassword | String |
receiver | Address |
weiAmount | Integer |
databytes | String |
Integer
Description: Method getSilverBalance
Kind: global function
Param | Type |
---|---|
userId | Address |
Address
Description: Method getAddressByPubKey
Kind: global function
Param | Type |
---|---|
pubKey | String |
Object
Description: Method getSystemAccounts
Integer
Description: Method getGoldBalance_Public
Kind: global function
Param | Type |
---|---|
userId | Address |
Transaction
Description: Method transferOut
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
srcUserId | Address |
password | String |
destUserId | Address |
amount | Integer |
Transaction
Description: Method transferOut_Public
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
srcUserId | Address |
password | String |
destUserId | Address |
amount | Integer |
Integer
Description: Method getBalanceWeis_Public
Kind: global function
Param | Type |
---|---|
userId | Address |
Integer
Description: Method buyGoldForWeis_Public
Kind: global function
Param | Type |
---|---|
userId | Address |
password | String |
goldAmount | Integer |
weisAmount | Integer |
Integer
Description: Method weisAmountForGold_Public
Kind: global function
Param | Type |
---|---|
userId | Address |
goldAmount | Integer |
Integer
Description: Method getLastBlock
Integer
Description: Method getLastBlock_Public
Integer
Description: Method silverCostInGold
Kind: global function
Param | Type |
---|---|
userId | Address |
silverAmount | Integer |
Integer
Description: Method goldCostInSilver_Public
Kind: global function
Param | Type |
---|---|
userId | Address |
goldAmount | Integer |
Transaction
Description: Method approveUserToSpendMySilver
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
fromUserId | Address |
password | String |
silverAmount | Integer |
toUserId | Address |
Transaction
Description: Method approveTreasuryToSpendMySilver
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
fromUserId | Address |
password | String |
silverAmount | Integer |
Transaction
Description: Method sendSilver
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
senderId | Address |
senderPassword | String |
silverAmount | Integer |
toUserId | Address |
Transaction
Description: Method sendGold_Public
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
senderId | Address |
senderPassword | String |
goldAmount | Integer |
toUserId | Address |
Transaction
Description: Method spendAlienSilver
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
spenderId | Address |
password | String |
fromUserId | Address |
silverAmount | Integer |
toUserId | Address |
Boolean
Description: Method isValidUser
Kind: global function
Param | Type |
---|---|
account | Address |
password | String |
Boolean
Description: Method isValidUser_Public
Kind: global function
Param | Type |
---|---|
account | Address |
password | String |
Integer
Description: Method getEligibilityValue
Kind: global function
Param | Type |
---|---|
userId | Address |
Transaction
Description: Method wantVote
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
userPassword | String |
iWish | Boolean |
Integer
Description: Method getVoteRewardInSilver
Kind: global function
Param | Type |
---|---|
userId | Address |
Integer
Description: Method getVoteCostInSilver
Kind: global function
Param | Type |
---|---|
userId | Address |
Address
Description: Method getContractVoters
Kind: global function
Returns: Address
- - Array of
Param | Type |
---|---|
userId | Address |
Address
Description: Method getQuorumVoters
Kind: global function
Returns: Address
- - Array of
Address
Description: Method getCandidatesPool
Kind: global function
Returns: Address
- - Array of
Param | Type |
---|---|
userId | Address |
Boolean
Description: Method isInCandidatesPool
Kind: global function
Param | Type |
---|---|
userId | Address |
Boolean
Description: Method hasPledge
Kind: global function
Param | Type |
---|---|
userId | Address |
Boolean
Description: Method isLicensedForVoting
Kind: global function
Param | Type |
---|---|
userId | Address |
Address
Description: Method getMyCoinbase
Transaction
Description: Method withdrawPledge
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
