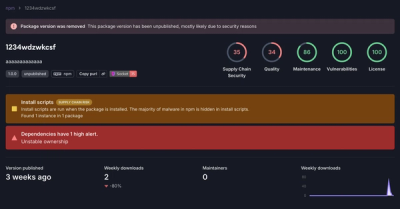
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
A convenient way to interact with the Elarian APIs.
You can install the package from npm by running:
$ npm install elarian
// on node
const { Elarian } = require('elarian');
/*
or in the browser
<script src="dist/elarian.min.js"></script>
const { Elarian } = ElarianSDK;
*/
// ...
const client = await Elarian.newInstance({
apiKey: 'YOUR_API_KEY', // or authToken: 'YOUR_AUTH_TOKEN'
orgId: 'YOUR_ORG_ID',
appId: 'YOUR_APP_ID',
});
client.on('ussdSession', async ({ data, customer}, callback) => {
const {
input,
sessionId,
} = data;
const appData = await customer.leaseAppData();
let {
name,
state = 'newbie',
} = appData;
const menu = {
text: null,
isTerminal: true,
};
switch (state) {
case 'veteran':
if (name) {
menu.text = `Welcome back ${name}! What is your new name?`;
menu.isTerminal = false;
} else {
name = input.value;
menu.text = `Thank you for trying Elarian, ${name}!`;
menu.isTerminal = true;
await customer.sendMessage(
{ number: 'Elarian', provider: 'telco' },
{ text: `Hey ${name}! Thank you for trying out Elarian` },
);
}
break;
case 'newbie':
default:
menu.text = 'Hey there, welcome to Elarian! What\'s your name?';
menu.isTerminal = false;
state = 'veteran';
break;
}
await customer.updateAppData({ state, name });
callback(null, menu);
});
Take a look at the API docs here. For detailed info on this SDK, see the documentation.
Run all tests:
$ npm install
$ npm test
If you find a bug, please file an issue on our issue tracker on GitHub.
FAQs
Elarian JavaScript SDK
The npm package elarian receives a total of 13 weekly downloads. As such, elarian popularity was classified as not popular.
We found that elarian demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.