Easy Bluetooth Classic

A Library for easy implementation of Serial Bluetooth Classic on React Native. 💙
Looking for Bluetooth Low Energy? Look here.
Requirements
- Android 4.1 (API 16)
- React Native >= 0.40.0
Use
Configuration
import EasyBluetooth from 'easy-bluetooth-classic';
...
var config = {
"uuid": "00001101-0000-1000-8000-00805f9b34fb",
"deviceName": "Bluetooth Example Project",
"bufferSize": 1024,
"characterDelimiter": "\n"
}
EasyBluetooth.init(config)
.then(function (config) {
console.log("config done!");
})
.catch(function (ex) {
console.warn(ex);
});
Scanning
EasyBluetooth.startScan()
.then(function (devices) {
console.log("all devices found:");
console.log(devices);
})
.catch(function (ex) {
console.warn(ex);
});
Connecting
EasyBluetooth.connect(device)
.then(() => {
console.log("Connected!");
})
.catch((ex) => {
console.warn(ex);
})
Writing
EasyBluetooth.writeln("Works in React Native!")
.then(() => {
console.log("Writing...")
})
.catch((ex) => {
console.warn(ex);
})
Events
componentWillMount() {
this.onDeviceFoundEvent = EasyBluetooth.addOnDeviceFoundListener(this.onDeviceFound.bind(this));
this.onStatusChangeEvent = EasyBluetooth.addOnStatusChangeListener(this.onStatusChange.bind(this));
this.onDataReadEvent = EasyBluetooth.addOnDataReadListener(this.onDataRead.bind(this));
this.onDeviceNameEvent = EasyBluetooth.addOnDeviceNameListener(this.onDeviceName.bind(this));
}
...
onDeviceFound(device) {
console.log("onDeviceFound");
console.log(device);
}
onStatusChange(status) {
console.log("onStatusChange");
console.log(status);
}
onDataRead(data) {
console.log("onDataRead");
console.log(data);
}
onDeviceName(name) {
console.log("onDeviceName");
console.log(name);
}
...
componentWillUnmount() {
this.onDeviceFoundEvent.remove();
this.onStatusChangeEvent.remove();
this.onDataReadEvent.remove();
this.onDeviceNameEvent.remove();
}
Install
-
Run in console:
npm i -S easy-bluetooth-classic
-
Link:
react-native link easy-bluetooth-classic
-
Add jitpack repository in android/build.gradle
:
allprojects {
repositories {
...
maven { url "https://jitpack.io" }
}
}
Known issues
Contribute
New features, bug fixes and improvements are welcome! For questions and suggestions use the issues.
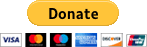
Licence
The MIT License (MIT)
Copyright (c) 2017 Douglas Nassif Roma Junior
See the full licence file.