userPassword | String |
Transaction
Description: Method offerBuyVoting
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
userPassword | String |
silverAmount | Integer |
Boolean
Description: Method offerExchangeGoldForWeis
Kind: global function
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyer | Address |
goldAmount | Integer |
weisAmount | Integer |
Boolean
Description: Method offerExchangeWeisForGold
Kind: global function
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyer | Address |
goldAmount | Integer |
weisAmount | Integer |
Boolean
Description: Method recallExchangeOffer
Kind: global function
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
offerId | Integer |
Object
Description: Method getExchangeOffer
Kind: global function
Param | Type |
---|---|
userId | Address |
offerId | Integer |
Object
Description: Method getExchangeOffers
Kind: global function
Returns: Object
- - Array of
Param | Type |
---|---|
userId | Address |
offersIds | Integer |
Boolean
Description: Method acceptExchangeGoldForWeisOffer
Kind: global function
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
offerId | Integer |
goldAmount | Integer |
Boolean
Description: Method acceptExchangeWeisForGoldOffer
Kind: global function
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
offerId | Integer |
weisAmount | Integer |
Boolean
Description: Method declineExchangeOffer
Kind: global function
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
offerId | Integer |
Integer
Description: Method getExchangeOffersIdsToMe
Kind: global function
Returns: Integer
- - Array of
Param | Type | Description |
---|---|---|
userId | Address | |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
Integer
Description: Method getExchangeOffersCountFor
Kind: global function
Param | Type |
---|---|
userId | Address |
Event
Description: Method getExchangePurchaseHistory
Kind: global function
Returns: Event
- - Array of Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
startBlock | Integer |
Event
Description: Method getExchangeSellHistory
Kind: global function
Returns: Event
- - Array of Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
startBlock | Integer |
Transaction
Description: Method sendMessageSecured
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
userPassword | String |
cipherText | BytesArray |
BytesArray
Description: Method getMessageSecured
Kind: global function
Param | Type |
---|---|
userId | Address |
messageId | Integer |
Integer
Description: Method getMessagesSecuredCount
Kind: global function
Param | Type |
---|---|
userId | Address |
Integer
Description: Method getLastReadMessageIndex
Kind: global function
Param | Type |
---|---|
userId | Address |
Transaction
Description: Method setAttributeSecured
Kind: global function
Returns: Transaction
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
userPassword | String |
attrId | Integer |
cipherText | BytesArray |
BytesArray
Description: Method getAttributeSecured
Kind: global function
Param | Type |
---|---|
userId | Address |
attrId | Integer |
(Back)
Integer
Description: Method addDab
Integer
Description: Method addDabList
Integer
Description: Method addTag
Integer
Description: Method getTagsIdsForDab
Integer
Description: Method getOwnedDabsIds
Integer
Description: Method getSharedDabsIds
Integer
Description: Method getCustodiedDabsIds
Asset
Description: Method getDabs
Tag
Description: Method getTags
Integer
Description: Method getDabPreviousVersionsIds
Key
Description: Method getTagEncryptedKey
Dab
Description: Method exploreTagRelationsPage
Dab
Description: Method getAvailableDabs
License
Description: Method getAvailableLicenses
License
Description: Method getAvailableLicensesForDab
License
Description: Method getSellOfferedLicenses
License
Description: Method getBuyOfferedLicenses
License
Description: Method getSellOfferedLicensesForDab
License
Description: Method getBuyOfferedLicensesForDab
Boolean
Description: Method actualiseDeals
Asset
Description: Method getDabsPage
Boolean
Description: Method offerSellOwnership
Boolean
Description: Method offerBuyOwnership
Boolean
Description: Method approveOwnershipDeal
Boolean
Description: Method offerBuyCustody
