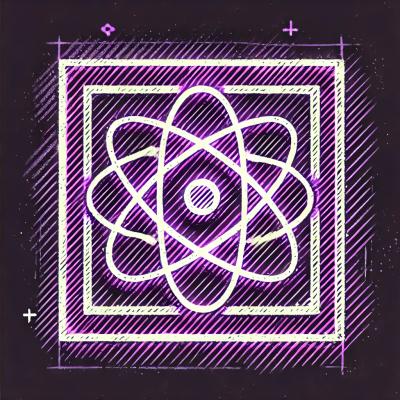
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
effects-as-data-server
Advanced tools
effects-as-data-server
is a lightweight server built on Koa. It is designed for building web applications with effects-as-data.
The guiding principals behind the development of this server are:
ctx
object and "return" by settings properties on that object.effects-as-data
. This means rapid development and clean code. In my experience, web servers have a way of turning into spaghetti quickly.npm i effects-as-data-server
The following middleware is enabled by default. All, except koa-router
, can be disabled. See init below.
effects-as-data-server
comes bundled with effects-as-data-universal
by default.
const { init, cmds, send, router } = require("effects-as-data-server");
function* helloWorld({ body, query, params, headers, cookies }) {
const result = yield cmds.echo("hello world");
return send(result);
}
const { start, stop } = init({
port: 3000,
routes: [router.get("/hello-world", helloWorld)]
});
// Start the server
start().catch(console.error);
const { helloWorld } = require("./path/to/hello-world");
const { args, testFn } = require("effects-as-data/test");
const { cmds } = require("effects-as-data-server");
test(
'helloWorld() should return "hello world"',
testFn(helloWorld, () => {
return args()
.yieldCmd(cmds.echo("hello world"))
.yieldReturns("hello world")
.returns(send("hello world"));
})
);
const { init } = require('effects-as-data-server');
function myCustomInterpreter () {
return 'I always return this';
}
const { start, stop } = init({
port: 3000,
interpreters: {
myCustomInterpreter
},
...
});
// Start the server
start().catch(console.error);
Just normal Koa.
const { init, router } = require("effects-as-data-server");
// Just a normal koa middleware function
function helloWorld({ ctx, body, query, params, headers, cookies }) {
ctx.body = "hello world";
}
const { start, stop } = init({
port: 3000,
routes: [router.get("/hello-world", helloWorld)]
});
// Start the server
start().catch(console.error);
The init function accepts an array of middleware functions.
const { init, router } = require('effects-as-data-server');
// Just a normal koa middleware function
function customMiddleware (ctx, next) {
const start = Date.now();
await next();
const ms = Date.now() - start;
ctx.set('X-Response-Time', `${ms}ms`);
}
const { start, stop } = init({
port: 3000,
middlware: [customMiddleware]
});
// Start the server
start().catch(console.error);
The following functions are exported from effects-as-data-server
.
init
is used to initialize a server.
options
<[Object]> Effects-as-data server options. Can have the following fields:
routes
<[Array]> An array of routes created using the router
.context
<[Object]> Effects-as-data context.helmet
<[Object]> Options to be passed to koa-helmet
.disableHelmet
<[boolean]> Disable koa-helmet
if true
. Default is false
.middleware
<[Array]> An array of Koa middleware.port
<[Number]> The port on which the server runs.cookie
<[Object]> Options to be passed to koa-cookie
.disableCookie
<[Boolean]> Disable koa-cookie
if true
. Default is false
.bodyParser
<[Object]> Options to be passed to koa-bodyparser
.disableBodyParser
<[Boolean]> Disable koa-bodyparser
if true
. Default is false
.test
<[Boolean]> If true
, don't print startup messages to console. Default is false
.Returns: An object with a start
and stop
function used to start and stop the server, respectively.
Create a response to be returned by an EAD function and sent to the client.
body
<[Any]> The response body. requiredstatus
<[Number]> The response status. Ex: 200
, 204
, 400
, etc.headers
<[Object]> An object of headers. Ex: { 'X-Response-Time': 32 }
cookies
<[Array]> An array of cookies created using the createCookie
function.Returns: An object containing the response body, status, headers, and cookies.
Create a not found response to be returned by an EAD function and sent to the client. This is a convenience function that wraps send
.
body
<[Any]> The response body. requiredheaders
<[Object]> An object of headers. Ex: { 'X-Response-Time': 32 }
cookies
<[Array]> An array of cookies created using the createCookie
function.Returns: An object containing the response body, status of 404, headers, and cookies.
Create a not authorized response to be returned by an EAD function and sent to the client. This is a convenience function that wraps send
.
body
<[Any]> The response body. requiredheaders
<[Object]> An object of headers. Ex: { 'X-Response-Time': 32 }
cookies
<[Array]> An array of cookies created using the createCookie
function.Returns: An object containing the response body, status of 401, headers, and cookies.
Create a cookie which, internall, will be set with Koa's ctx.cookies.set
.
name
<[String]> Name of the cookie. requiredvalue
<[String]> Value of the cookie. requiredoptions
<[Object]> Options for Koa's ctx.cookies.set
function which is used to set the cookie.Returns: A cookie to be set.
The router is exported from effects-as-data-server
:
const { router } = require('effects-as-data-server');
const { start, stop } = init({
port: 3000,
routes: [
router.get('/api/users', function * () { ... })
router.get('/api/users/:id', function * () { ... })
router.post('/api/users', function * () { ... })
router.put('/api/users/:id', function * () { ... })
],
...
});
path
<[String]> A string path for this route. Ex: /api/users
function
<[Function]> An EAD function or a Koa middleware function.Returns: A route for the routes
array passed to the init
function.
path
<[String]> A string path for this route. Ex: /api/users
function
<[Function]> An EAD function or a Koa middleware function.Returns: A route for the routes
array passed to the init
function.
path
<[String]> A string path for this route. Ex: /api/users/32
function
<[Function]> An EAD function or a Koa middleware function.Returns: A route for the routes
array passed to the init
function.
path
<[String]> A string path for this route. Ex: /api/users/32
function
<[Function]> An EAD function or a Koa middleware function.Returns: A route for the routes
array passed to the init
function.
path
<[String]> A string path for this route. Ex: /api/users/32
function
<[Function]> An EAD function or a Koa middleware function.Returns: A route for the routes
array passed to the init
function.
FAQs
A simple server for effects-as-data
The npm package effects-as-data-server receives a total of 0 weekly downloads. As such, effects-as-data-server popularity was classified as not popular.
We found that effects-as-data-server demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.