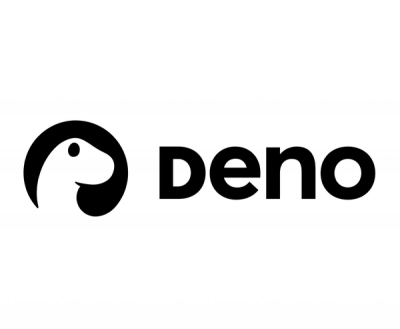
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
eden-class
Advanced tools
#Class
[DESCRIPTION]
npm install eden-class
var class = require('eden-class');
[Argument] argument();
Argument Testing
[Argument]
class().argument();
RESULTS
object capture(Bool);
For Async we lose the protected and private access. This grabs it before it is removed from the scope
object
class().capture();
RESULTS
this inspect(Mixed, String|null);
Force outputs any class property
mixed
string|null
this
class().inspect();
RESULTS
this loadState(*string);
Returns a state that was previously saved
this
class().loadState();
RESULTS
this loop(*function, Integer);
Loops through returned result sets
*function
integer
this
class().loop();
RESULTS
this on(*string, *function, Bool);
Attaches an instance to be notified when an event has been triggered
*string
*function
bool
this
class().on();
RESULTS
this once(*string, Function);
Attaches an instance to be notified when an event has been triggered, when the event was fired it will be removed on the event stack.
*string
function
this
class().once();
RESULTS
this saveState(*string, Mixed);
Sets instance state for later usage.
*string - the state name
mixed
this
class().saveState();
RESULTS
[Syncopate] sync(Function);
Starts a synchronous thread
[Syncopate]
class().sync();
RESULTS
this trigger(*string, Mixed[,mixed..]);
Notify all observers of that a specific event has happened
*string
mixed[,mixed..]
this
class().trigger();
RESULTS
this when(*mixed, *function);
Invokes Callback if conditional callback is true
*mixed
*function
this
class().when();
RESULTS
FAQs
Eden JS Base Class
We found that eden-class demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.