Boolean
Description: Method offerSellCustody
Boolean
Description: Method approveCustodyDeal
Boolean
Description: Method offerSellAccess
Boolean
Description: Method offerBuyAccess
Boolean
Description: Method approveAccessDeal
Boolean
Description: Method offerSellReshare
Boolean
Description: Method offerBuyReshare
Boolean
Description: Method approveReshareDeal
Boolean
Description: Method offerSellTag
Boolean
Description: Method offerBuyTag
Boolean
Description: Method approveTagDeal
Boolean
Description: Method offerSellToTag
Boolean
Description: Method offerBuyToTag
Boolean
Description: Method approveToTagDeal
Boolean
Description: Method offerSellTagAccess
Boolean
Description: Method offerBuyTagAccess
Boolean
Description: Method approveTagAccessDeal
Boolean
Description: Method offerSellExplore
Boolean
Description: Method offerBuyExplore
Boolean
Description: Method approveExploreDeal
Boolean
Description: Method offerSellBulk
Boolean
Description: Method offerBuyBulk
Boolean
Description: Method approveBulkDeal
Boolean
Description: Method offerSellTransitive
Boolean
Description: Method offerBuyTransitive
Boolean
Description: Method approveTransitiveDeal
Integer
Description: Method getTotalLicenseFee
Boolean
Description: Method trustToBuyAccess
Boolean
Description: Method trustToBuyOwnership
Boolean
Description: Method untrustToBuyAccess
Boolean
Description: Method untrustToBuyOwnership
Deal
Description: Method getDealsForDab
Boolean
Description: Method addRelation
Boolean
Description: Method isHierarchicalChildOf
Integer
Description: Method checkUserPrvPayment
Integer
Description: Method addDataSecured
BytesArray
Description: Method getSecuredDabsInfoPage
BytesArray
Description: Method getSecuredDabsInfo
BytesArray
Description: Method listSecuredOwnedDabs
BytesArray
Description: Method listSecuredSharedDabs
BytesArray
Description: Method listSecuredCustodiedDabs
BytesArray
Description: Method getSecuredDabsInfo
Integer
Description: Method payBill
Integer
Description: Method addDab
Kind: global function
Param | Type | Description |
---|---|---|
ownerId | Address | |
ownerPassword | String | |
assetUrl | String | |
publicDescription | String | |
linkedDabId | Integer | (optional. default: 0) |
custodians | Address | (optional. default: []) - Array of |
publicityType | Integer | (optional. default: 0) Look at Constants description in Docs. |
dataHash | Integer | (optional. default: 0) |
Integer
Description: Method addDabList
Kind: global function
Returns: Integer
- - Array of
Param | Type | Description |
---|---|---|
ownerId | Address | |
ownerPassword | String | |
dataArr | Object | Array of |
Integer
Description: Method addTag
Kind: global function
Param | Type | Description |
---|---|---|
ownerId | Address | |
ownerPassword | String | |
publicDescription | String | |
custodians | Address | (optional. default: []) - Array of |
title | String | |
encryptedKey | String | (optional. default: null) |
Integer
Description: Method getTagsIdsForDab
Kind: global function
Returns: Integer
- - Array of
Param | Type |
---|---|
dabId | Integer |
requestor | Address |
Integer
Description: Method getOwnedDabsIds
Kind: global function
Returns: Integer
- - Array of
Param | Type |
---|---|
userId | Address |
pos | Integer |
size | Integer |
Integer
Description: Method getSharedDabsIds
Kind: global function
Returns: Integer
- - Array of
Param | Type |
---|---|
userId | Address |
Integer
Description: Method getCustodiedDabsIds
Kind: global function
Returns: Integer
- - Array of
Param | Type |
---|---|
userId | Address |
Asset
Description: Method getDabs
Kind: global function
Returns: Asset
- - Array of Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabIds | Integer | Array of |
Tag
Description: Method getTags
Kind: global function
Returns: Tag
- - Array of Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
tagIds | Integer | Array of |
Integer
Description: Method getDabPreviousVersionsIds
Kind: global function
Returns: Integer
- - Array of
Param | Type |
---|---|
dabId | Integer |
maxLength | Integer |
requestor | Address |
Key
Description: Method getTagEncryptedKey
Kind: global function
Returns: Key
- Look at Type description in Docs.
