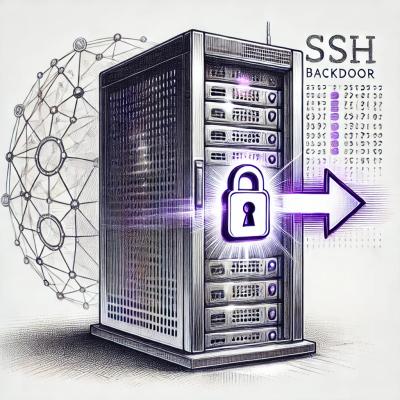
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
electra-js
Advanced tools
Javascript API middleware allowing clients to interact with Electra blockchain.
Javascript API allowing clients to interact with Electra blockchain.
npm i electra-js
All the wallet methods are contained within the composed method .wallet
of the instanciated ElectraJs
class.
Case A: Light Wallet
In the case of a light wallet (listening and broadcasting to the blockchain via public web-services), you only need to instanciate ElectraJs without any setting:
import ElectraJs from 'electra-js'
const electraJs = new ElectraJs()
// We can then call electraJs.wallet.anyWalletMethod(...) to start using it.
Case B: Hard Wallet
In the case of a hard wallet, that is utilizing the deamon RPC server, you need to specify the RPC server settings during the ElectraJs instanciation:
import ElectraJs from 'electra-js'
const electraJs = new ElectraJs({
rpcServerAuth: {
username: 'user',
password: 'pass'
},
rpcServerUri: 'http://127.0.0.1:5788'
})
// We can then call electraJs.wallet.anyWalletMethod(...) to start using it.
The wallet can bear 2 states: EMPTY
or READY
. It will always start as EMPTY
once intanciated.
The first wallet method that MUST be called in any case is electraJs.wallet.generate()
(described afterwards).
Note
<parameter>
is a mandatory parameter.
[parameter]
is an optional parameter.
Getters:
wallet.addresses
List of the wallet HD addresses.
Response:
Array<{
hash: string
isCiphered: boolean
isHD: boolean
label: string
privateKey: string
}>
wallet.allAddresses
List of the wallet non-HD (random) and HD addresses.
Response:
Array<{
hash: string
isCiphered: boolean
isHD: boolean
label: string
privateKey: string
}>
wallet.isHD
Is this a HD wallet ?
Response:
boolean
wallet.lockState
Is this wallet locked ?
In the case of a light wallet, "STAKING" state can't happen.
Response:
enum {
LOCKED = 'LOCKED',
STAKING = 'STAKING',
UNLOCKED = 'UNLOCKED'
}
wallet.mnemonic
Wallet HD Mnenonic.
ONLY available when generating a brand new Wallet, which happens after calling #generate() with an undefined parameter on a Wallet instance with an "EMPTY" #state.
Response:
string
wallet.randomAddresses
List of the wallet non-HD (random) addresses.
Response:
Array<{
hash: string
isCiphered: boolean
isHD: boolean
label: string
privateKey: string
}>
wallet.state
Wallet current state.
Response:
enum {
EMPTY = 'EMPTY',
READY = 'READY'
}
wallet.transactions
List of the wallet transactions.
Response:
Array<{
amount: number
date: number // Unix timestamp in seconds
fromAddressHash: string
hash: string
toAddressHash: string
}>
Methods:
wallet.export([unsafe])
Export wallet data with ciphered private keys, or unciphered if is set to TRUE.
See EIP-0001.
Parameters:
<unsafe> boolean Export the wallet with its private keys deciphered if TRUE. Optional. Default to FALSE.
Response:
[
VERSION_INTEGER,
CHAINS_COUNT_INTEGER,
HIERARCHICAL_DETERMINISTIC_MASTER_NODE_PRIVATE_KEY_STRING,
RANDOM_ADDRESSES_PRIVATE_KEYS_STRING_ARRAY
]
wallet.generate([mnemonic], [mnemonicExtension], [chainsCount])
Generate an HD wallet from either the provided mnemonic seed, or a randomly generated one, including ‒ at least ‒ the first derived chain address.
In case the [mnemonicExtension] is specified, it MUST be encoded in UTF-8 using NFKD.
