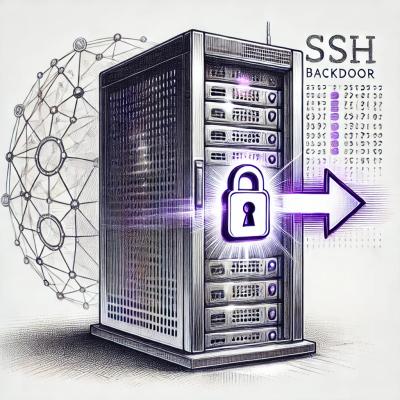
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
easy-mysql-js
Advanced tools
You can do MySQL database operations (select, insert, update, delete, insertOrUpdate) easily with this package.
Install the package
$ npm i easy-mysql-js
Initialize prisma
$ npx prisma init
NOTE: If you update your database tables, columns, etc. you need to run:
$ npx prisma db pull
$ npx prisma generate
To run this project, you will need to add the following environment variables to your .env file
DATABASE_URL="mysql://your_db_username:your_db_password@your_ip_address:3306/your_db_name"
NODE_ENV="development"
const {Select, SelectSingle, SelectMulti} = require('easy-mysql-js')
async function test() {
/*-----------------------------------------------------------------------------------------
--- SELECT --------------------------------------------------------------------------------
You can get records from the database with your custom sql query.
Returns an array
*/
const query = 'select * from users where id=1' // sql query
const select_result = await Select(query)
console.log(select_result)
// Success => [ { id: 1, name: 'qwe', email: 'qwe@asd.com', phone: '1235' } ]
// Error => []
// ----------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------
/*-----------------------------------------------------------------------------------------
--- SELECT SINGLE -------------------------------------------------------------------------
You can get single record from the database with unique column.
NOTE: Column in where_condition must be unique or primary key.
Returns an object
*/
const table_name = "users" // your database table name
const where_condition = {id:1} // => where id=1
const return_columns = {id:true,name:true,email:true} // => select id,name,email
const selectSingle_result = await SelectSingle(table_name, where_condition, return_columns)
console.log(selectSingle_result)
// Success => { id: 1, name: 'qwe', email: 'qwe@asd.com', phone: '1235' }
// Error => null
// ----------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------
/*-----------------------------------------------------------------------------------------
--- SELECT MULTI --------------------------------------------------------------------------
You can get multi records from the database with a column.
Returns an array
*/
const where_condition2 = {name:'test'} // => where name='test'
const return_columns = {id:true,name:true,email:true} // => select id,name,email
const selectMulti_result = await SelectMulti(table_name, where_condition, return_columns)
console.log(selectMulti_result)
// Success => [{ id: 1, name: 'qwe', email: 'qwe@asd.com', phone: '1235' },{ id: 2, name: 'asd', email: 't@t.com', phone: '12344955' }, ... ]
// Error => []
// ----------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------
}
test()
const {Insert} = require('easy-mysql-js')
async function test() {
/*-----------------------------------------------------------------------------------------
--- INSERT --------------------------------------------------------------------------------
You can insert a new record to database easily with table_name and data_to_insert parameters.
Returns true or false
*/
const table_name = "users" // your database table name
const data_to_insert = {name:"name",email:"asd@asd.com",phone:"1234567890"} // your table columns
const insert_result = await Insert(table_name, data_to_insert)
console.log(insert_result)
// Success => true
// Error => false
// ----------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------
}
test()
const {Update} = require('easy-mysql-js')
async function test() {
/*-----------------------------------------------------------------------------------------
--- UPDATE --------------------------------------------------------------------------------
You can update the record in the database with table_name, where_condition and data_to_set
parameters.
Returns true or false
*/
const table_name = "users" // your database table name
const where_condition = {email:"asd@asd.com"} // where email='asd@asd.com'
const data_to_set = {email:"qwe@qwe.com"} // set email='qwe@qwe.com'
const update_result = await Update(table_name, where_condition, data_to_set)
console.log(update_result)
// Success => true
// Error => false
// ----------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------
}
test()
const {InsertOrUpdate} = require('easy-mysql-js')
async function test() {
/*-----------------------------------------------------------------------------------------
--- INSERT OR UPDATE ----------------------------------------------------------------------
You can insert or update the record easily with table_name, where_condition, data_to_update
and data_to_insert parameters.
If the record exists then it will be updated. If the record does not exist then it will be
inserted.
Returns true or false
*/
const table_name = "users" // your database table name
const where_condition = {id:1} // where id=1
const data_to_update = {phone:"123123"} // if the record exists then update the columns
const data_to_insert = {name:"name2",email:"qwe@qwe.com",phone:"1234567891"} // if the record does not exist then add a new record
const insertOrUpdate_result = await InsertOrUpdate(table_name, where_condition, data_to_update, data_to_insert)
console.log(insertOrUpdate_result)
// Success => true
// Error => false
// ----------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------
}
test()
const {Delete} = require('easy-mysql-js')
async function test() {
/*-----------------------------------------------------------------------------------------
--- DELETE --------------------------------------------------------------------------------
You can delete the records from the database with where_condition.
Returns true or false
*/
const table_name = "users" // your database table name
const where_condition = {id:1} // where id=1
const delete_result = await Delete(table_name, where_condition)
console.log(delete_result)
// Success => true
// Error => false
// ----------------------------------------------------------------------------------------
// ----------------------------------------------------------------------------------------
}
test()
This project is licensed under the MIT License
FAQs
Easy MySQL for NodeJS
The npm package easy-mysql-js receives a total of 11,408 weekly downloads. As such, easy-mysql-js popularity was classified as popular.
We found that easy-mysql-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.