Param | Type |
---|---|
buyer | Address |
seller | Address |
tagId | Integer |
Dab
Description: Method exploreTagRelationsPage
Kind: global function
Returns: Dab
- - Array of Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
userPassword | String | |
rootTagId | Integer | |
tagId | Integer | |
pos | Integer | (optional. default: 0) |
Dab
Description: Method getAvailableDabs
Kind: global function
Returns: Dab
- - Array of Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Integer | Look at Constants description in Docs. |
licenseTypeCode | Integer | Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
License
Description: Method getAvailableLicenses
Kind: global function
Returns: License
- - Array of Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Integer | Look at Constants description in Docs. |
licenseTypeCode | Integer | Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
License
Description: Method getAvailableLicensesForDab
Kind: global function
Returns: License
- - Array of Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
dabId | Integer |
License
Description: Method getSellOfferedLicenses
Kind: global function
Returns: License
- - Array of Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Integer | Look at Constants description in Docs. |
licenseTypeCode | Integer | Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
License
Description: Method getBuyOfferedLicenses
Kind: global function
Returns: License
- - Array of Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
dabTypeCode | Integer | Look at Constants description in Docs. |
licenseTypeCode | Integer | Look at Constants description in Docs. |
pos | Integer | (optional. default: 0) |
size | Integer | (optional. default: 10) |
License
Description: Method getSellOfferedLicensesForDab
Kind: global function
Returns: License
- - Array of Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
dabId | Integer |
License
Description: Method getBuyOfferedLicensesForDab
Kind: global function
Returns: License
- - Array of Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
dabId | Integer |
Boolean
Description: Method actualiseDeals
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
userPassword | String | |
licenseTypeCode | Integer | Look at Constants description in Docs. |
Asset
Description: Method getDabsPage
Kind: global function
Returns: Asset
- - Array of Look at Type description in Docs.
Param | Type | Description |
---|---|---|
userId | Address | |
startDabId | Integer | (optional. default: 0) |
pageSize | Integer | (optional. default: 10) |
Boolean
Description: Method offerSellOwnership
Kind: global function
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyer | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Description: Method offerBuyOwnership
Kind: global function
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
dabId | Integer |
maxPrice | Integer |
Boolean
Description: Method approveOwnershipDeal
Kind: global function
Param | Type |
---|---|
custodian | Address |
custodianPassword | String |
buyer | Address |
dabId | Integer |
Boolean
Description: Method offerBuyCustody
Kind: global function
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
dabId | Integer |
maxPrice | Integer |
Boolean
Description: Method offerSellCustody
Kind: global function
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyer | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Description: Method approveCustodyDeal
Kind: global function
Param | Type |
---|---|
custodian | Address |
custodianPassword | String |
buyer | Address |
dabId | Integer |
Boolean
Description: Method offerSellAccess
Kind: global function
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyerId | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Description: Method offerBuyAccess
Kind: global function
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
dabId | Integer |
maxPrice | Integer |
Boolean
Description: Method approveAccessDeal
Kind: global function
Param | Type |
---|---|
custodian | Address |
custodianPassword | String |
buyer | Address |
dabId | Integer |
Boolean
Description: Method offerSellReshare
Kind: global function
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyerId | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Description: Method offerBuyReshare
Kind: global function
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
dabId | Integer |
maxPrice | Integer |
Boolean
Description: Method approveReshareDeal
Kind: global function
Param | Type |
---|---|
custodian | Address |
custodianPassword | String |
buyer | Address |
dabId | Integer |
Boolean
Description: Method offerSellTag