The method can only be called when the wallet #state is 'EMPTY' and will set its #state to 'READY' if successful.
Parameters:
[mnemonic] string The 12 words mnemomic. Optional.
A new one will be generated and accessible via #mnemonic getter if not provided.
[mnemonicExtension] string The mnemonic extension. Optional.
[chainsCount] number Number of chain addresses already generated. Optional. Default to 1.
Response:
Promise<void>
wallet.getBalance([addressHash])
Get the global wallet balance, or the
balance if specified.
Parameters:
[addressHash] string A wallet chain or random address hash. Optional.
Response:
Promise<number>
wallet.lock(<passphrase>, [forStakingOnly])
Lock the wallet, that is cipher all its private keys.
Parameters:
<passphrase> string Wallet encryption passphrase.
[forStakingOnly] boolean Optional. Default to TRUE.
Response:
Promise<void>
wallet.reset()
Reset the current wallet properties and switch the #state to "EMPTY".
Parameters:
N/A
Response:
void
wallet.send(<amount>, <toAddressHash>)
Create and broadcast a new transaction of .
Parameters:
<amount> number Amount, in ECA.
<toAddressHash> string Recipient address hash.
Response:
Promise<void>
wallet.unlock(<passphrase>)
Unlock the wallet, that is decipher all its private keys.
Parameters:
<passphrase> string Wallet encryption passphrase.
Response:
Promise<void>
webServices.getCurrentPriceIn([currency])
Get the current price of ECA via CoinMarketCap.
Parameters:
[currency] string One of: 'AUD', 'BRL', 'CAD', 'CHF', 'CLP', 'CNY', 'CZK', 'DKK', 'EUR', 'GBP',
'HKD', 'HUF', 'IDR', 'ILS', 'INR', 'JPY', 'KRW', 'MXN', 'MYR', 'NOK',
'NZD', 'PHP', 'PKR', 'PLN', 'RUB', 'SEK', 'SGD', 'THB', 'TRY', 'TWD',
'USD', 'ZAR'
Optional. Default to 'USD'.
Response:
number
git clone https://github.com/Electra-project/Electra-JS.git
cd Electra-JS
npm i
Once you're all set up, you can start coding.
npm start
will automatically start a "live" watch :
build
folder),├ build Development release
├ dist Production release (the one distributed via npm)
│ ├ index.d.ts - Types declarations for clients written in Typescript
│ └ index.js - Main bundle
├ node_modules Dependencies local installation directory
├ src The main directory
├ tasks Specific tasks run via the npm scripts
├ test Production release main bundle checkings (import/require tests)
│ ├ browser - Browser compatibility tests (tested via Selenium WebDriver)
│ │ ├ index.html - HTML container served by Express
│ │ ├ index.spec.js - Tests suite run within the browser
│ │ └ index.ts - CLI browser tests runner (checking for browser errors)
│ ├ index.js - Javascript checkings
│ └ index.ts - Typescript checkings
├ .editorconfig Common IDE and Editors configuration
├ .gitignore Files and directories ignored by Git
├ .npmignore Files and directories ignored in the npm published package
├ .npmrc The npm workspace options
├ .travis.yml Travis CI automated tests configuration
├ LICENSE License
├ package-lock.json Accurately versionned list of the npm dependencies tree
├ package.json The npm configuration
├ README.md The current file
├ tsconfig.json Typescript configuration (tsc options)
├ tslint.json TSLint configuration
├ webpack.common.js Common Webpack configuration
├ webpack.dev.js Webpack development configuration
└ webpack.prod.js Webpack production configuration
1/3 Prepare the release
npm version [minor|patch]
It will automatically :
dist/index.js
& dist/index.d.ts
.package.json
(npm job).dist/index.js
.X.Y.Z
matching the new version (npm job).2/3 Push the release
git push origin HEAD
You then need to wait for Travis CI tests to pass.
3/3 Publish the release
npm publish
FAQs
Javascript API middleware allowing clients to interact with Electra blockchain.
The npm package electra-js receives a total of 6 weekly downloads. As such, electra-js popularity was classified as not popular.
We found that electra-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.