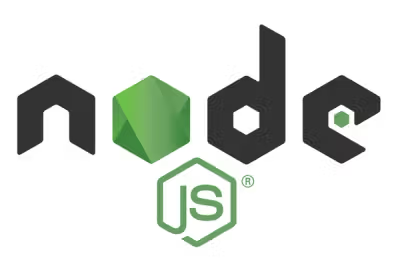
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
A preprocessor for Jest to snapshot test TypeScript declaration (.d.ts) files
A preprocessor for Jest to snapshot test TypeScript declaration (.d.ts) files
# using npm
npm install --save-dev dts-jest jest typescript
# using yarn
yarn add --dev dts-jest jest typescript
require jest@>=28
and typescript@>=4
dts-jest | jest | typescript |
---|---|---|
26 | >=28 | >=4 |
25 | >=28 <30 | >=2.3 <5 |
24 | 27 | >=2.3 <5 |
23 | >=22 <27 | >=2.3 <5 |
Modify your Jest config so that looks something like:
(./package.json)
{
"scripts": {
"test": "jest"
},
"jest": {
"moduleFileExtensions": ["ts", "js", "json"],
"testRegex": "/dts-jest/.+\\.ts$",
"transform": {"/dts-jest/.+\\.ts$": "dts-jest/transform"}
}
}
This setup allow you to test files **/dts-jest/**/*.ts
via dts-jest
.
The test cases must start with a comment @dts-jest
, and the next line should be an expression that you want to test its type or value.
(./dts-jest/example.ts)
// @dts-jest:pass:snap
Math.max(1);
// @dts-jest:pass
Math.min(1, 2, 3); //=> 1
// @dts-jest:fail:snap
Math.max('123');
// @ts-expect-error:snap
Math.max('123');
// @dts-jest[flags] [description]
expression //=> expected
// @ts-expect-error[flags] [description]
expression //=> expected
Note: @ts-expect-error
is treated as an alias of @dts-jest:fail
in dts-jest
.
expression
:show
: console.log(type)
:pass
: expect(() => type)
.not
.toThrow()
:fail
: expect(() => type)
.toThrow()
:snap
:
expect(type)
.toMatchSnapshot()
expect(type)
.toThrowErrorMatchingSnapshot()
:not-any
: expect(type)
.not
.toBe("any")
//=> expected
or /*=> expected */
?
: console.log(value)
:error
: expect(() => value)
.toThrow()
:no-error
: expect(() => value)
.not
.toThrow()
expect(value)
.toEqual(expected)
Test cases after this pattern will be marked as that group.
// @dts-jest:group[flag] [description]
If you need a block scope for your tests you can use a Block Statement.
// @dts-jest:group[flag] [description]
{
// your tests
}
''
describe
:only
: describe.only
:skip
: describe.skip
File-level config uses the first comment to set, only docblock will be detected.
/** @dts-jest [action:option] ... */
enable
: set to true
disable
: set to false
It's recommended you to run Jest in watching mode via --watch
flag.
npm run test -- --watch
NOTE: If you had changed the version of dts-jest
, you might have to use --no-cache
flag since Jest may use the older cache.
After running the example tests with npm run test
, you'll get the following result:
PASS tests/example.ts
Math.max(1)
✓ (type) should not throw error
✓ (type) should match snapshot
Math.max('123')
✓ (type) should throw error
✓ (type) should match snapshot
Math.min(1, 2, 3)
✓ (type) should not throw error
Snapshot Summary
› 2 snapshots written in 1 test suite.
Test Suites: 1 passed, 1 total
Tests: 5 passed, 5 total
Snapshots: 2 added, 2 total
Time: 0.000s
Ran all test suites.
Since snapshot testing will always pass and write the result at the first time, it's reommended you to use :show
flag to see the result first without writing results.
(./dts-jest/example.ts)
// @dts-jest:pass:show
Math.max(1);
// @dts-jest:fail:show
Math.max('123');
// @dts-jest:pass
Math.min(1, 2, 3); //=> 1
PASS dts-jest/example.ts
Math.max(1)
✓ (type) should show report
✓ (type) should not throw error
Math.max('123')
✓ (type) should show report
✓ (type) should throw error
Math.min(1, 2, 3)
✓ (type) should not throw error
Test Suites: 1 passed, 1 total
Tests: 5 passed, 5 total
Snapshots: 0 total
Time: 0.000s
Ran all test suites.
console.log dts-jest/example.ts:2
Inferred
Math.max(1)
to be
number
console.log dts-jest/example.ts:5
Inferring
Math.max('123')
but throw
Argument of type '"123"' is not assignable to parameter of type 'number'.
Configs are in _dts_jest_
field of Jest config globals
.
There are several options
true
false
false
typescript
(node resolution)<cwd>
available{}
tsconfig.json
(string) typeRoots
for object) to usets.TypeFormatFlags.NoTruncation
true
For example:
(./package.json)
{
"jest": {
"globals": {
"_dts_jest_": {
"compiler_options": {
"strict": true,
"target": "es6"
}
}
}
}
}
Originally, snapshots and source content are in different files, it is hard to check their difference before/after, so here comes the dts-jest-remap
for generating diff-friendly snapshots.
(./tests/example.ts)
// @dts-jest:snap
Math.max(1, 2, 3);
(./tests/__snapshots__
/example.ts.snap) note this file is generated by Jest
// Jest Snapshot v1, https://goo.gl/fbAQLP
exports[`Math.max(1, 2, 3) 1`] = `"number"`;
This command will combine both snapshots and source content in one file:
dts-jest-remap ./tests/example.ts --outDir ./snapshots
(./snapshots/example.ts)
// @dts-jest:snap -> number
Math.max(1, 2, 3);
Usage: dts-jest-remap [--outDir <path>] [--rename <template>] <TestFileGlob> ...
Options:
--check, -c Throw error if target content is different from output
content [boolean]
--help, -h Show help [boolean]
--listDifferent, -l Print the filenames of files that their target content is
different from output content [boolean]
--outDir, -o Redirect output structure to the directory [string]
--rename, -r Rename output filename using template {{variable}},
available variable: filename, basename, extname [string]
--typescript, -t Specify which TypeScript source to use [string]
--version, -v Show version number [boolean]
If you'd like to know which typescript you are using, add dts-jest/reporter
to your Jest reporters, for example:
{
"reporters": [
"default",
"dts-jest/reporter"
]
}
It'll show the TS version and path after testing:
[dts-jest] using TypeScript v0.0.0 from path/to/typescript
Compiler option 'lib' requires a value of type list
jest
> globals
> _dts_jest_
will be transformed into objects.setupFiles
to set configs (globals._dts_jest_ = { ... }
).Debug Failure
new RegExp('something')
.# lint
yarn run lint
# test
yarn run test
# build
yarn run build
MIT © Ika
26.0.2 (2024-02-25)
FAQs
A preprocessor for Jest to snapshot test TypeScript declaration (.d.ts) files
The npm package dts-jest receives a total of 314 weekly downloads. As such, dts-jest popularity was classified as not popular.
We found that dts-jest demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.