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
seller | Address | |
sellerPassword | String | |
buyerId | Address | |
dabId | Integer | (optional. default: 0) |
minPrice | Integer | |
tagId | Integer |
Boolean
Description: Method offerBuyTag
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
buyer | Address | |
buyerPassword | String | |
dabId | Integer | (optional. default: 0) |
maxPrice | Integer | |
tagId | Integer |
Boolean
Description: Method approveTagDeal
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
custodian | Address |
custodianPassword | String |
buyer | Address |
dabId | Integer |
tagId | Integer |
Boolean
Description: Method offerSellToTag
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
seller | Address | |
sellerPassword | String | |
buyerId | Address | |
buyerTagId | Integer | (optional. default: 0) |
minPrice | Integer | |
sellerDabId | Integer |
Boolean
Description: Method offerBuyToTag
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
buyer | Address | |
buyerPassword | String | |
buyerTagId | Integer | (optional. default: 0) |
maxPrice | Integer | |
sellerDabId | Integer |
Boolean
Description: Method approveToTagDeal
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
custodian | Address |
custodianPassword | String |
buyer | Address |
buyerTagId | Integer |
sellerDabId | Integer |
Boolean
Description: Method offerSellTagAccess
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyerId | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Description: Method offerBuyTagAccess
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
dabId | Integer |
maxPrice | Integer |
Boolean
Description: Method approveTagAccessDeal
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
custodian | Address |
custodianPassword | String |
buyer | Address |
dabId | Integer |
Boolean
Description: Method offerSellExplore
Kind: global function
Returns: Boolean
- isOK
Param | Type | Description |
---|---|---|
seller | Address | |
sellerPassword | String | |
buyerId | Address | |
dabId | Integer | |
minPrice | Integer | |
buyerPubKeyEncryptedTagKey | String | (optional. default: null) |
Boolean
Description: Method offerBuyExplore
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
dabId | Integer |
maxPrice | Integer |
Boolean
Description: Method approveExploreDeal
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
custodian | Address |
custodianPassword | String |
buyer | Address |
dabId | Integer |
Boolean
Description: Method offerSellBulk
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyerId | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Description: Method offerBuyBulk
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
dabId | Integer |
maxPrice | Integer |
Boolean
Description: Method approveBulkDeal
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
custodian | Address |
custodianPassword | String |
buyer | Address |
dabId | Integer |
Boolean
Description: Method offerSellTransitive
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyerId | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Description: Method offerBuyTransitive
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
buyer | Address |
buyerPassword | String |
dabId | Integer |
maxPrice | Integer |
Boolean
Description: Method approveTransitiveDeal
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
custodian | Address |
custodianPassword | String |
buyer | Address |
dabId | Integer |
Integer
Description: Method getTotalLicenseFee
Kind: global function
Param | Type | Description |
---|---|---|
licenseTypeCode | Integer | Look at Constants description in Docs. |
Boolean
Description: Method trustToBuyAccess
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyer | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Description: Method trustToBuyOwnership
Kind: global function
Returns: Boolean
- isOK
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyer | Address |
dabId | Integer |
minPrice | Integer |
Boolean
Description: Method untrustToBuyAccess
Kind: global function
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyer | Address |
dabId | Integer |
Boolean
Description: Method untrustToBuyOwnership
Kind: global function
Param | Type |
---|---|
seller | Address |
sellerPassword | String |
buyer | Address |
dabId | Integer |
Deal
Description: Method getDealsForDab
Kind: global function
Returns: Deal
- - Array of Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
dabId | Integer |
Boolean
Description: Method addRelation
Kind: global function
Param | Type | Description |
---|---|---|
userId | Address | |
userPassword | String | |
licenseTypeCode | Integer | Look at Constants description in Docs. |
fromDabId | Integer | |
toDabId | Integer |
Boolean
Description: Method isHierarchicalChildOf
Kind: global function
Param | Type |
---|---|
userId | Address |
parentDabId | Integer |
dabId | Integer |
Integer
Description: Method checkUserPrvPayment
Kind: global function
Returns: Integer
- msgId
Param | Type |
---|---|
msgId | Integer |
userId | Address |
userPassword | String |
userToCheck | Address |
zetonsAmount | Integer |
Integer
Description: Method addDataSecured
Kind: global function
Returns: Integer
- dabId
Param | Type |
---|---|
userId | Address |
userPassword | String |
dabHash | String |
cipherText | String |
BytesArray
Description: Method getSecuredDabsInfoPage
Kind: global function
Returns: BytesArray
- cipheredText - Array of
Param | Type | Description |
---|---|---|
userId | Address | |
startDabId | Integer | |
pageSize | Integer | (optional. default: 10) |
BytesArray
Description: Method getSecuredDabsInfo
Kind: global function
Returns: BytesArray
- cipheredText - Array of
Param | Type | Description |
---|---|---|
userId | Address | |
dabsIds | Integer | Array of |
BytesArray
Description: Method listSecuredOwnedDabs
Kind: global function
Returns: BytesArray
- cipheredText - Array of
Param | Type |
---|---|
userId | Address |
BytesArray
Description: Method listSecuredSharedDabs
Kind: global function
Returns: BytesArray
- cipheredText - Array of
Param | Type |
---|---|
userId | Address |
BytesArray
Description: Method listSecuredCustodiedDabs
Kind: global function
Returns: BytesArray
- cipheredText
Param | Type |
---|---|
userId | Address |
BytesArray
Description: Method getSecuredDabsInfo
Kind: global function
Returns: BytesArray
- cipheredText - Array of
Param | Type | Description |
---|---|---|
userId | Address | |
dabsIds | Integer | Array of |
Integer
Description: Method payBill
Kind: global function
Returns: Integer
- paidAmount
Param | Type |
---|---|
userId | Address |
userPassword | String |
price | Integer |
signature | String |
seller | Address |
(Back)
Vault
Description: Method listVaults
Ticket
Description: Method getUploadTicket
Ticket
Description: Method getAccessTicket
Ticket
Description: Method getAccessTicketSecured
Ticket
Description: Method getBulkAccessTicket
Vault
Description: Method listVaults
Kind: global function
Returns: Vault
- - Array of Look at Type description in Docs.
Param | Type |
---|---|
ownerId | Address |
Ticket
Description: Method getUploadTicket
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type |
---|---|
userId | Address |
password | String |
vaultAuth | Object |
Ticket
Description: Method getAccessTicket
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
dabId | Integer | |
buyer | Address | |
seller | Address | |
argsBuyerSignature | String | |
publicKey | String | |
vaultAuth | Object | (optional. default: null) |
Ticket
Description: Method getAccessTicketSecured
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
dealMessageId | Integer | |
buyer | Address | |
seller | Address | |
argsBuyerSignature | String | |
publicKey | String | |
vaultAuth | Object | (optional. default: null) |
Ticket
Description: Method getBulkAccessTicket
Kind: global function
Returns: Ticket
- Look at Type description in Docs.
Param | Type | Description |
---|---|---|
dabId | Integer | |
buyer | Address | |
bulkSeller | Address | |
tagId | Integer | |
argsBuyerSignature | String | |
publicKey | String | |
vaultAuth | Object | (optional. default: null) |
(Back)
Boolean
Description: Method newAccount
String
Description: Method getChallenge
BytesArray
Description: Method getEncryptedKeyData
Description: Method setEncryptedKeyData
Boolean
Description: Method newAccount
Kind: global function
Param | Type |
---|---|
account | String |
secret | String |
encryptedKeyData | BytesArray |
String
Description: Method getChallenge
Kind: global function
Param | Type |
---|---|
account | String |
BytesArray
Description: Method getEncryptedKeyData
Kind: global function
Param | Type |
---|---|
account | String |
responseOnChallenge | String |
Description: Method setEncryptedKeyData
Kind: global function
Returns: unspecified
Param | Type |
---|---|
account | String |
responseOnChallenge | String |
keyData | BytesArray |
FAQs
Provides convenient way to call PDS server API of edzLabs.com's PDS service. Full API reference is located at [app.dase.io](https:*app.dase.io)
The npm package dsd-client-conn-lib receives a total of 32 weekly downloads. As such, dsd-client-conn-lib popularity was classified as not popular.
We found that dsd-client-conn-lib demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.